cleAndSphere.java // Step 1: Import the Scanner class for input import java.util.Scanner; /** * A Java program to calculate the circumference, area, * surface area, and volume based on an input radius. 1 3 4 5 Step 2: Enter your name for @author.and today's date for @version * @author () 8. 9. @version () */ public class CircleAndSphere { public static void main(String[] args) 10 11 12 13 14 15 // Step 3: Create a Scanner object to read input from the keyboard Scanner sc = new Scanner(System.in); 16 17 18 // Step 4: Display a prompt "Enter the radius: " // 19 and stay on the same line 20 System.out.print("Enter the radius"); 21 22 // Step 5: Read a double number for radius and 23 // store it in a variable 24 double radius = sc.nextDouble(); 25 26 // Step 6: Calculate the circumference using the input radius 27 // double result = 2*Math.PI * radius; and store it in a variable 28 29 30 31 // Step 7: Calculate the area using the input radius // double area = Math.PI* radius* radius; 32 and store it in a variable 33 34 35 // Step 8: Calculate the surface area using the input radius 36 // and store it in a variable 37
![rcleAndSphere.java
// Step 1: Import the Scanner class for input
import java.util.Scanner;
1
/**
* A Java program to calculate the circumference, area,
* surface area, and volume based on an input radius.
4
Step 2: Enter your name for @author.and today's date for @version
* @author
* @version ()
7
8.
10
*/
public class CircleAndSphere
{
public static void main(String[] args)
11
12
13
14
15
// Step 3: Create a Scanner object to read input from the keyboard
Scanner sc = new Scanner(System.in);
16
17
18
// Step 4: Display a prompt "Enter the radius: "
19
//
and stay on the same line
System.out.print("Enter the radius");
20
21
22
// Step 5: Read a double number for radius and
23
//
store it in a variable
24
double radius = sc.nextDouble();
25
26
// Step 6: Calculate the circumference using the input radius
27
//
double result = 2*Math. PI * radius;
and store it in a variable
28
29
30
31
// Step 7: Calculate the area using the input radius
32
//
and store it in a variable
33
double area = Math.PI* radius* radius;
34
35
// Step 8: Calculate the surface area using the input radius
//
36
and store it in a variable
37](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F170cb9b5-2292-4ecc-9f24-3c60291ff66d%2F81ef913a-ca1d-4459-ba5c-2d24d1a2a644%2Fqobygt9_processed.jpeg&w=3840&q=75)


CORRECTED PROGRAM CODE:
// Step 1: Import the Scanner class for input
import java.util.Scanner;
/*
* A Java program to calculate the circumference, area,
* surface area, and volume based on an input radius.
*
* Step 2: Enter your name for @author. and today's date for @version
* @author ()
* @version ()
*/
public class Main
{
public static void main(String[] args)
{
// Step 3: Create a Scanner object to read input from the keyboard
Scanner sc = new Scanner (System. in);
// Step 4: Display a prompt "Enter the radius: "
// and stay on the same line
System.out.print("Enter the radius");
// Step 5: Read a double number for radius and
// store it in a variable
double radius = sc.nextDouble();
// Step 6: Calculate the circumference using the input radius
// and store it in a variable
double result = 2*Math.PI * radius;
// Step 7: Calculate the area using the input radius
// and store it in a variable
double area = Math.PI* radius* radius;
// Step 8: Calculate the surface area using the input radius
// and store it in a variable
double SurfaceArea = 4* area;
// Step 9: Calculate the volume using the input radius
// and store it in a variable
double volume = 4*Math.PI*Math.pow(radius, 2);
// Step 10: Display the radius and calculated values
// Sample output for radius 5
// The radius: 5.0
// The circle circumference: 31.41592653589793
// The circle area: 78:53981633974483
// The sphere surface area: 314.1592653589793
// The sphere volume: 523.5987755982989
System. out.println("The radius: "+radius);
System.out.println(" The circle circumference: " + result) ;
System.out.println(" The circle area: "+area) ;
System.out.println(" The sphere surface are:" + SurfaceArea) ;
}
}
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

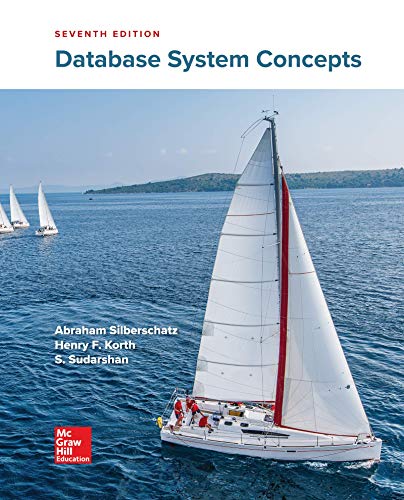
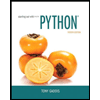
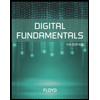
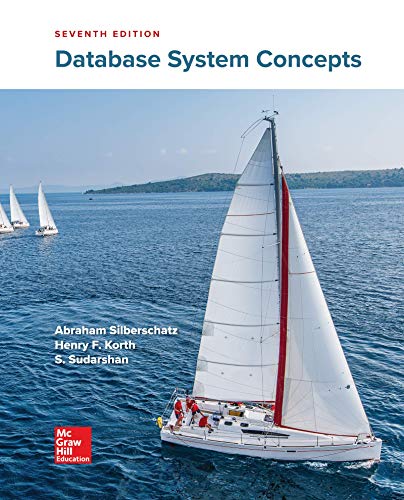
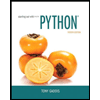
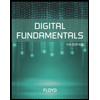
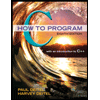
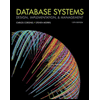
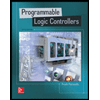