Create a program to allow one of the cards (e.g. card 0) to be twice as rare as the other cards. Using a rare card will make the number of boxes needed to buy much larger. Use Program 1 as a reference to create. Use Code from Program 1 Below:
Computer Science
** Java Program **
Create a program to allow one of the cards (e.g. card 0) to be twice as rare as the other cards. Using a rare card will make the number of boxes needed to buy much larger. Use Program 1 as a reference to create.
Use Code from Program 1 Below:
public static void main(String[] args) {
/**
* A cereal producer puts in each box of cereals a collector card
* with player from your favorite sport.
* We would like to know which is the average number of boxes that we need to buy
* in order to get all the cards.
* To compute the number we will run simulations that will randomly pick a card
* for each bought box, until we get all the cards.
* The user will provide the number of players in the promotion
* and how many simulations we will run.
* We want to compute and print some statistics related to the performed simulations:
* - average number of boxes bought
* - minimum number of boxes bought
* - maximum number of boxes bought.
*
*
*/
public class CardCollectorSimulation {
/**
* @param args
*/
public static void main(String[] args) {
System.out.println("Card Collector Simulation");
// Read the number of players in the promotion
Scanner scanner=new Scanner(System.in);
int numberOfPlayers;
boolean error;
do {
System.out.print("Number of players: ");
numberOfPlayers = scanner.nextInt();
error = numberOfPlayers<2;
if (error) {
System.out.println("Please provide a whole number greater than 1.");
}
} while(error);
// Read the number of simulations
int numberOfSimulations;
do {
System.out.println("Number of simulations:");
numberOfSimulations = scanner.nextInt();
error = numberOfSimulations < 1;
if (error) {
System.out.println("Please provide a whole number at least 1.");
}
} while (error);
// Define the array for the results of simulations
int[] numberOfBoxes = new int[numberOfSimulations];
// for each simulation, run the simulation and store the results
for (int s=1; s<=numberOfSimulations; s++) {
// run the simulation s
// initialize the marker that keeps what cards I already bought
boolean[] bought = new boolean[numberOfPlayers];
// no card bought
for (int c=0; c<numberOfPlayers; c++) bought[c]=false;
int numberOfDifferentCards = 0;
int boxNumber=0;
do {
boxNumber++;
int cardNumber = (int)(Math.random()*numberOfPlayers);
if (!bought[cardNumber]) {
numberOfDifferentCards++;
bought[cardNumber] = true;
}
} while(numberOfDifferentCards<numberOfPlayers);
System.out.printf("Simulation %5d needs %4d boxes.\n", s, boxNumber);
numberOfBoxes[s-1]=boxNumber;
}
// process the results to compute average, minimum, maximum
int sum = numberOfBoxes[0];
int minimum = numberOfBoxes[0];
int maximum = numberOfBoxes[0];
for (int s=1; s<numberOfSimulations; s++) {
sum += numberOfBoxes[s];
if (numberOfBoxes[s] < minimum) minimum = numberOfBoxes[s];
else if (numberOfBoxes[s] > maximum) maximum = numberOfBoxes[s];
}
double average = 1.0 * sum / numberOfSimulations;
// print the results
System.out.println("Average number of boxes: "+average);
System.out.println("Minimum number of boxes: "+minimum);
System.out.println("Maximum number of boxes: "+maximum);
}
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

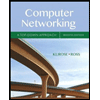
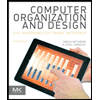
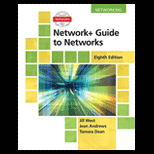
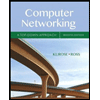
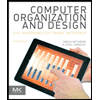
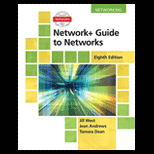
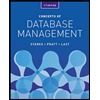
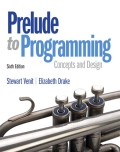
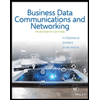