Code in Java Define a class named MobilePhone with the attributes brand name, model, color and price. Define 3 constructors - the first one is the default constructor, the second one initializes all data members and the third one gives an initial value to the brand name Apple, model iPhone XR and price to 54250 while allowing color to be initialized by the user. Also, write a display method that prints in this manner: Brand: Samsung Model: S10 Color: Black Price: 53300.0 Write a method updatePrice that updates the price of the mobile phone. The method accepts a price. If the value of the price is negative the price must be deducted with the value. But if positive price must be added with the value. It will not allow update of price if will result to a negative value for price. For example: Test Result MobilePhone phone = new MobilePhone("Samsung", "S10", "Black", 53300.00); phone.display(); Brand: Samsung Model: S10 Color: Black Price: 53300.0 MobilePhone phone = new MobilePhone(); phone.updatePrice(1200); phone.display(); Brand: null Model: null Color: null Price: 1200.0
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Code in Java
Define a class named MobilePhone with the attributes brand name, model, color and price. Define 3 constructors - the first one is the default constructor, the second one initializes all data members and the third one gives an initial value to the brand name Apple, model iPhone XR and price to 54250 while allowing color to be initialized by the user. Also, write a display method that prints in this manner:
Brand: Samsung
Model: S10
Color: Black
Price: 53300.0
Write a method updatePrice that updates the price of the mobile phone. The method accepts a price. If the value of the price is negative the price must be deducted with the value. But if positive price must be added with the value. It will not allow update of price if will result to a negative value for price.
For example:
Test | Result |
---|---|
MobilePhone phone = new MobilePhone("Samsung", "S10", "Black", 53300.00); phone.display(); |
Brand: Samsung Model: S10 Color: Black Price: 53300.0 |
MobilePhone phone = new MobilePhone(); phone.updatePrice(1200); phone.display(); |
Brand: null Model: null Color: null Price: 1200.0 |


Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 3 images

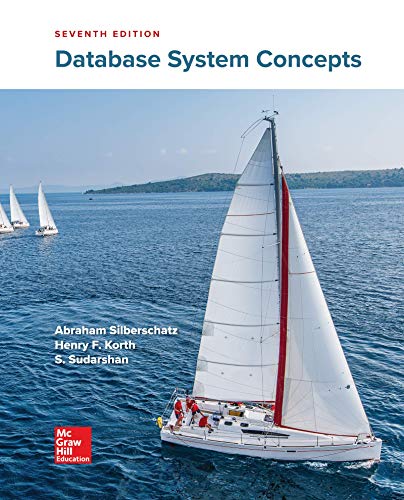
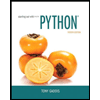
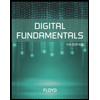
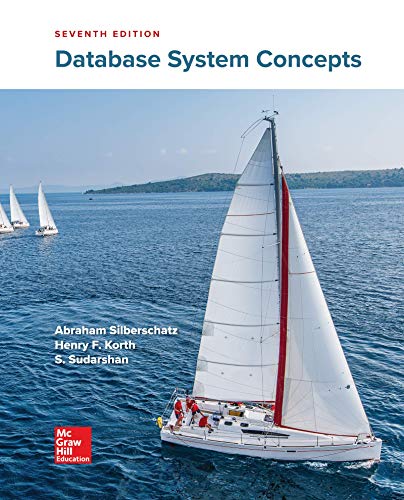
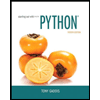
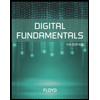
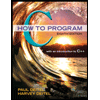
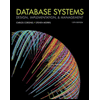
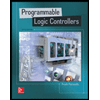