ement four arithmetic operator overload functions (+,-,*,/). c) Implement six relational operator overload functions (==,!=,>,>=,<,<=). d) Implement the insertion operator overload function (<<). e) Implement the extraction operator overload function (>>). f) Implement the subscript operator overload function ([]). Make sure that each fu
C++ (Please answer the ques with showing the code)
Question :
Operator Overloading
a) Implement two unary operator overload functions (-,+,!).
b) Implement four arithmetic operator overload functions (+,-,*,/).
c) Implement six relational operator overload functions (==,!=,>,>=,<,<=).
d) Implement the insertion operator overload function (<<).
e) Implement the extraction operator overload function (>>).
f) Implement the subscript operator overload function ([]).
Make sure that each function is optimally overloaded for its purpose. (pick between member, non-member, friend as appropriate)
Notes Add
Rational Numbers
Given a/b + c/d:
Step 1: Find the LCM of b and d.
Step 2: Create a new Rational Number: ((a*(LCM/b) + (c*(LCM/d)) / LCM.
Step 3: Reduce the new Rational Number from step 2.
Step 4: Return the new Rational Number.
Subtract Rational Numbers
Given a/b - c/d:
Step 1: Find the LCM of b and d.
Step 2: Create a new Rational Number: ((a*(LCM/b) - (c*(LCM/d)) / LCM.
Step 3: Reduce the new Rational Number from step 2.
Step 4: Return the new Rational Number.
Multiply Rational Numbers
Given a/b * c/d:
Step 1: Create a new Rational Number: (a*c) / (b*d).
Step 2: Return the new Rational Number.
Divide Rational Numbers
Given a/b * c/d:
Step 1: Create a new Rational Number: (a*c) / (b*d).
Step 2: Return the new Rational Number.
Compare Rational Numbers:
greater than Determine if a/b > c/d:
Step 1: Find the LCM of b and d.
Step 2: If (a*(LCM/b) > (c*(LCM/d) return true, otherwise false.
Compare Rational Numbers:
less than Determine if a/b < c/d:
Step 1: Find the LCM of b and d.
Step 2: If (a*(LCM/b) < (c*(LCM/d) return true, otherwise false.
Use following main function to test your program. (have to use this main function)
( From here please check the picture that i attached output is in the image.)
(its urgent. please check the code from your side that it works.)
![Use following main function to test your program. (have to use this main function)
int main() {
cout << endl;
RatNum r1(1,2), r2(1,6), r3(2,5);
// test operator overloads
cout << "\nInput/Output Stream Operators: " << endl;
}
RatNum r4;
cout << "Enter a rational number: ";
cin >> r4;
cout << r4 << endl;
cout << "Negation Operation: " << endl;
cout <<-r4 << endl;
// test arithmetic overloads
cout << "\nArithmetic Operators: " << endl;
RatNum r5 r1 + r2;
cout << r1 <<"+" << r2 << "=" <<r5 << endl;
RatNum r6 r1 r2;
cout << r1 <<"-" << r2 << "=" << r6 << endl;
RatNum r7 r1 * r2;
cout << r1 <<"* " << r2 <<
RatNum r8 r1/r2;
Due date: Mar 3, 11:59 PM
"=" <<r7 << endl;
cout << r1 <<"/" << r2 << "=" << r8 << endl;
// test arithmetic operation chaining
cout << "\nArithmetic Chaining: " << endl;
RatNum r9 = r5 + r6 r7 * r8;
cout <<r5 << "+" << r6 <<"-" << r7 <<" * " << r8 << " = " << r9 << endl;
// test relational operator overload
cout << "\nRelational Operators: " << endl;
cout << r5 << " == " << r6 << "? " << (r5==r6) << endl;
cout <<r5 << " != " << r6 << "? " << (r5!=r6) << endl;
cout <<r5 <<">" << r6 <<"? " << (r5>r6) << endl;
cout <<r5 <<"<" << r6 <<"? " << (r5<r6) << endl;
// test subscript overload
cout << "\nSubscript Operator: " << endl;
cout << r5 <<" num=" << r5[1] << " den=" << r5[2] << endl;
cout << endl;
return 0;](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fefd607aa-6776-4e28-838c-67fa22c2e02e%2F7e33800f-e4ad-4d66-ada2-80da63378ecf%2F1eolev_processed.png&w=3840&q=75)


We need to implement the RatNum class as per the given data.
Step by step
Solved in 3 steps with 1 images

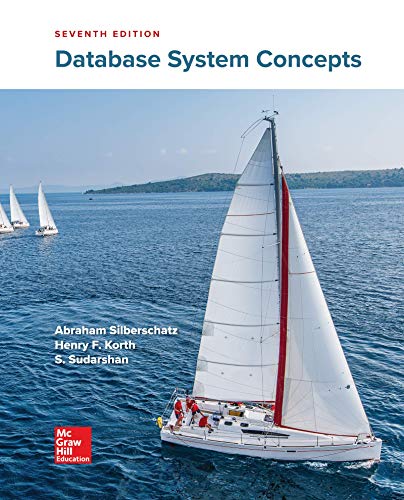
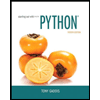
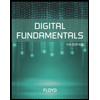
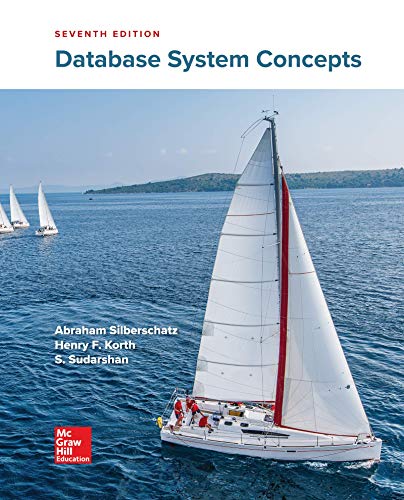
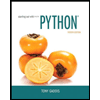
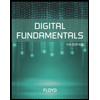
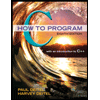
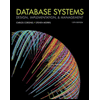
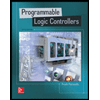