Can you explain line by line what the below C++ program is doing. Please and thank you C++ source code: #include <iostream>#include <thread>#include <atomic> std::atomic<int> counter(0); void incrementCounter(int iterations) { for (int i = 0; i < iterations; ++i) { counter.fetch_add(1, std::memory_order_relaxed); // std::memory_order_relaxed is used for simplicity, you may choose a different memory order based on your requirements }} int main() { const int numThreads = 5; const int iterationsPerThread = 100000; std::thread threads[numThreads]; // Launch threads for (int i = 0; i < numThreads; ++i) { threads[i] = std::thread(incrementCounter, iterationsPerThread); } // Join threads for (int i = 0; i < numThreads; ++i) { threads[i].join(); } std::cout << "Counter value: " << counter.load(std::memory_order_relaxed) << std::endl; return 0;}
Can you explain line by line what the below C++
Please and thank you
C++ source code:
#include <iostream>
#include <thread>
#include <atomic>
std::atomic<int> counter(0);
void incrementCounter(int iterations) {
for (int i = 0; i < iterations; ++i) {
counter.fetch_add(1, std::memory_order_relaxed);
// std::memory_order_relaxed is used for simplicity, you may choose a different memory order based on your requirements
}
}
int main() {
const int numThreads = 5;
const int iterationsPerThread = 100000;
std::thread threads[numThreads];
// Launch threads
for (int i = 0; i < numThreads; ++i) {
threads[i] = std::thread(incrementCounter, iterationsPerThread);
}
// Join threads
for (int i = 0; i < numThreads; ++i) {
threads[i].join();
}
std::cout << "Counter value: " << counter.load(std::memory_order_relaxed) << std::endl;
return 0;
}
Unlock instant AI solutions
Tap the button
to generate a solution
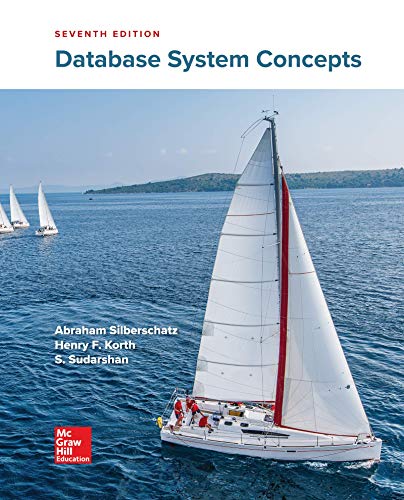
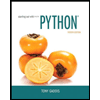
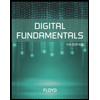
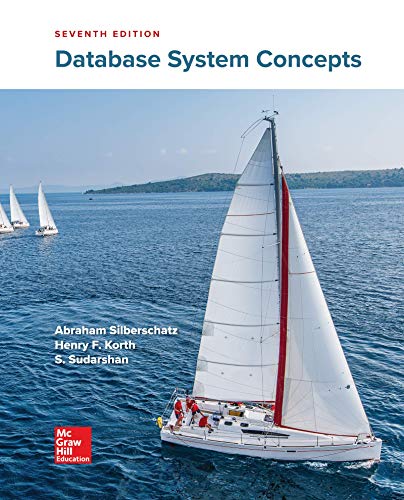
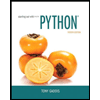
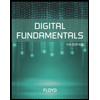
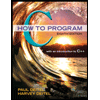
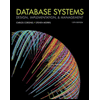
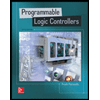