create a Word document that contains the pseudocode, flowchart, and test plan for both projects (Programming Project 4(import java.util.Scanner; public class Exercise06_25 { public static void main(String[] args) { Scanner input = new Scanner(System.in); System.out.print("Enter time in milliseconds: "); long milliseconds = input.nextLong(); System.out.println(convertMillis(milliseconds)); } public static String convertMillis(long millis) { // Calculate the number of hours, minutes, and seconds long hours = millis / 3600000; millis %= 3600000; long minutes = millis / 60000; millis %= 60000; long seconds = millis / 1000; // Return the formatted string return hours + ":" + minutes + ":" + seconds; } }) and Programming Project 5(import java.util.Scanner; public class Exercise06_37 { public static void main(String[] args) { Scanner input = new Scanner(System.in); System.out.print("Enter an integer: "); int number = input.nextInt(); System.out.print("Enter the width: "); int width = input.nextInt(); System.out.println("The formatted number is " + format(number, width)); } public static String format(int number, int width) { String numberString = String.valueOf(number); // Convert the number to a string // Check if the width is smaller than the length of the number if (width < numberString.length()) { return numberString; } // Add prefix 0s to the number string until it reaches the specified width while (numberString.length() < width) { numberString = "0" + numberString; }
create a Word document that contains the pseudocode, flowchart, and test plan for both projects (
public class Exercise06_25 {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
System.out.print("Enter time in milliseconds: ");
long milliseconds = input.nextLong();
System.out.println(convertMillis(milliseconds));
}
public static String convertMillis(long millis) {
// Calculate the number of hours, minutes, and seconds
long hours = millis / 3600000;
millis %= 3600000;
long minutes = millis / 60000;
millis %= 60000;
long seconds = millis / 1000;
// Return the formatted string
return hours + ":" + minutes + ":" + seconds;
}
}) and Programming Project 5(import java.util.Scanner;
public class Exercise06_37 {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
System.out.print("Enter an integer: ");
int number = input.nextInt();
System.out.print("Enter the width: ");
int width = input.nextInt();
System.out.println("The formatted number is " + format(number, width));
}
public static String format(int number, int width) {
String numberString = String.valueOf(number); // Convert the number to a string
// Check if the width is smaller than the length of the number
if (width < numberString.length()) {
return numberString;
}
// Add prefix 0s to the number string until it reaches the specified width
while (numberString.length() < width) {
numberString = "0" + numberString;
}
return numberString;
}
}
)) that you completed in Revel.

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

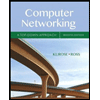
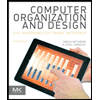
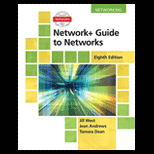
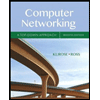
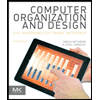
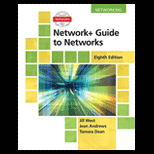
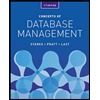
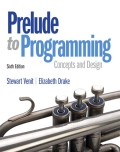
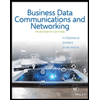