CONVERT THIS CODE TO C#
CONVERT THIS CODE TO C#
Code For ATM Withdraw Sysytem
C++ code:
#include <iostream>
#include <stdlib.h>
#include <string.h>
using namespace std;
class Bank {
// Private variables used inside class
private:
string name;
int accnumber;
char type[10];
int amount = 0;
int tot = 0;
// Public variables
public:
// Function to set the person's data
void setvalue()
{
cout << "Enter name\n";
cin.ignore();
// To use space in string
getline(cin, name);
cout << "Enter Account number\n";
cin >> accnumber;
cout << "Enter Account type\n";
cin >> type;
cout << "Enter Balance\n";
cin >> tot;
}
// Function to display the required data
void showdata()
{
cout << "Name:" << name << endl;
cout << "Account No:" << accnumber << endl;
cout << "Account type:" << type << endl;
cout << "Balance:" << tot << endl;
}
// Function to deposit the amount in ATM
void deposit()
{
cout << "\nEnter amount to be Deposited\n";
cin >> amount;
}
// Function to show the balance amount
void showbal()
{
tot = tot + amount;
cout << "\nTotal balance is: " << tot;
}
// Function to withdraw the amount in ATM
void withdrawl()
{
int a, avai_balance;
cout << "Enter amount to withdraw\n";
cin >> a;
avai_balance = tot - a;
cout << "Available Balance is" << avai_balance;
}
};
// Driver Code
int main()
{
// Object of class
Bank b;
int choice;
// Infinite while loop to choose
// options everytime
while (1) {
cout << "\n~~~~~~~~~~~~~~~~~~~~~~~~~~"
<< "~~~~~~~~~~~~~~~~~~~~~~~~~~~~"
<< "~~~WELCOME~~~~~~~~~~~~~~~~~~"
<< "~~~~~~~~~~~~~~~~~~~~~~~~~~~~"
<< "~~~~~~~~~\n\n";
cout << "Enter Your Choice\n";
cout << "\t1. Enter name, Account "
<< "number, Account type\n";
cout << "\t2. Balance Enquiry\n";
cout << "\t3. Deposit Money\n";
cout << "\t4. Show Total balance\n";
cout << "\t5. Withdraw Money\n";
cout << "\t6. Cancel\n";
cin >> choice;
// Choices to select from
switch (choice) {
case 1:
b.setvalue();
break;
case 2:
b.showdata();
break;
case 3:
b.deposit();
break;
case 4:
b.showbal();
break;
case 5:
b.withdrawl();
break;
case 6:
exit(1);
break;
default:
cout << "\nInvalid choice\n";
}
}
}
Code For ATM Pin changing Sysytem:
#include "atm.h"
#include <iostream>
#include <iomanip>
#include <string>
#include <fstream>
#include <conio.h>
#include <stdlib.h>
using namespace std;
void ATM::verifyPIN()
{
string pass="";
int i;
char pinNumber;
string accountList = "E:/CpE 31 Projects/Toledo/acct.lst";
ifstream accountFileIn;
accountFileIn.open(accountList.data(), ios::in);
if (accountFileIn.is_open())
{
accountFileIn >> ID >> PIN >> balance;
}
cout << "Enter PIN number: ";
pinNumber = getch();
while(pinNumber != 13)
{
pass.push_back(pinNumber);
cout << '*';
pinNumber = getch();
}
system("CLS");
for (i = 2; i > 0; i--)
{
if(pass != PIN)
{
cout << "Incorrect PIN. Try again: ";
pinNumber = getch();
while(pinNumber != 13)
{
pass.push_back(pinNumber);
cout << '*';
pinNumber = getch();
}
system("CLS");
if (i == 1 && pass != PIN)
{
cout << "Number of attempts out. ATM will now close." << endl;
exit(1);
}
else if (i == 1 && pass == PIN)
{
}
}
else
{
}
}
accountFileIn.close();
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

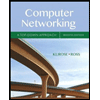
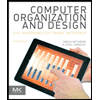
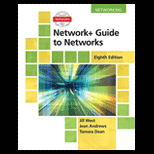
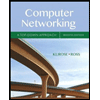
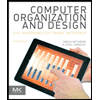
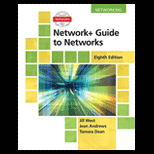
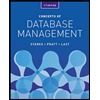
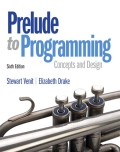
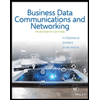