Code below is of tic tac toe game in C. With 2d array. But it is between player 1 and player 2. I want modification in my code so that the game is between player 1 and computer. The computer move will be through rand() function. Please help me out.
Code below is of tic tac toe game in C. With 2d array. But it is between player 1 and player 2. I want modification in my code so that the game is between player 1 and computer. The computer move will be through rand() function. Please help me out.
Code:
#include<stdio.h>
#include <stdbool.h>
char matrix[3][3] = {{'1', '2', '3'},
{'4', '5', '6'},
{'7', '8', '9'}};
int choice, row, column;
char mark = 'O';
bool isDraw = false;
void showmatrix() {
int t;
for (t = 0; t < 3; t++) {
printf(" %c | %c | %c ", matrix[t][0],
matrix[t][1], matrix[t][2]);
if (t != 2) printf("\n---|---|---\n");
}
printf("\n");
printf("\n");
}
void user_turn() {
if (mark == 'O') {
printf("User turn : ");
} else if (mark == 'X') {
printf("Computer turn : ");
}
scanf("%d", &choice);
switch (choice) {
case 1:
row = 0;
column = 0;
break;
case 2:
row = 0;
column = 1;
break;
case 3:
row = 0;
column = 2;
break;
case 4:
row = 1;
column = 0;
break;
case 5:
row = 1;
column = 1;
break;
case 6:
row = 1;
column = 2;
break;
case 7:
row = 2;
column = 0;
break;
case 8:
row = 2;
column = 1;
break;
case 9:
row = 2;
column = 2;
break;
default:
printf("Invalid Move");
}
if (mark == 'X' && matrix[row][column] != 'X' && matrix[row][column] != 'O') {
matrix[row][column] = 'X';
mark = 'O';
} else if (mark == 'O' && matrix[row][column] != 'X' && matrix[row][column] != 'O') {
matrix[row][column] = 'O';
mark = 'X';
} else {
user_turn();
}
showmatrix();
}
bool gameover() {
for (int i = 0; i < 3; i++) { /*checking rows and columns*/
if (matrix[i][0] == matrix[i][1] && matrix[i][0] == matrix[i][2] ||
matrix[0][i] == matrix[1][i] && matrix[0][i] == matrix[2][i])
return false;
}
for (int i = 0; i < 3; i++) { /*checking if game is drawn*/
for (int j = 0; j < 3; j++) {
if (matrix[i][j] != 'X' && matrix[i][j] != 'O')
return true;
}
}
if (matrix[0][0] == matrix[1][1] && matrix[0][0] == matrix[2][2] || /*checking diagonals*/
matrix[0][2] == matrix[1][1] && matrix[0][2] == matrix[2][0]){
return false;
}
isDraw = true;
return false;
}
int main() {
while (gameover()) {
showmatrix();
user_turn();
gameover();
}
if (mark == 'X' && !isDraw) {
printf("User wins the game");
} else if (mark == 'O' && !isDraw) {
printf("Computer wins the game");
} else
printf("The game ended in a draw");
return 0;

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

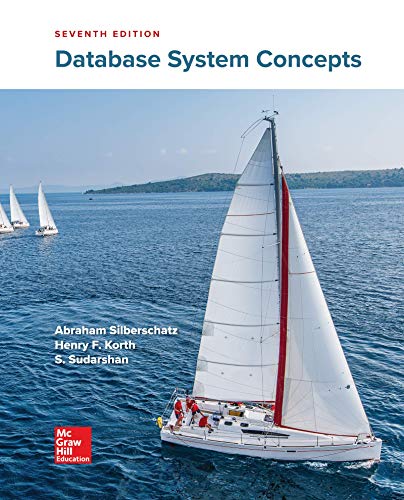
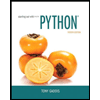
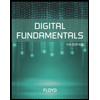
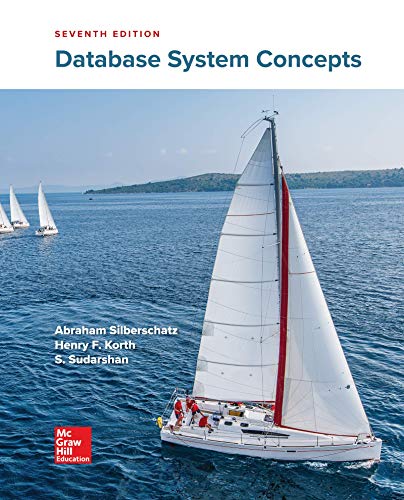
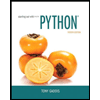
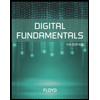
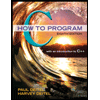
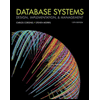
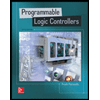