Can you write this in C++??
Assignment 8 B: Hit Boxes (Part 2). Back in Assignment 2 (so long ago!) we created a simple program to determine what a hit box would be. Now we’re going to use that information to determine if two characters would collide based on those hit boxes.
You will create a Player class that takes in the following private attributes (as integers) when creates
Width
Height
X position
Y position
In addition to Getter methods for all four attributes, the Player class should also have the following methods:
MoveHorizontal(int x_delta)
◦ Takes in either a negative integer (for moving left) or a positive integer (for moving right). It should update the X position of the Player object
MoveVertical(int y_delta)
◦ Takes in either a negative integer (for moving down) or a positive integer (for moving up). It should update the Y position of the Player object
DidTheyCollide(Player otherPlayer)
◦ Takes in another player object, and returns true if they collided with each other (and false otherwise).
To detect collisions in the DidTheyCollide() method, you will use the following equation for AxisAligned Bounding Box collision detection:
[PseudoCode]
if(Player1’s X Coordinate < (Player2’s Width + X Coordinate) AND
(Player1’s Width + X Coordinate) > Player2’s X Coordinate AND
Player1’s Y Coordinate < (Player2’s Height + Y Coordinate) AND
(Player1’s Height + Y Coordinate) > Player2’s Y Coordinate)
{
PRINT(“We collided!”)
}
Source:
https://learnopengl.com/In-Practice/2D-Game/Collisions/Collision-detection
You will then create a second class, Assignment8B, that will operate as a driver class. It will create two Player objects based on user input, and then prompt the user to choose a Player to move. After the player moves, the program should check to see if the two Player objects have collided. The program should keep prompting the user to move a player until they collide.
(user inout is in bold)
Sample Output:
[Collision Tester]
Create Player 1
Enter X position: 0
Enter Y position: 0
Enter Player Hitbox Width: 10
Enter Player Hitbox Height: 10
Create Player 2
Enter X position: -10
Enter Y position: 0
Enter Player Hitbox Width: 5
Enter Player Hitbox Height: 14
Player 1 is at (0,0) and Player 2 is at (-10,0)
Which one do you want to move?
1
Which direction should Player 1 move (up, down, left, or right)?
Up
How far should Player 1 move?
2
Player 1 is at (0,2) and Player 2 is at (-10,0)
Which one do you want to move?
2
Which direction should Player 2 move (up, down, left, or right)?
Right
How far should Player 2 move?
12
Player 1 is at (0,2) and Player 2 is at (2,0)
They collided!
Program Ends

Step by step
Solved in 2 steps with 1 images

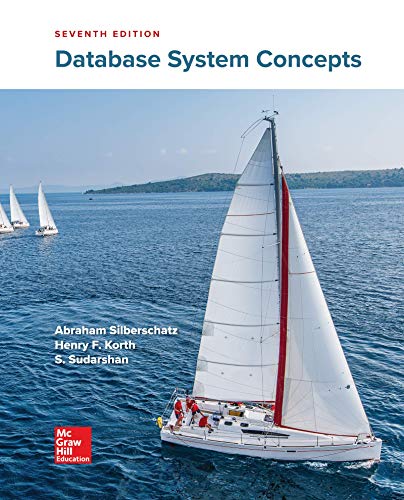
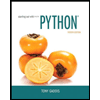
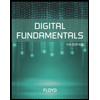
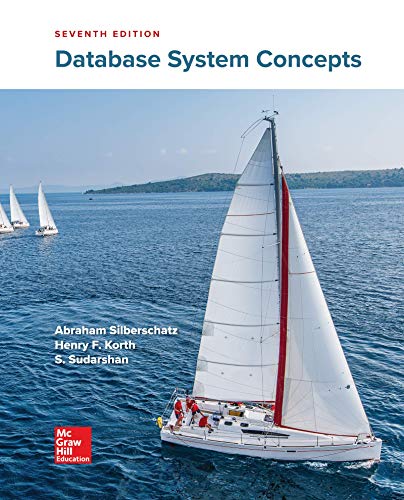
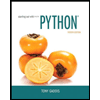
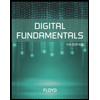
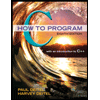
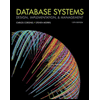
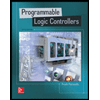