Write a program that calls a method that takes a character array as a parameter (you can initialize an array in the main method) and returns the number of vowels in the array. Partial program is given below.
![1. Write a program that calls a method that takes a character array as a
parameter (you can initialize an array in the main method) and returns the
number of vowels in the array. Partial program is given below.
public class CountVowels{
public static void main(String[] args){
char[] arr=
System.out.print("There are "+vowels(arr)+" vowels in the array");](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F480f989f-2af6-48a5-ab4d-ef9ac310c5f5%2Fce5e4aa1-46e9-42a6-92f9-a2cf315502f0%2Fxrb2jcw_processed.png&w=3840&q=75)

Program code:
//define class CountVowels
public class CountVowels
{
//define a method to count the vowels
static int vowels(char arr[])
{
//declare the variables
int count = 0;
char ch;
//iterate a for loop
for (int i = 0; i < arr.length; i++)
{
//get the first charecter to ch
ch = arr[i];
//check if ch is a vowel
if (ch == 'a' || ch == 'e' || ch == 'i' || ch == 'o' || ch == 'u' ||
ch == 'A' || ch == 'E' || ch == 'I' || ch == 'O' || ch == 'U')
//increment the count by 1
count++;
}
//return the value of count
return count;
}
//define main function
public static void main(String[] args)
{
//charecter array
char[] arr={'H','e','l','l','o'};
//print the number of vowels
System.out.print("There are "+vowels(arr)+" vowels in the array");
}
}
Program code #1:
Step by step
Solved in 3 steps with 2 images

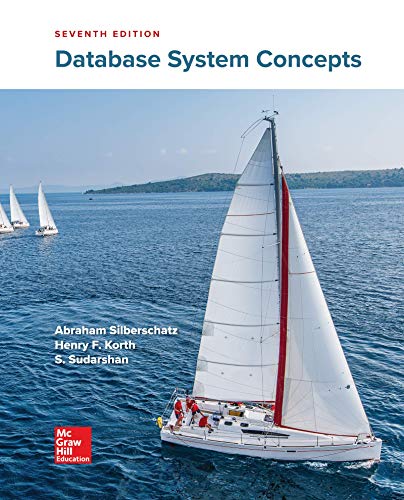
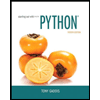
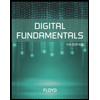
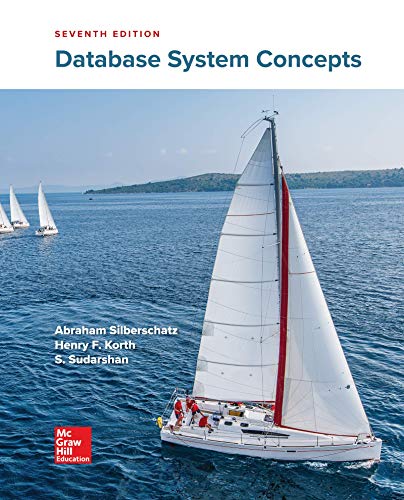
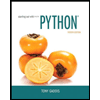
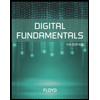
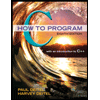
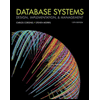
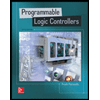