Develop a high-quality, menu-driven object-oriented C++ program that creates a small database, using a binary search tree structure to store and process the data. Your program must include: a Film class that stores all the data for a Film and provides appropriate methods to support good software engineering principles, a FilmDatabase class that stores the binary search tree and provides appropriate methods in support of database queries and reporting using good software engineering principles, an application that interacts with the end-user. The design is up to you and may include any number of classes in support of the given application. A Menu class is strongly recommended. Note that the binary search tree must store Film objects, and the >, <, and == operators must be defined for that class because the BinarySearchTree class uses those overloaded operators. Data Details: The database contains data pertaining to the 100 highest grossing films of 2017. A comma delimited file named Films2017.csv contains the initial data. Each record is stored on one line of the file in the following format: Data Data type Rank int Film Title (key) string Studio string Total Gross double Total Theaters int Opening Gross double Opening Theaters int Opening Date string Each of the data fields is separated in the file using the comma (,) character as a delimiter. There is no comma (,) character after the last field on the line. The data is considered clean; There are no errors in the input file. When storing the data in the binary search tree, use the data types shown above. The Film Title will serve as the key field. Therefore, an inorder traversal of the BST will produce the Films in order of title. The menu system consists of a main menu and sub-menus. All menu choices are selected by entering the letter of the desired choice. After a selection is processed, the current menu should be re-displayed. Do NOT use recursion to do this; use a loop. The current menu continues until the X option (return to main menu or exit) is selected. When the application begins, the following main menu should be displayed: MAIN MENU A - About the Application R - Reports S - Search the Database X - Exit the Program Enter Selection ->
Please help me with this code in C++, I dont know to write a menu for this. Please help me create a Film.h, Film.cpp, Main, and A Menu. thank you!!
Develop a high-quality, menu-driven object-oriented C++ program that creates a small
Your program must include:
- a Film class that stores all the data for a Film and provides appropriate methods to support good software engineering principles,
- a FilmDatabase class that stores the binary search tree and provides appropriate methods in support of database queries and reporting using good software engineering principles,
- an application that interacts with the end-user. The design is up to you and may include any number of classes in support of the given application. A Menu class is strongly recommended.
Note that the binary search tree must store Film objects, and the >, <, and == operators must be defined for that class because the BinarySearchTree class uses those overloaded operators.
Data Details:
The database contains data pertaining to the 100 highest grossing films of 2017. A comma delimited file named Films2017.csv contains the initial data. Each record is stored on one line of the file in the following format:
Data | Data type |
Rank | int |
Film Title (key) | string |
Studio | string |
Total Gross | double |
Total Theaters | int |
Opening Gross | double |
Opening Theaters | int |
Opening Date | string |
Each of the data fields is separated in the file using the comma (,) character as a delimiter. There is no comma (,) character after the last field on the line. The data is considered clean; There are no errors in the input file.
When storing the data in the binary search tree, use the data types shown above. The Film Title will serve as the key field. Therefore, an inorder traversal of the BST will produce the Films in order of title.
The menu system consists of a main menu and sub-menus. All menu choices are selected by entering the letter of the desired choice. After a selection is processed, the current menu should be re-displayed. Do NOT use recursion to do this; use a loop. The current menu continues until the X option (return to main menu or exit) is selected.
When the application begins, the following main menu should be displayed:
MAIN MENU
A - About the Application
R - Reports
S - Search the Database
X - Exit the Program
Enter Selection ->
BinaryTreeADT.H:
#ifndef BINARYTREEADT_H
#define BINARYTREEADT_H
template <class T>
class BinaryTreeADT {
public:
virtual bool isEmpty() const = 0;
virtual bool add(const T& newItem) = 0;
virtual bool remove(const T& delItem) = 0;
virtual void clear() = 0;
virtual bool contains(const T& findItem) const = 0;
virtual void inorderTraverse(void visit(T&)) const = 0;
private:
};
#endif /* BINARYTREEADT_H */
BinarySearchTree.H:
#ifndef BINARYSEARCHTREE_H
#define BINARYSEARCHTREE_H
#include "BinaryTreeADT.h"
#include "BinaryNode.h"
template <class T>
class BinarySearchTree : BinaryTreeADT<T> {
public:
BinarySearchTree();
BinarySearchTree(const BinarySearchTree& orig);
virtual ~BinarySearchTree();
//interface methods
bool isEmpty() const;
bool add(const T& newItem);
bool remove(const T& delItem);
void clear();
bool contains(const T& findItem) const;
void inorderTraverse(void visit(T& item)) const;
private:
BinaryNode<T>* root;
//recursive methods
void destroyTree(BinaryNode<T>* currRoot);
BinaryNode<T>* copyTree(const BinaryNode<T>* currRoot) const;
BinaryNode<T>* placeNode(BinaryNode<T>* currRoot, BinaryNode<T>* newNode);
bool findNode(BinaryNode<T>* currRoot, const T& item) const;
void inorder(BinaryNode<T>* currRoot, void visit(T& item)) const;
};
#include "BinarySearchTree.cpp"
#endif /* BINARYSEARCHTREE_H */
BinaryNode.H:
#ifndef BINARYNODE_H
#define BINARYNODE_H
template <class T>
class BinaryNode {
public:
BinaryNode();
BinaryNode(const T& newItem,
BinaryNode<T>* left = nullptr,
BinaryNode<T>* right = nullptr);
BinaryNode(const BinaryNode& orig);
virtual ~BinaryNode();
T getItem() const;
void setItem(const T& newItem);
BinaryNode<T>* getLeftChild() const;
BinaryNode<T>* getRightChild() const;
void setLeftChild(BinaryNode<T>* left);
void setRightChild(BinaryNode<T>* right);
bool isLeaf() const;
private:
T item;
BinaryNode<T>* leftChild;
BinaryNode<T>* rightChild;
};
#include "BinaryNode.cpp"
#endif /* BINARYNODE_H */



Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

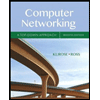
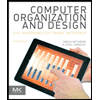
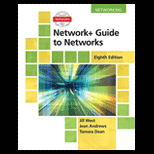
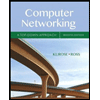
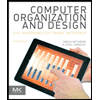
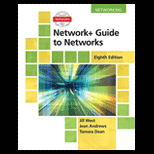
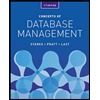
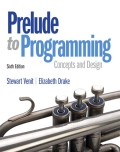
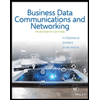