Correct the coding user enter number 2 in single seat make it unavailable import 'dart:io'; void main() { stdout.writeln("Types of seat (Double / Single)?: "); String? types = stdin.readLineSync(); dynamicseats(types); dynamicprice(types); } void dynamicseats(types) { if (types == 'Single') { Map single = { 1: "A", 4: "A", 7: "A", 10: "A", 13: "A", 16: "A", 19: "A", 22: "A", 25: "A", 28: "A" }; print("The available seats: $single"); stdout.writeln("Choose your seat number?: "); var Singleseat = stdin.readLineSync(); var intSingleseat = int.parse(Singleseat!); single.remove(intSingleseat); stdout.write( "\nYour seat type is $types and your seat number is $intSingleseat "); print("\nAvailable seats: $single"); } else if (types == 'Double') { stdout.writeln("Choose (Isle / Window)?: "); String? BC = stdin.readLineSync(); Map isle = { 2: "B", 5: "B", 8: "B", 11: "B", 14: "B", 17: "B", 20: "B", 23: "B", 26: "B", 29: "B" }; Map window = { 3: "C", 6: "C", 9: "C", 12: "C", 15: "C", 18: "C", 21: "C", 24: "C", 27: "C", 30: "C" }; if (BC == 'Isle') { print("The available seats: $isle"); stdout.writeln("Choose your seat number?: "); var IsleSeat = stdin.readLineSync(); var intIsleSeat = int.parse(IsleSeat!); isle.remove(intIsleSeat); stdout.write( "\nYour seat type is $types ($BC) and your seat number is $intIsleSeat "); print("\nAvailable seats: $isle"); } else if (BC == 'Window') { print(window); stdout.writeln("Choose your seat number?: "); var Windowseat = stdin.readLineSync(); var intWindowseat = int.parse(Windowseat!); window.remove(intWindowseat); stdout.write( "\nYour seat type is $types ($BC) and your seat number is $intWindowseat "); print("\nAvailable seats: $window"); } } } void dynamicprice(types) { if (types == 'Single') { String price = "RM30"; stdout.write("The price for Single seat is $price"); } else if (types == 'Double') { String price = "RM45"; stdout.write("The price for Double (Isle/Window) seat is $price"); } }
Correct the coding user enter number 2 in single seat make it unavailable import 'dart:io'; void main() { stdout.writeln("Types of seat (Double / Single)?: "); String? types = stdin.readLineSync(); dynamicseats(types); dynamicprice(types); } void dynamicseats(types) { if (types == 'Single') { Map single = { 1: "A", 4: "A", 7: "A", 10: "A", 13: "A", 16: "A", 19: "A", 22: "A", 25: "A", 28: "A" }; print("The available seats: $single"); stdout.writeln("Choose your seat number?: "); var Singleseat = stdin.readLineSync(); var intSingleseat = int.parse(Singleseat!); single.remove(intSingleseat); stdout.write( "\nYour seat type is $types and your seat number is $intSingleseat "); print("\nAvailable seats: $single"); } else if (types == 'Double') { stdout.writeln("Choose (Isle / Window)?: "); String? BC = stdin.readLineSync(); Map isle = { 2: "B", 5: "B", 8: "B", 11: "B", 14: "B", 17: "B", 20: "B", 23: "B", 26: "B", 29: "B" }; Map window = { 3: "C", 6: "C", 9: "C", 12: "C", 15: "C", 18: "C", 21: "C", 24: "C", 27: "C", 30: "C" }; if (BC == 'Isle') { print("The available seats: $isle"); stdout.writeln("Choose your seat number?: "); var IsleSeat = stdin.readLineSync(); var intIsleSeat = int.parse(IsleSeat!); isle.remove(intIsleSeat); stdout.write( "\nYour seat type is $types ($BC) and your seat number is $intIsleSeat "); print("\nAvailable seats: $isle"); } else if (BC == 'Window') { print(window); stdout.writeln("Choose your seat number?: "); var Windowseat = stdin.readLineSync(); var intWindowseat = int.parse(Windowseat!); window.remove(intWindowseat); stdout.write( "\nYour seat type is $types ($BC) and your seat number is $intWindowseat "); print("\nAvailable seats: $window"); } } } void dynamicprice(types) { if (types == 'Single') { String price = "RM30"; stdout.write("The price for Single seat is $price"); } else if (types == 'Double') { String price = "RM45"; stdout.write("The price for Double (Isle/Window) seat is $price"); } }
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Topic Video
Question
Correct the coding
user enter number 2 in single seat make it unavailable
import 'dart:io';
void main() {
stdout.writeln("Types of seat (Double / Single)?: ");
String? types = stdin.readLineSync();
dynamicseats(types);
dynamicprice(types);
}
void dynamicseats(types) {
if (types == 'Single') {
Map<int, String> single = {
1: "A",
4: "A",
7: "A",
10: "A",
13: "A",
16: "A",
19: "A",
22: "A",
25: "A",
28: "A"
};
print("The available seats: $single");
stdout.writeln("Choose your seat number?: ");
var Singleseat = stdin.readLineSync();
var intSingleseat = int.parse(Singleseat!);
single.remove(intSingleseat);
stdout.write(
"\nYour seat type is $types and your seat number is $intSingleseat ");
print("\nAvailable seats: $single");
} else if (types == 'Double') {
stdout.writeln("Choose (Isle / Window)?: ");
String? BC = stdin.readLineSync();
Map<int, String> isle = {
2: "B",
5: "B",
8: "B",
11: "B",
14: "B",
17: "B",
20: "B",
23: "B",
26: "B",
29: "B"
};
Map<int, String> window = {
3: "C",
6: "C",
9: "C",
12: "C",
15: "C",
18: "C",
21: "C",
24: "C",
27: "C",
30: "C"
};
if (BC == 'Isle') {
print("The available seats: $isle");
stdout.writeln("Choose your seat number?: ");
var IsleSeat = stdin.readLineSync();
var intIsleSeat = int.parse(IsleSeat!);
isle.remove(intIsleSeat);
stdout.write(
"\nYour seat type is $types ($BC) and your seat number is $intIsleSeat ");
print("\nAvailable seats: $isle");
} else if (BC == 'Window') {
print(window);
stdout.writeln("Choose your seat number?: ");
var Windowseat = stdin.readLineSync();
var intWindowseat = int.parse(Windowseat!);
window.remove(intWindowseat);
stdout.write(
"\nYour seat type is $types ($BC) and your seat number is $intWindowseat ");
print("\nAvailable seats: $window");
}
}
}
void dynamicprice(types) {
if (types == 'Single') {
String price = "RM30";
stdout.write("The price for Single seat is $price");
} else if (types == 'Double') {
String price = "RM45";
stdout.write("The price for Double (Isle/Window) seat is $price");
}
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-engineering and related others by exploring similar questions and additional content below.Recommended textbooks for you
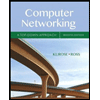
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
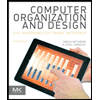
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
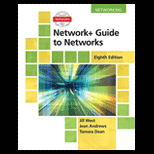
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
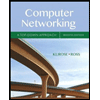
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
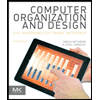
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
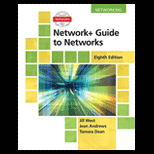
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
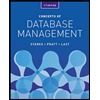
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
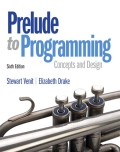
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
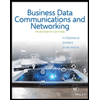
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY