Data structure & Algorithum java program 1) Add a constructor to the class "AList" that creates a list from a given array of objects. 2) Add a method "getPosition" to the "AList" class that returns the position of a given object in the list. The header of the method is as follows : public int getPosition(T anObject) 3) Write a program that thoroughly tests all the methods in the class "AList". 4) Include comments to all source code.
Data structure & Algorithum java program 1) Add a constructor to the class "AList" that creates a list from a given array of objects. 2) Add a method "getPosition" to the "AList" class that returns the position of a given object in the list. The header of the method is as follows : public int getPosition(T anObject) 3) Write a program that thoroughly tests all the methods in the class "AList". 4) Include comments to all source code.
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
Data structure & Algorithum java
1) Add a constructor to the class "AList" that creates a list from a given array of objects.
2) Add a method "getPosition" to the "AList" class that returns the position of a given object in the list. The header of the method is as follows : public int getPosition(T anObject)
3) Write a program that thoroughly tests all the methods in the class "AList".
4) Include comments to all source code.
![Here's a transcription of the image:
```java
import chapter5.ListInterface.*;
public class AList<T> implements ListInterface<T> {
private T[] list;
private int numberOfEntries;
private boolean integrityOK = false;
private static final int DEFAULT_CAPACITY = 25;
private static final int MAX_CAPACITY = 1000;
public AList() throws Exception {
this(DEFAULT_CAPACITY);
}
public AList(int initialCapacity) throws Exception {
integrityOK = false;
// is capacity too small?
if (initialCapacity < DEFAULT_CAPACITY)
initialCapacity = DEFAULT_CAPACITY;
else
checkCapacity(initialCapacity);
T[] tempList = (T[]) new Object[initialCapacity + 1];
list = tempList;
numberOfEntries = 0;
integrityOK = true;
}
private void checkCapacity(int capacity) throws Exception {
if (capacity > MAX_CAPACITY)
throw new Exception("Attempt to create a list whose capacity exceeds the max.");
}
@Override
public void add(T newEntry) {
add(numberOfEntries + 1, newEntry);
}
@Override
public void add(int newPosition, T newEntry) {
try {
checkIntegrity();
if ((newPosition >= 1) && (newPosition <= numberOfEntries + 1)) {
if (newPosition <= numberOfEntries + 1) {
makeRoom(newPosition);
list[newPosition] = newEntry;
numberOfEntries++;
ensureCapacity();
}
}
} catch (Exception e) {
System.out.println(e.getMessage());
}
}
private void checkIntegrity() {
// Implementation not shown
}
private void makeRoom(int newPosition) {
// Implementation not shown
}
private void ensureCapacity() {
// Implementation not shown
}
}
```
### Overview
This code defines a Java class called `AList` that implements the `ListInterface` interface. The class is designed to manage a list that can dynamically grow as new elements are added. It includes key attributes and methods:
1. **Attributes**:
- `list`: An array to store the entries.
- `numberOfEntries`: An integer tracking the number of entries in the list.
- `integrityOK`: A boolean indicating whether](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fdad5a646-4583-4c4b-beba-29e57d936914%2F5368ca6d-ccb5-40bd-bb12-92101512392a%2Fpb8czqc_processed.jpeg&w=3840&q=75)
Transcribed Image Text:Here's a transcription of the image:
```java
import chapter5.ListInterface.*;
public class AList<T> implements ListInterface<T> {
private T[] list;
private int numberOfEntries;
private boolean integrityOK = false;
private static final int DEFAULT_CAPACITY = 25;
private static final int MAX_CAPACITY = 1000;
public AList() throws Exception {
this(DEFAULT_CAPACITY);
}
public AList(int initialCapacity) throws Exception {
integrityOK = false;
// is capacity too small?
if (initialCapacity < DEFAULT_CAPACITY)
initialCapacity = DEFAULT_CAPACITY;
else
checkCapacity(initialCapacity);
T[] tempList = (T[]) new Object[initialCapacity + 1];
list = tempList;
numberOfEntries = 0;
integrityOK = true;
}
private void checkCapacity(int capacity) throws Exception {
if (capacity > MAX_CAPACITY)
throw new Exception("Attempt to create a list whose capacity exceeds the max.");
}
@Override
public void add(T newEntry) {
add(numberOfEntries + 1, newEntry);
}
@Override
public void add(int newPosition, T newEntry) {
try {
checkIntegrity();
if ((newPosition >= 1) && (newPosition <= numberOfEntries + 1)) {
if (newPosition <= numberOfEntries + 1) {
makeRoom(newPosition);
list[newPosition] = newEntry;
numberOfEntries++;
ensureCapacity();
}
}
} catch (Exception e) {
System.out.println(e.getMessage());
}
}
private void checkIntegrity() {
// Implementation not shown
}
private void makeRoom(int newPosition) {
// Implementation not shown
}
private void ensureCapacity() {
// Implementation not shown
}
}
```
### Overview
This code defines a Java class called `AList` that implements the `ListInterface` interface. The class is designed to manage a list that can dynamically grow as new elements are added. It includes key attributes and methods:
1. **Attributes**:
- `list`: An array to store the entries.
- `numberOfEntries`: An integer tracking the number of entries in the list.
- `integrityOK`: A boolean indicating whether
![The image shows a section of Java code, likely part of a class handling an array-based list structure. Below is a transcription and explanation of the code:
```java
private void ensureCapacity() throws Exception {
int capacity = list.length - 1;
if (numberOfEntries >= capacity) {
int newCapacity = 2 * capacity;
checkCapacity(newCapacity);
list = Arrays.copyOf(list, newCapacity + 1);
}
}
private void makeRoom(int newPosition) {
int newIndex = newPosition;
int lastIndex = numberOfEntries;
for (int index = lastIndex; index >= newIndex; index--) {
list[index + 1] = list[index];
}
}
private void checkIntegrity() throws Exception {
if (!integrityOK)
throw new Exception("AList object is corrupt.");
}
@Override
public T remove(int givenPosition) {
// TODO Auto-generated method stub
return null;
}
@Override
public void clear() {
// TODO Auto-generated method stub
}
@Override
public T replace(int givenPosition, T newEntry) {
// TODO Auto-generated method stub
return null;
}
@Override
public T getEntry(int givenPosition) {
// TODO Auto-generated method stub
return null;
}
@Override
public T[] toArray() {
try {
checkIntegrity();
T[] result = (T[]) new Object[numberOfEntries + 1];
for (int index = 0; index < numberOfEntries; index++)
result[index] = list[index + 1];
return result;
} catch (Exception e) {
e.printStackTrace();
return null;
}
}
```
### Explanation:
1. **ensureCapacity()**: Ensures the array backing the list has sufficient capacity. If not, it doubles the capacity and copies the existing elements into a new array with the increased size.
2. **makeRoom()**: Shifts elements to make space for a new entry at the specified position. It starts from the end (lastIndex) and shifts each element one position to the right up to newIndex.
3. **checkIntegrity()**: Checks if the list is in a valid state before performing operations; throws an exception if the list is corrupt.
4. **remove, clear, replace, getEntry**: These method signatures are present, but their implementations are](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fdad5a646-4583-4c4b-beba-29e57d936914%2F5368ca6d-ccb5-40bd-bb12-92101512392a%2F47l508l_processed.jpeg&w=3840&q=75)
Transcribed Image Text:The image shows a section of Java code, likely part of a class handling an array-based list structure. Below is a transcription and explanation of the code:
```java
private void ensureCapacity() throws Exception {
int capacity = list.length - 1;
if (numberOfEntries >= capacity) {
int newCapacity = 2 * capacity;
checkCapacity(newCapacity);
list = Arrays.copyOf(list, newCapacity + 1);
}
}
private void makeRoom(int newPosition) {
int newIndex = newPosition;
int lastIndex = numberOfEntries;
for (int index = lastIndex; index >= newIndex; index--) {
list[index + 1] = list[index];
}
}
private void checkIntegrity() throws Exception {
if (!integrityOK)
throw new Exception("AList object is corrupt.");
}
@Override
public T remove(int givenPosition) {
// TODO Auto-generated method stub
return null;
}
@Override
public void clear() {
// TODO Auto-generated method stub
}
@Override
public T replace(int givenPosition, T newEntry) {
// TODO Auto-generated method stub
return null;
}
@Override
public T getEntry(int givenPosition) {
// TODO Auto-generated method stub
return null;
}
@Override
public T[] toArray() {
try {
checkIntegrity();
T[] result = (T[]) new Object[numberOfEntries + 1];
for (int index = 0; index < numberOfEntries; index++)
result[index] = list[index + 1];
return result;
} catch (Exception e) {
e.printStackTrace();
return null;
}
}
```
### Explanation:
1. **ensureCapacity()**: Ensures the array backing the list has sufficient capacity. If not, it doubles the capacity and copies the existing elements into a new array with the increased size.
2. **makeRoom()**: Shifts elements to make space for a new entry at the specified position. It starts from the end (lastIndex) and shifts each element one position to the right up to newIndex.
3. **checkIntegrity()**: Checks if the list is in a valid state before performing operations; throws an exception if the list is corrupt.
4. **remove, clear, replace, getEntry**: These method signatures are present, but their implementations are
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 4 images

Recommended textbooks for you
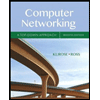
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
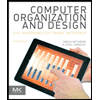
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
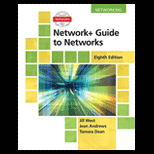
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
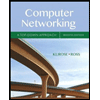
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
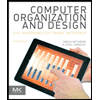
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
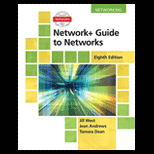
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
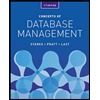
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
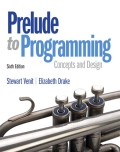
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
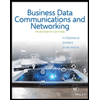
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY