Create a method multiply(), that receives the 2D array you created in the program below as as an input, multiplies all of its elements and displays the product. Hint: your method won’t return anything import java.util.*; public class Two_D_Ragged { public static void Using_array_class(int R_array[][]) {System.out.println(""); System.out.println("using Arrays class Through the Ragged Array"); //Array.toString method is used to show array elements in R_array[0] System.out.println(Arrays.toString(R_array[0])); //Array.toString method is used to show array elements in R_array[1] System.out.println(Arrays.toString(R_array[1])); //Array.toString method is used to show array elements in R_array[2] System.out.println(Arrays.toString(R_array[2])); } //end of Using_array_class method public static void Using_for_each(int R_array[][]) { System.out.println("for-each-loop Through the Ragged Array"); int k = 0; //prints elements in R_array[0] for (int i:R_array[0]) { System.out.print(R_array[0][k] +" "); k++; } System.out.println(""); int l = 0; //prints elements in R_array[1] for (int i:R_array[1]) { System.out.print(R_array[1][l] +" "); l++; } System.out.println(""); int m = 0; //prints elements in R_array[2] for (int i:R_array[2]) { System.out.print(R_array[2][m] +" "); m++; } } //end of Using_for_each method public static void Using_for_loop(int R_array[][]) { System.out.println("for-loop Through the Ragged Array"); for (int i = 0; i < R_array.length; i++) { for (int j = 0; j < R_array[i].length; j++) { System.out.print(R_array[i][j]+ " "); } System.out.println(""); } } public static void main(String[] args) { int[][] R_array = new int [3][]; R_array[0] = new int[]{10, 20, 30}; R_array[1] = new int[]{40, 50}; R_array[2] = new int[]{60}; Using_for_loop(R_array);//calling method to print array elements using for loop Using_for_each(R_array); //calling method to print array elements using for-each loop Using_array_class(R_array); //calling method to print array elements using Array class }//end of main method }//end of Two_D_Ragged class
Create a method multiply(), that receives the 2D array you created in the program below as as an input, multiplies all of its elements and displays the product.
Hint: your method won’t return anything
import java.util.*;
public class Two_D_Ragged {
public static void Using_array_class(int R_array[][]) {
System.out.println("");
System.out.println("using Arrays class Through the Ragged Array");
//Array.toString method is used to show array elements in R_array[0]
System.out.println(Arrays.toString(R_array[0]));
//Array.toString method is used to show array elements in R_array[1]
System.out.println(Arrays.toString(R_array[1]));
//Array.toString method is used to show array elements in R_array[2]
System.out.println(Arrays.toString(R_array[2]));
} //end of Using_array_class method
public static void Using_for_each(int R_array[][]) {
System.out.println("for-each-loop Through the Ragged Array");
int k = 0;
//prints elements in R_array[0]
for (int i:R_array[0]) {
System.out.print(R_array[0][k] +" ");
k++;
}
System.out.println("");
int l = 0;
//prints elements in R_array[1]
for (int i:R_array[1]) {
System.out.print(R_array[1][l] +" ");
l++;
}
System.out.println("");
int m = 0;
//prints elements in R_array[2]
for (int i:R_array[2]) {
System.out.print(R_array[2][m] +" ");
m++;
}
} //end of Using_for_each method
public static void Using_for_loop(int R_array[][]) {
System.out.println("for-loop Through the Ragged Array");
for (int i = 0; i < R_array.length; i++) {
for (int j = 0; j < R_array[i].length; j++) {
System.out.print(R_array[i][j]+ " ");
}
System.out.println("");
}
}
public static void main(String[] args) {
int[][] R_array = new int [3][];
R_array[0] = new int[]{10, 20, 30};
R_array[1] = new int[]{40, 50};
R_array[2] = new int[]{60};
Using_for_loop(R_array);//calling method to print array elements using for loop
Using_for_each(R_array); //calling method to print array elements using for-each loop
Using_array_class(R_array); //calling method to print array elements using Array class
}//end of main method
}//end of Two_D_Ragged class

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

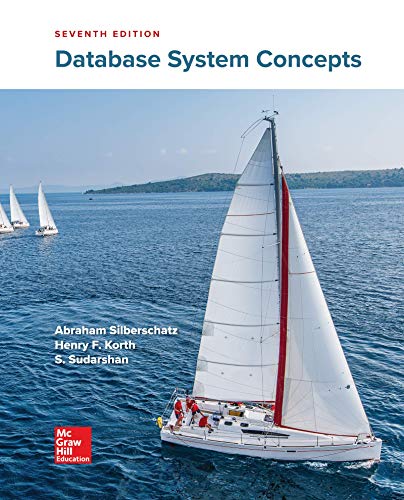
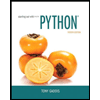
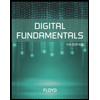
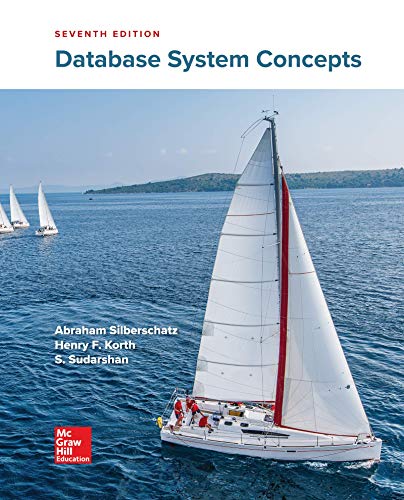
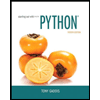
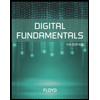
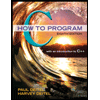
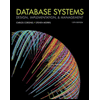
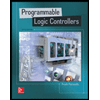