CHALLENGE ACTIVITY 6.5.2: Function definition: Volume of a pyramid with modular functions. Define a function calc_pyramid_volume() with parameters base_length, base_width, and pyramid_height, that returns the volume of a pyramid with a rectangular base. calc_pyramid_volume() calls the given calc_base_area() function in the calculation. Relevant geometry equations: Volume = base area x height x 1/3 (Watch out for integer division). Sample output with inputs: 4.5 2.1 3.0 Volume for 4.5, 2.1, 3.0 is: 9.45 Use Python please.
CHALLENGE ACTIVITY 6.5.2: Function definition: Volume of a pyramid with modular functions. Define a function calc_pyramid_volume() with parameters base_length, base_width, and pyramid_height, that returns the volume of a pyramid with a rectangular base. calc_pyramid_volume() calls the given calc_base_area() function in the calculation. Relevant geometry equations: Volume = base area x height x 1/3 (Watch out for integer division). Sample output with inputs: 4.5 2.1 3.0 Volume for 4.5, 2.1, 3.0 is: 9.45 Use Python please.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Concept explainers
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Question
CHALLENGE ACTIVITY
6.5.2: Function definition: Volume of a pyramid with modular functions.
Define a function calc_pyramid_volume() with parameters base_length, base_width, and pyramid_height, that returns the volume of a pyramid with a rectangular base. calc_pyramid_volume() calls the given calc_base_area() function in the calculation.
Relevant geometry equations:
Volume = base area x height x 1/3
(Watch out for integer division).
Sample output with inputs: 4.5 2.1 3.0
Relevant geometry equations:
Volume = base area x height x 1/3
(Watch out for integer division).
Sample output with inputs: 4.5 2.1 3.0
Volume for 4.5, 2.1, 3.0 is: 9.45
Use Python please.

Transcribed Image Text:This image displays a Python code snippet intended for educational purposes, specifically for calculating the volume of a pyramid. The snippet includes a function and some interactive inputs. Here’s a breakdown of the script:
### Python Code Breakdown
1. **Function Definition**:
- `def calc_base_area(base_length, base_width):`
- This line defines a function named `calc_base_area` that takes two parameters: `base_length` and `base_width`.
- `return base_length * base_width`
- This line calculates and returns the area of the base of the pyramid by multiplying the `base_length` by the `base_width`.
2. **Space for Additional Code**:
- `''' Your solution goes here '''`
- This placeholder comment indicates where additional code or logic can be inserted.
3. **Input Collection**:
- `length = float(input())`
- `width = float(input())`
- `height = float(input())`
- These lines prompt the user to input the dimensions of the pyramid's base and its height. The inputs are converted to floating-point numbers for accurate calculations.
4. **Output Statement**:
- `print('Volume for', length, width, height, "is:", calc_pyramid_volume(length, width, height))`
- This line outputs the calculated volume of the pyramid. It calls a function `calc_pyramid_volume` (presumably defined elsewhere, as it is not included in the snippet) with the dimensions entered by the user.
### Notes
- The code snippet includes a function to calculate the base area, but the logic to calculate the pyramid's volume (`calc_pyramid_volume`) is not shown and would need to be defined elsewhere in the educational material.
- The image does not contain any graphs or diagrams.
- The button labeled "Run" suggests this code is part of an executable notebook or educational platform that allows code execution within the interface.
This educational content helps learners understand how to interactively collect input in Python and use functions to perform calculations.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
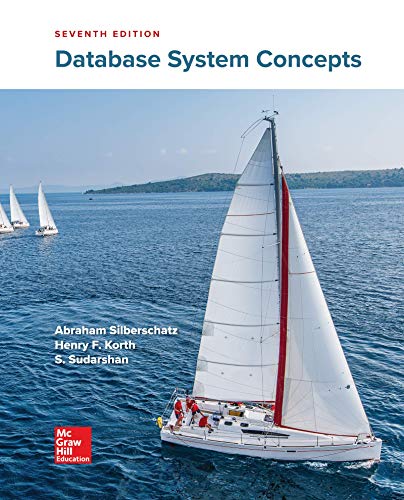
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
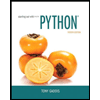
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
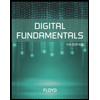
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
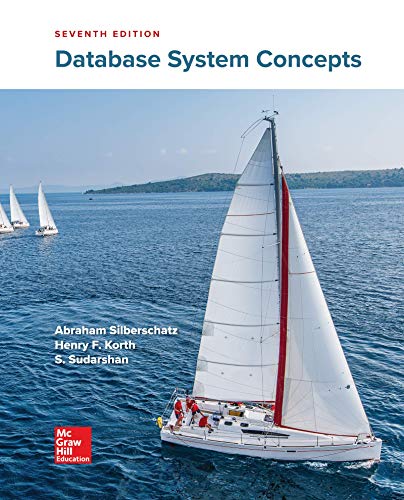
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
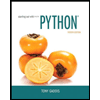
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
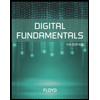
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
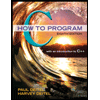
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
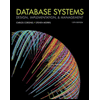
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
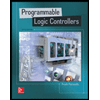
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education