Provide a Java class named SortedPriorityQueue that implements a priority queue using a Java array of type int. The constructor of the class should be passed the size of the queue. Each time the add method is called, the value passed to it should be inserted into the array to ensure that the array remains in sorted order. If the array is full when add is called, a RuntimeException should be thrown. If the array is empty when remove is called, a RuntimeException should also be thrown Make the implementation as efficient as possible.
Provide a Java class named SortedPriorityQueue that implements a priority queue
using a Java array of type int. The constructor of the class should be passed the size of
the queue. Each time the add method is called, the value passed to it should be inserted
into the array to ensure that the array remains in sorted order. If the array is full when add
is called, a RuntimeException should be thrown. If the array is empty when remove is
called, a RuntimeException should also be thrown Make the implementation as efficient
as possible.

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Consider the following sorting
void sort(int[] array) {
SortedPriorityQueue queue = new SortedPriorityQueue(100); for (int i = 0; i < array.length; i++)
queue.add(array[i]);
for (int i = 0; i < array.length; i++)
array[i] = queue.remove();
}
Analyze its execution time efficiency in the worst case. In your analysis you may ignore the possibility that the array may overflow. Indicate whether this implementation is more or less efficient than the one that uses the Java priority queue.
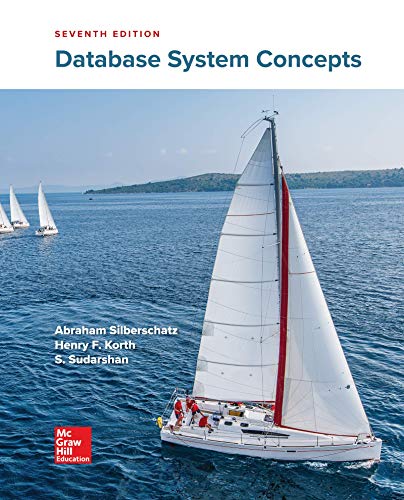
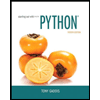
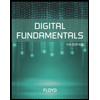
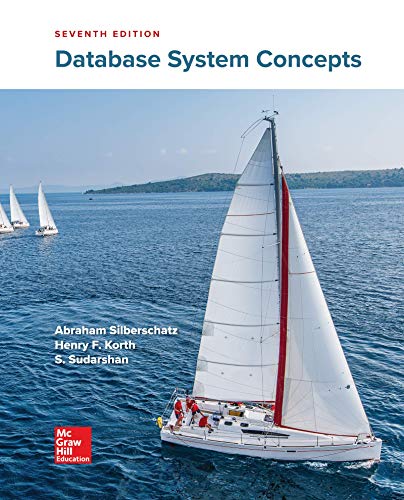
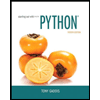
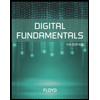
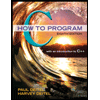
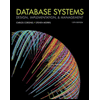
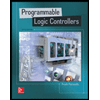