Can you fix this please? with 5 53 5099 1223 567 17 4 1871 8069 3581 6841 #include using namespace std; const int SORT_MAX_SIZE = 8; bool IsPrimeRecur(int dividend, int divisor) { if (divisor == 1) { return true; } if (dividend % divisor == 0) { return false; } return IsPrimeRecur(dividend, divisor-1); } bool IsArrayPrimeRecur(int arr[], int size, int index) { cout << "Entering IsArrayPrimeRecur" << endl; if (index == size) { cout << "Leaving IsArrayPrimeRecur" << endl; return true; } if (!IsPrimeRecur(arr[index], arr[index]-1)) { cout << "Leaving IsArrayPrimeRecur" << endl; return false; } return IsArrayPrimeRecur(arr, size, index+1); } bool IsArrayPrimeIter(int arr[], int size) { cout << "Entering IsArrayPrimeIter" << endl; for (int i = 0; i < size; i++) { for (int j = 2; j < arr[i]; j++) { if (arr[i] % j == 0) { cout << "Leaving IsArrayPrimeIter" << endl; return false; } } } cout << "Leaving IsArrayPrimeIter" << endl; return true; } int main() { cout << "Please enter your input data on one line only." << endl; int size; cin >> size; int arr[SORT_MAX_SIZE]; for (int i = 0; i < size; i++) { cin >> arr[i]; } if (IsArrayPrimeIter(arr, size)) { cout << "Array was found to be prime using iteration" << endl; } else { cout << "Not a Prime Array using iteration" << endl; } if (IsArrayPrimeRecur(arr, size, 0)) { cout << "Array was found to be prime using recursion" << endl; } else { cout << "Not a Prime Array using recursion" << endl; } return 0; }
Can you fix this please? with
- 5 53 5099 1223 567 17
- 4 1871 8069 3581 6841
#include <iostream>
using namespace std;
const int SORT_MAX_SIZE = 8;
bool IsPrimeRecur(int dividend, int divisor) {
if (divisor == 1) {
return true;
}
if (dividend % divisor == 0) {
return false;
}
return IsPrimeRecur(dividend, divisor-1);
}
bool IsArrayPrimeRecur(int arr[], int size, int index) {
cout << "Entering IsArrayPrimeRecur" << endl;
if (index == size) {
cout << "Leaving IsArrayPrimeRecur" << endl;
return true;
}
if (!IsPrimeRecur(arr[index], arr[index]-1)) {
cout << "Leaving IsArrayPrimeRecur" << endl;
return false;
}
return IsArrayPrimeRecur(arr, size, index+1);
}
bool IsArrayPrimeIter(int arr[], int size) {
cout << "Entering IsArrayPrimeIter" << endl;
for (int i = 0; i < size; i++) {
for (int j = 2; j < arr[i]; j++) {
if (arr[i] % j == 0) {
cout << "Leaving IsArrayPrimeIter" << endl;
return false;
}
}
}
cout << "Leaving IsArrayPrimeIter" << endl;
return true;
}
int main() {
cout << "Please enter your input data on one line only." << endl;
int size;
cin >> size;
int arr[SORT_MAX_SIZE];
for (int i = 0; i < size; i++) {
cin >> arr[i];
}
if (IsArrayPrimeIter(arr, size)) {
cout << "Array was found to be prime using iteration" << endl;
} else {
cout << "Not a Prime Array using iteration" << endl;
}
if (IsArrayPrimeRecur(arr, size, 0)) {
cout << "Array was found to be prime using recursion" << endl;
} else {
cout << "Not a Prime Array using recursion" << endl;
}
return 0;
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 4 images

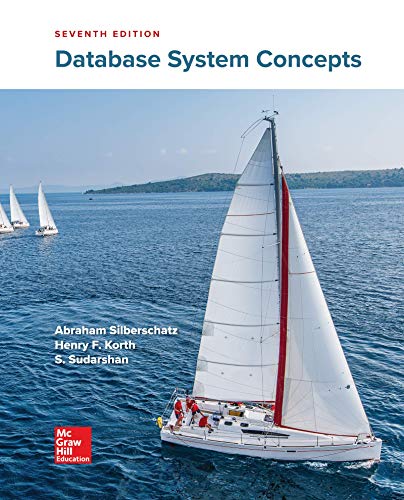
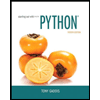
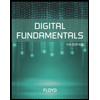
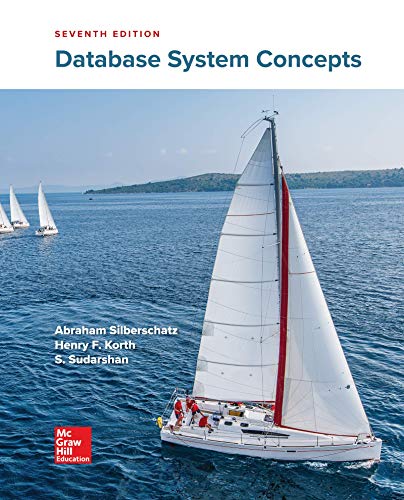
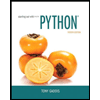
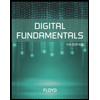
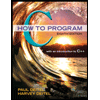
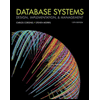
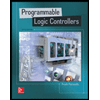