Consider the code and output below. Is Car more likely to be a struct or a class? func test(prototype: Car) -> String { prototype.speed = 100 // Assume that speed is an Int variable prototype.saveDiagnostics() // Assume saveDiagnostics stores information about changes to the car state prototype.speed = 120 prototype.saveDiagnostics() prototype.speed = 200 prototype.saveDiagnostics() return prototype.GetDiagnostics() // Assume GetDiagnostics returns a diagnostics report } var myCar = Car(speed: 0) print("Speed before testing: \(myCar.speed)") var result = test(prototype: myCar) print("Speed after testing: \(myCar.speed)") Screen output: Speed before testing: 0 Speed after testing: 0 1. Car is a class 2. Car is a struct 3. Car is neither a class nor a struct
Consider the code and output below. Is Car more likely to be a struct or a class?
func test(prototype: Car) -> String {
prototype.speed = 100 // Assume that speed is an Int variable
prototype.saveDiagnostics() // Assume saveDiagnostics stores information about changes to the car state
prototype.speed = 120
prototype.saveDiagnostics()
prototype.speed = 200
prototype.saveDiagnostics()
return prototype.GetDiagnostics() // Assume GetDiagnostics returns a diagnostics report
}
var myCar = Car(speed: 0)
print("Speed before testing: \(myCar.speed)")
var result = test(prototype: myCar)
print("Speed after testing: \(myCar.speed)")
Screen output:
Speed before testing: 0
Speed after testing: 0

- The question is to know if the code provided is using struct or a class.
Step by step
Solved in 2 steps

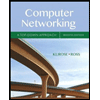
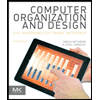
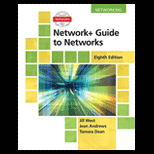
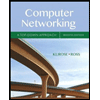
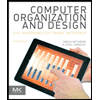
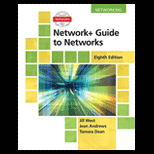
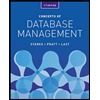
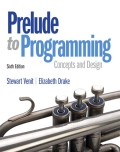
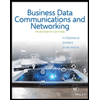