Convert this Java code to C++: public class Problem5 { public static void main(String[] args) { //loop variables int i = 0; int num = 0; int num_count = 0; //Empty String to store prime numbers String primeNumbers = ""; //First for loop which runs from 1 to 10000 for (i = 1; i <= 10000; i++) { int counter = 0; //second for loop which runs from selected number to 1 for (num = i; num >= 1; num--) { //using modulus operator to check if selected number is divisible by any number from num(i) to 1 if (i % num == 0) { counter = counter + 1; //count the divisible } } //if counter is 2 then it is prime number(divisible by themselves and one (1)) if (counter == 2) { //Appended the Prime number to the String primeNumbers = primeNumbers + i + " "; num_count++; //arrange numbers, 50 numbers per line if (num_count == 30) { primeNumbers += "\n"; num_count = 0; } } } //display prime numbers System.out.println("Prime numbers from 1 to 10000: "); System.out.println(primeNumbers); }}
Convert this Java code to C++:
public class Problem5 {
public static void main(String[] args) {
//loop variables
int i = 0;
int num = 0;
int num_count = 0;
//Empty String to store prime numbers
String primeNumbers = "";
//First for loop which runs from 1 to 10000
for (i = 1; i <= 10000; i++) {
int counter = 0;
//second for loop which runs from selected number to 1
for (num = i; num >= 1; num--) {
//using modulus operator to check if selected number is divisible by any number from num(i) to 1
if (i % num == 0) {
counter = counter + 1; //count the divisible
}
}
//if counter is 2 then it is prime number(divisible by themselves and one (1))
if (counter == 2) {
//Appended the Prime number to the String
primeNumbers = primeNumbers + i + " ";
num_count++;
//arrange numbers, 50 numbers per line
if (num_count == 30) {
primeNumbers += "\n";
num_count = 0;
}
}
}
//display prime numbers
System.out.println("Prime numbers from 1 to 10000: ");
System.out.println(primeNumbers);
}
}

Step by step
Solved in 2 steps

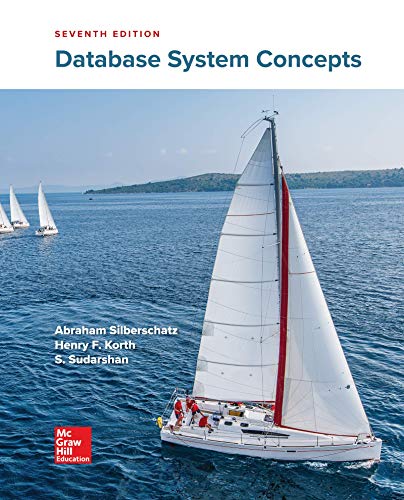
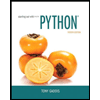
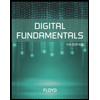
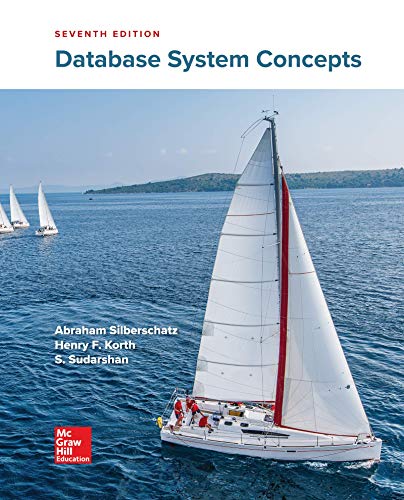
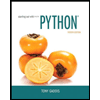
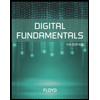
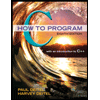
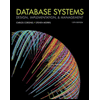
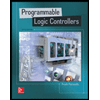