Exercise 1: Assume that the data file has hours, payRate, stateTax, and fedTax on one line for each employee. stateTax and fedTax are given as decimals (5% would be .05). Complete this program by filing in the code (places in bold) Exercise 2: Run the program. Note: the data file does not exist so you shoulé get the error message: Error opening file It may not exist where indicated Exercise 3: Create a data file with the following information: 40 15.00 .05 12 50 10 05 .11 60 12.50.05 13
This
and net pay. The code is basically completed just help me fix the bugs.
Exercise 5: Change the program so that the output goes to an output file calledpay.out and run the program. You can use any logical internal name you wish for the output file.
#include <fstream>
#include <iostream>
#include <iomanip>
using namespace std;
int main()
{
// Fill in the code to define payfile as an input file
ifstream payfile;
float gross;
float net;
float hours;
float payRate;
float stateTax;
float fedTax;
cout << fixed << setprecision(2) << showpoint;
// Fill in the code to open payfile and attach it to the physical file
// named payroll.dat
payfile.open("payroll.dat");
// Fill in code to write a conditional statement to check if payfile
// does not exist.
if(!payfile)
{
cout << "Error opening file. \n";
cout << "It may not exist where indicated" << endl;
return 1;
}
ofstream outfile("pay.out");
cout << "Payrate Hours Gross Pay Net Pay"
<< endl << endl;
outfile << "Payrate Hours Gross Pay Net Pay"
<< endl << endl;
// Fill in code to prime the read for the payfile file.
payfile >> hours;
// Fill in code to write a loop condition to run while payfile has more
// data to process.
while(!payfile.eof())
{
payfile >> payRate >> stateTax >> fedTax;
gross = payRate * hours;
net = gross - (gross * stateTax) - (gross * fedTax);
cout << payRate << setw(15) << hours << setw(12) << gross
<< setw(12) << net << endl;
outfile << payRate << setw(15) << hours << setw(12) << gross
<< setw(12) << net << endl;
payfile >> hours ;// Fill in the code to finish this with the appropriate
// variable to be input
}
payfile.close();
outfile.close();
return 0;
}


Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 3 images

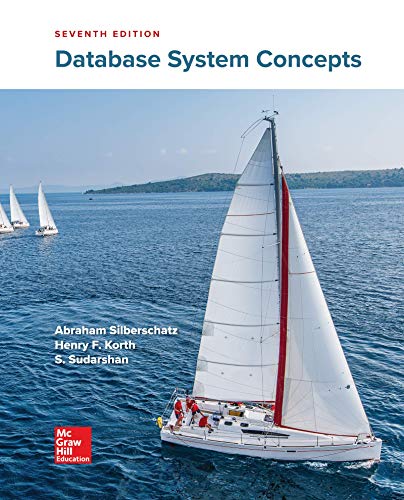
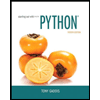
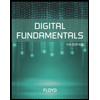
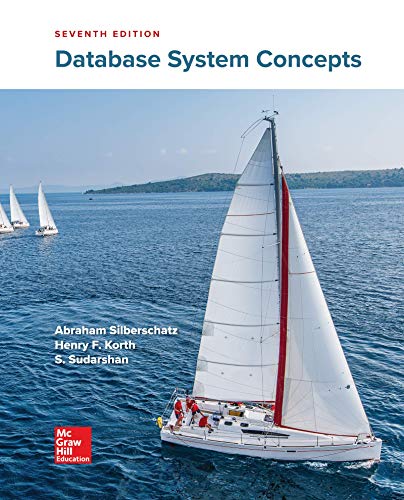
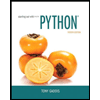
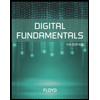
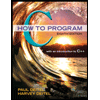
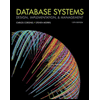
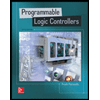