OCaml Code: Below is the code and attached are images of the error and the expected output the code must print out. Make sure to fix the error and print out the expected output. interpreter.ml type 'a str = Cons of 'a * ('a stream) | Nil and 'a stream = unit -> 'a str exception Subscript exception Empty let head (s :'a stream) : 'a = match s () with | Cons (hd,tl) -> hd | Nil -> raise Empty let tail (s :'a stream) : 'a stream = match s () with | Cons (hd,tl) -> tl | Nil -> raise Empty let null (s : 'a stream) = match s () with | Nil -> true | _ -> false
OCaml Code: Below is the code and attached are images of the error and the expected output the code must print out. Make sure to fix the error and print out the expected output.
interpreter.ml
type 'a str = Cons of 'a * ('a stream) | Nil
and 'a stream = unit -> 'a str
exception Subscript
exception Empty
let head (s :'a stream) : 'a =
match s () with
| Cons (hd,tl) -> hd
| Nil -> raise Empty
let tail (s :'a stream) : 'a stream =
match s () with
| Cons (hd,tl) -> tl
| Nil -> raise Empty
let null (s : 'a stream) =
match s () with
| Nil -> true
| _ -> false
let rec take (n: int) (s: 'a stream) : 'a list =
match n with
| n when n > 0 -> head s :: take (n - 1) (tail s)
| 0 -> []
| _ -> raise Subscript
let rec nth (n: int) (s: 'a stream) : 'a =
match n with
| n when n > 0 -> nth (n - 1) (tail s)
| 0 -> head s
| _ -> raise Subscript
let rec map (f: 'a -> 'b) (s:'a stream) : 'b stream =
fun () -> Cons (f (head s), map f (tail s))
let rec filter (s: 'a stream) (f: 'a -> bool) : 'a stream =
if f (head s)
then fun () -> Cons (head s, filter (tail s) f)
else filter (tail s) f
let rec sieve (s: int stream) : int stream =
fun () -> Cons(head s, sieve (filter (tail s) (fun x -> x mod (head s) <> 0)))
let rec fromn (n: int) = fun () -> Cons (n, fromn (n + 1))
let rec fib n m = fun () -> Cons (n, fib m (n+m))
let even : int -> bool = fun x -> x mod 2 = 0
let odd : int -> bool = fun x -> x mod 2 <> 0
let squares : int stream = map (fun x -> x * x) (fromn 1)
let fibs : int stream =
let rec fib_helper a b =
fun () -> Cons(a, fib_helper b (a + b))
in
fib_helper 0 1
let evenFibs : int stream = filter fibs even
let oddFibs : int stream = filter fibs odd
let is_prime (n: int) : bool =
let rec is_not_divisible_by (d: int) : bool =
d * d > n || (n mod d <> 0 && is_not_divisible_by (d + 1))
in
n > 1 && is_not_divisible_by 2
let primes : int stream =
let rec prime_helper n =
if is_prime n then fun () -> Cons(n, prime_helper (n + 1))
else prime_helper (n + 1)
in
prime_helper 2
let rev_zip_diff : 'a stream -> 'b stream -> ('b * 'a -> 'c) -> ('b * 'a * 'c) stream =
fun a b f ->
let rec zip_diff_helper sa sb =
match (sa (), sb ()) with
| (Cons (ha, ta), Cons (hb, tb)) ->
fun () -> Cons ((hb, ha, f (hb, ha)), zip_diff_helper ta tb)
| _ -> fun () -> Nil
in
zip_diff_helper (a ()) (b ())
let rec printGenList : 'a list -> ('a -> unit) -> unit =
fun l f ->
match l with
| [] -> ()
| hd :: tl ->
f hd;
printGenList tl f
let rec printList : int list -> string -> unit =
fun l filename ->
let oc = open_out filename in
let rec print_element = function
| [] -> ()
| [x] -> output_string oc (string_of_int x)
| x :: xs ->
output_string oc (string_of_int x ^ " ");
print_element xs
in
print_element l;
close_out oc
let rec printPairList : (int * int) list -> string -> unit =
fun l filename ->
let oc = open_out filename in
let rec print_pair = function
| [] -> ()
| [(x, y)] -> output_string oc ("(" ^ string_of_int x ^ ", " ^ string_of_int y ^ ")")
| (x, y) :: xs ->
output_string oc ("(" ^ string_of_int x ^ ", " ^ string_of_int y ^ "), ");
print_pair xs
in
print_pair l;
close_out oc
![5 Example output for the entire homework
Note that printList and printPairList must print to the output file, we show the contents of the file
as string for example only.
even 2;;
- bool = true
even 3;;
- bool= false
odd 2;;
- bool = false
odd 3;;
- bool = true
take 10 squares;;
- int list = [1; 4; 9; 16; 25; 36; 49; 64; 81; 100]
take 10 fibs;;
-: int list = [0; 1; 1; 2; 3; 5; 8; 13; 21; 34]
take 10 evenFibs;;
- int list = [0; 2; 8; 34; 144; 610; 2584; 10946; 46368; 196418]
take 10 oddFibs;;
-: int list = [1; 1; 3; 5; 13; 21; 55; 89; 233; 377]
take 10 primes ; ;
- : int list = [2; 3; 5; 7; 11; 13; 17; 19; 23; 29]
take 5 (rev_zip_diff evenFibs oddFibs (fun (x,y) -> x - y)) ;;
- (int int * int) list =
[(1, 0, 1); (1, 2, -1); (3, 8, -5); (5, 34, -29); (13, 144, -131)]
printGenList["how"; "the"; "turntables"] (fun s-> print_string (s^ " ")) ;;
how the turntables : unit = ()
printList [2; 4; 6; 8] "printList.txt";; (* "2 4 6 8 " *)
printPairList [(2, 1); (3, 2); (4, 3)] "printPairList.txt";; (* "(2, 1) (3, 2) (4, 3) " *)
4](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fe7ddc10c-4670-40fd-b02c-6a60c5fcc2f2%2F09aa0946-f6b2-49d4-ad89-dbcc6e95a2ec%2Fx3uslr_processed.png&w=3840&q=75)


Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 4 images

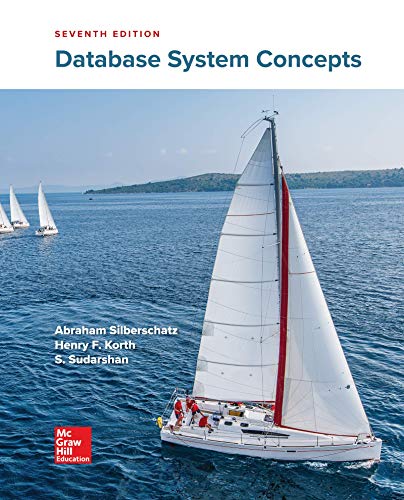
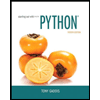
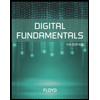
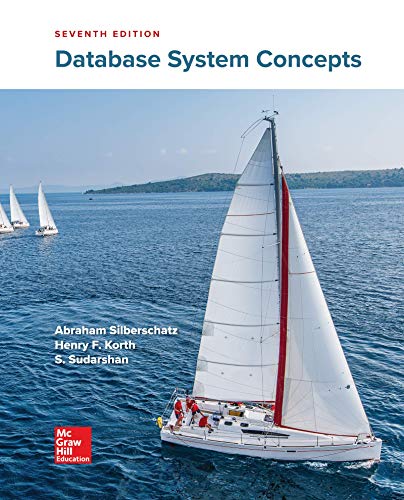
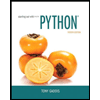
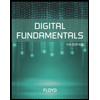
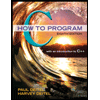
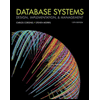
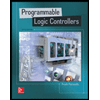