Create your own vector class which will test algorithms from the STL Derive class myVector from vector. myVector must implement the following methods: int seqSearch(T searchItem); int binarySearch(T searchItem); void bubbleSort(); void insertionSort(); Create a test program to create some vectors and test your methods above. Recall from your reading that binary search only works on a sorted list. Add a static member to the class to “remember” if the list is sorted ( i.e. binarySearch() should first sort the vector if it’s not sorted already). Use the template below as a starter for your assignment. All comments in bold represent code which you need to implement. #include #include #include using namespace std; template class myVector: public vector { public: int seqSearch(T searchItem); int binarySearch(T searchItem); void bubbleSort(); void insertionSort(); }; template int myVector::seqSearch(T searchItem) { //implement sequential search } template void myVector::bubbleSort() { //implement bubble sort } template void myVector::insertionSort() { //implement insertion sort } template int myVector::binarySearch(T searchItem) { //implement binary search } int main() { //define test vector(s) myVector nameList; //add values to the vector(s) //test sort methods //test search methods //print sorted vector using range based for loop //define new test vector(s) //define an iterator to each of the above vector containers //add values to the vector(s) //test the STL sort method //test the STL binary_search algorithm //print the resulting vector(s) using an iterator return 0; } Useful notes: this->size(); //length of vector from within myVector class this->at(index); //value at specified index of vector from within myVector class
Create your own vector class which will test algorithms from the STL
Derive class myVector from vector. myVector must implement the following methods:
int seqSearch(T searchItem);
int binarySearch(T searchItem);
void bubbleSort();
void insertionSort();
Create a test program to create some vectors and test your methods above. Recall from your reading that binary search only works on a sorted list. Add a static member to the class to “remember” if the list is sorted ( i.e. binarySearch() should first sort the vector if it’s not sorted already).
Use the template below as a starter for your assignment. All comments in bold represent code which you need to implement.
#include <iostream>
#include <string>
#include <vector>
using namespace std;
template <class T>
class myVector: public vector<T> {
public:
int seqSearch(T searchItem);
int binarySearch(T searchItem);
void bubbleSort();
void insertionSort();
};
template <class T>
int myVector<T>::seqSearch(T searchItem)
{
//implement sequential search
}
template <class T>
void myVector<T>::bubbleSort()
{
//implement bubble sort
}
template <class T>
void myVector<T>::insertionSort()
{
//implement insertion sort
}
template <class T>
int myVector<T>::binarySearch(T searchItem)
{
//implement binary search
}
int main()
{
//define test vector(s)
myVector<string> nameList;
//add values to the vector(s)
//test sort methods
//test search methods
//print sorted vector using range based for loop
//define new test vector(s)
//define an iterator to each of the above vector containers
//add values to the vector(s)
//test the STL sort method
//test the STL binary_search
//print the resulting vector(s) using an iterator
return 0;
}
Useful notes:
this->size(); //length of vector from within myVector class
this->at(index); //value at specified index of vector from within myVector class

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 8 images

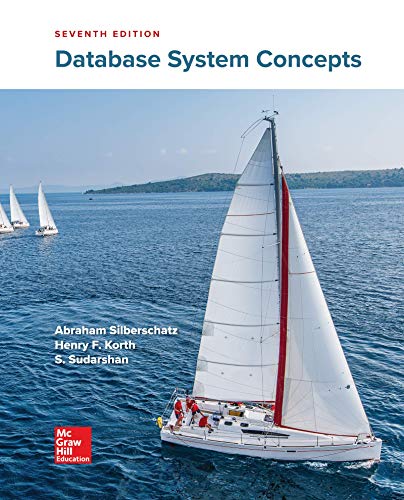
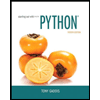
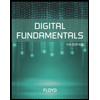
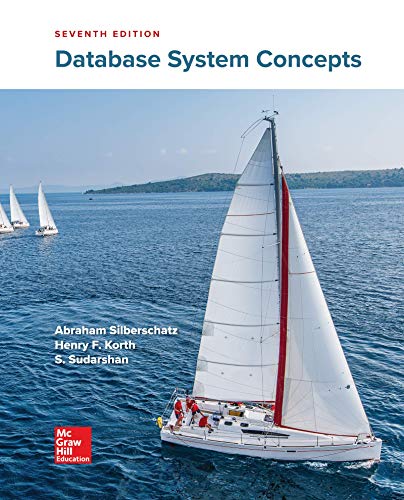
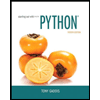
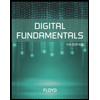
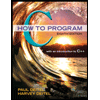
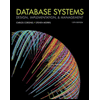
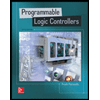