For each round, ... The computer rolls a 4-sided die but does not reveal the result. The user guesses if the computer rolled an even or an odd number. If the user guessed correctly, they win the round. Otherwise, the computer wins. o Play ends when all rounds have been played. Report the score and the winner. Pig: The object of this game is to be the first to score 100 points. It's a 2-person game, so have your program play against the computer. For each turn, roll one 10-sided die. If the roll is a 1 or a 2, the turn ends and no points are earned. If the roll is anything else, the player decides if they want to roll again and again to earn more points to add to their score. (The computer will always stop after it earns 20 points in a turn.) For example: The computer's turn: First roll = 6, 2« = 8, 3« = 2. The turn ends and the computer scores nothing. Your turn: 1* = 4, 2« = 8, 3« = 5, 4m = 9, 5ª = 9. You decide you want to quit, so you add the total of all these rolls to your score.
For each round, ... The computer rolls a 4-sided die but does not reveal the result. The user guesses if the computer rolled an even or an odd number. If the user guessed correctly, they win the round. Otherwise, the computer wins. o Play ends when all rounds have been played. Report the score and the winner. Pig: The object of this game is to be the first to score 100 points. It's a 2-person game, so have your program play against the computer. For each turn, roll one 10-sided die. If the roll is a 1 or a 2, the turn ends and no points are earned. If the roll is anything else, the player decides if they want to roll again and again to earn more points to add to their score. (The computer will always stop after it earns 20 points in a turn.) For example: The computer's turn: First roll = 6, 2« = 8, 3« = 2. The turn ends and the computer scores nothing. Your turn: 1* = 4, 2« = 8, 3« = 5, 4m = 9, 5ª = 9. You decide you want to quit, so you add the total of all these rolls to your score.
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
I need help creating a code for this program using python.

Transcribed Image Text:For each round, ...
The computer rolls a 4-sided die but does not reveal the result.
The user guesses if the computer rolled an even or an odd number.
If the user guessed correctly, they win the round. Otherwise, the computer
wins.
o Play ends when all rounds have been played. Report the score and the winner.
Pig: The object of this game is to be the first to score 100 points. It's a 2-person game, so have
your program play against the computer. For each turn, roll one 10-sided die. If the roll is a 1 or
a 2, the turn ends and no points are earned. If the roll is anything else, the player decides if they
want to roll again and again to earn more points to add to their score. (The computer will always
stop after it earns 20 points in a turn.) For example:
The computer's turn: First roll = 6, 2 = 8, 3d = 2. The turn ends and the computer scores
nothing.
Your turn: 1: = 4, 2« = 8, 3« = 5, 4 = 9, 5ª = 9. You decide you want to quit, so you add
the total of all these rolls to your score.
%3D

Transcribed Image Text:Program 10: Dice Games
There are several games you can play with dice, and the dice don't have to have 6 sides either. So, in this
program, you're going to create dice games.
12
8.
90
6.
Which means first you'll need a Die class containing:
A private variable for the number of sides
Getters and setters for the “sides" variable.
A constructor that takes as a parameter the number of sides wanted, with the default number
of sides being 6.
A method inside the class that returns the result of a roll (a random number representing one
side of the die)
I could explain how to get a random integer, which you'll need for this method, but
honestly the info at https://www.w3schools.com/python/ref random randint.asp is
great and even lets you try different things with their sample code.
Now when we write classes, it is common practice to make each class its own file. That way we don't
have to copy the code whenever we want to reuse it (which can cause bugs, as some of you have
learned from copying code!). Instead, we save the file then import the class into our main program (or
whatever else is using it). So save your Die class as a file called “Die.py" then put this line as the
beginning of your main program:
from Die import Die
And yes, both the Die class file and the main program file need to be in the same folder!
Once that's done, test your Die class. In your main program (under that import), create a die and roll it a
few times (printing the results) to make sure it works.
Working now? Ok, remove every line from the main program except that import line.
In the main program, you'll play a game. What game? It depends what the user wants to play!
Games:
Cho-Han: This is an old Japanese game.
Ask the user how many rounds they want to play.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

Recommended textbooks for you
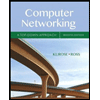
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
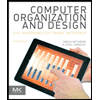
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
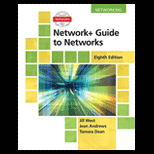
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
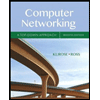
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
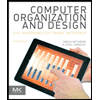
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
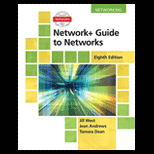
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
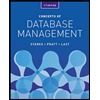
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
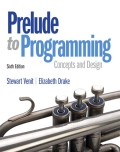
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
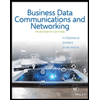
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY