OCaml Code: Attached are images of the instructions. Below is the skeleton code that must be used to produce the example output. Make sure to use the skeleton code to add all the functions needed that is mentioned in the instructions attached. Make sure to attach screenshots of the code and output. I need to make sure the code works. streams.ml type 'a str = Cons of 'a * ('a stream) | Nil and 'a stream = unit -> 'a str exception Subscript exception Empty let head (s :'a stream) : 'a = match s () with Cons (hd,tl) -> hd | Nil -> raise Empty let tail (s :'a stream) : 'a stream = match s () with Cons (hd,tl) -> tl | Nil -> raise Empty let null (s : 'a stream) = match s () with Nil -> true | _ -> false let rec take (n: int) (s: 'a stream) : 'a list = match n with n when n > 0 -> head s :: take (n - 1) (tail s) | 0 -> [] | _ -> raise Subscript let rec nth (n: int) (s: 'a stream) : 'a = match n with n when n > 0 -> nth (n - 1) (tail s) | 0 -> head s | _ -> raise Subscript let rec map (f: 'a -> 'b) (s:'a stream) : 'b stream = fun () -> Cons (f (head s), map f (tail s)) let rec filter (s: 'a stream) (f: 'a -> bool) : 'a stream = if f (head s) then fun () -> Cons (head s, filter (tail s) f) else filter (tail s) f let rec sieve (s: int stream) : int stream = fun () -> Cons(head s, sieve (filter (tail s) (fun x -> x mod (head s) <> 0))) let rec fromn (n: int) = fun () -> Cons (n, fromn (n + 1)) let rec fib n m = fun () -> Cons (n, fib m (n+m)) (* implement the streams and functions below *) let even : int -> bool = fun x -> true let odd : int -> bool = fun x -> true let squares : int stream = fun () -> Nil let fibs : int stream = fun () -> Nil let evenFibs : int stream = fun () -> Nil let oddFibs : int stream = fun () -> Nil let primes : int stream = fun () -> Nil let rev_zip_diff : 'a stream -> 'b stream -> ('b * 'a -> 'c) -> ('b * 'a * 'c) stream = fun a b f -> fun () -> Nil let rec printGenList : 'a list -> ('a -> unit) -> unit = fun l f -> () let rec printList : int list -> string -> unit = fun l f -> let oc = open_out f in close_out oc let rec printPairList : (int * int) list -> string -> unit = fun l f -> let oc = open_out f in close_out oc Example Output: even 2;; - : bool = true even 3;; - : bool = false odd 2;; - : bool = false odd 3;; - : bool = true take 10 squares;; - : int list = [1; 4; 9; 16; 25; 36; 49; 64; 81; 100] take 10 fibs;; - : int list = [0; 1; 1; 2; 3; 5; 8; 13; 21; 34] take 10 evenFibs;; - : int list = [0; 2; 8; 34; 144; 610; 2584; 10946; 46368; 196418] take 10 oddFibs;; - : int list = [1; 1; 3; 5; 13; 21; 55; 89; 233; 377] take 10 primes;; - : int list = [2; 3; 5; 7; 11; 13; 17; 19; 23; 29] take 5 (rev_zip_diff evenFibs oddFibs (fun (x,y) -> x - y));; - : (int * int * int) list = [(1, 0, 1); (1, 2, -1); (3, 8, -5); (5, 34, -29); (13, 144, -131)] printGenList ["how"; "the"; "turntables"] (fun s -> print_string (s ^ " "));; how the turntables - : unit = () printList [2; 4; 6; 8] "printList.txt";; (* "2 4 6 8 " *) printPairList [(2, 1); (3, 2); (4, 3)] "printPairList.txt";; (* "(2, 1) (3, 2) (4, 3) " *)
OCaml Code: Attached are images of the instructions. Below is the skeleton code that must be used to produce the example output. Make sure to use the skeleton code to add all the functions needed that is mentioned in the instructions attached. Make sure to attach screenshots of the code and output. I need to make sure the code works.
streams.ml
type 'a str = Cons of 'a * ('a stream) | Nil
and 'a stream = unit -> 'a str
exception Subscript
exception Empty
let head (s :'a stream) : 'a =
match s () with
Cons (hd,tl) -> hd
| Nil -> raise Empty
let tail (s :'a stream) : 'a stream =
match s () with
Cons (hd,tl) -> tl
| Nil -> raise Empty
let null (s : 'a stream) =
match s () with
Nil -> true
| _ -> false
let rec take (n: int) (s: 'a stream) : 'a list =
match n with
n when n > 0 -> head s :: take (n - 1) (tail s)
| 0 -> []
| _ -> raise Subscript
let rec nth (n: int) (s: 'a stream) : 'a =
match n with
n when n > 0 -> nth (n - 1) (tail s)
| 0 -> head s
| _ -> raise Subscript
let rec map (f: 'a -> 'b) (s:'a stream) : 'b stream =
fun () -> Cons (f (head s), map f (tail s))
let rec filter (s: 'a stream) (f: 'a -> bool) : 'a stream =
if f (head s)
then fun () -> Cons (head s, filter (tail s) f)
else filter (tail s) f
let rec sieve (s: int stream) : int stream =
fun () -> Cons(head s, sieve (filter (tail s) (fun x -> x mod (head s) <> 0)))
let rec fromn (n: int) = fun () -> Cons (n, fromn (n + 1))
let rec fib n m = fun () -> Cons (n, fib m (n+m))
(* implement the streams and functions below *)
let even : int -> bool = fun x -> true
let odd : int -> bool = fun x -> true
let squares : int stream = fun () -> Nil
let fibs : int stream = fun () -> Nil
let evenFibs : int stream = fun () -> Nil
let oddFibs : int stream = fun () -> Nil
let primes : int stream = fun () -> Nil
let rev_zip_diff : 'a stream -> 'b stream -> ('b * 'a -> 'c) -> ('b * 'a * 'c) stream =
fun a b f -> fun () -> Nil
let rec printGenList : 'a list -> ('a -> unit) -> unit =
fun l f -> ()
let rec printList : int list -> string -> unit =
fun l f ->
let oc = open_out f in
close_out oc
let rec printPairList : (int * int) list -> string -> unit =
fun l f ->
let oc = open_out f in
close_out oc
Example Output:
even 2;;
- : bool = true
even 3;;
- : bool = false
odd 2;;
- : bool = false
odd 3;;
- : bool = true
take 10 squares;;
- : int list = [1; 4; 9; 16; 25; 36; 49; 64; 81; 100]
take 10 fibs;;
- : int list = [0; 1; 1; 2; 3; 5; 8; 13; 21; 34]
take 10 evenFibs;;
- : int list = [0; 2; 8; 34; 144; 610; 2584; 10946; 46368; 196418]
take 10 oddFibs;;
- : int list = [1; 1; 3; 5; 13; 21; 55; 89; 233; 377]
take 10 primes;;
- : int list = [2; 3; 5; 7; 11; 13; 17; 19; 23; 29]
take 5 (rev_zip_diff evenFibs oddFibs (fun (x,y) -> x - y));;
- : (int * int * int) list =
[(1, 0, 1); (1, 2, -1); (3, 8, -5); (5, 34, -29); (13, 144, -131)]
printGenList ["how"; "the"; "turntables"] (fun s -> print_string (s ^ " "));;
how the turntables - : unit = ()
printList [2; 4; 6; 8] "printList.txt";; (* "2 4 6 8 " *)
printPairList [(2, 1); (3, 2); (4, 3)] "printPairList.txt";; (* "(2, 1) (3, 2) (4, 3) " *)



Trending now
This is a popular solution!
Step by step
Solved in 3 steps

What the hell is this? You just copied the skeleton code given. Please read the instructions and make sure to produce the example output. Make sure to give the correct code and include screenshots of the example output. I included the instructions again.
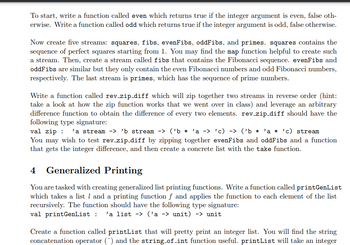
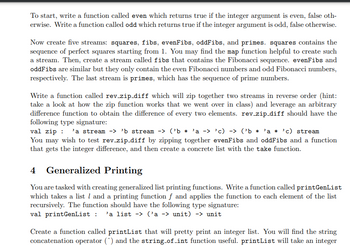
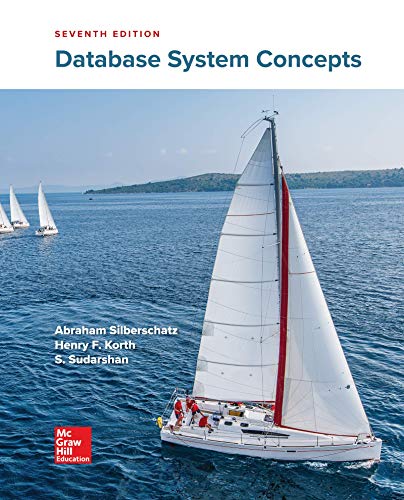
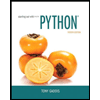
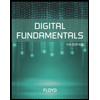
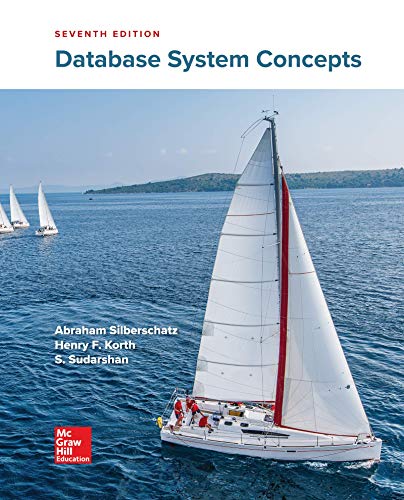
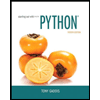
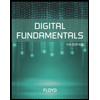
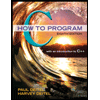
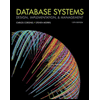
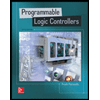