What would pseudocode look like for the following C++ application? C++ source code: #include <iostream> #include <thread> #include <mutex> using namespace std; // Define global mutex std::mutex mutex1; // Define countUp() function void countUp() { for(int i = 1; i <= 20; ++i) { // Lock mutex to protect shared resources std::lock_guard<std::mutex> lock(mutex1); // Print count std::cout << "Thread 1: Counting up -- " << i << std::endl; // Sleeping thread for a duration std::this_thread::sleep_for(std::chrono::milliseconds(400)); } } // Define countDown() function void countDown() { for(int i = 20; i >= 0; --i) { // Lock mutex to protect shared resources std::lock_guard<std::mutex> lock(mutex1); // Print count std::cout << "Thread 2: Counting down -- " << i << std::endl; // Sleeping thread for a duration std::this_thread::sleep_for(std::chrono::milliseconds(400)); } } // Main() function int main() { // Creating thread1 for countUp and joining it std::thread thread1(countUp); thread1.join(); // Creating thread2 for countDown and joining it std::thread thread2(countDown); thread2.join(); return 0; }
What would pseudocode look like for the following C++ application?
C++ source code:
#include <iostream>
#include <thread>
#include <mutex>
using namespace std;
// Define global mutex
std::mutex mutex1;
// Define countUp() function
void countUp() {
for(int i = 1; i <= 20; ++i) {
// Lock mutex to protect shared resources
std::lock_guard<std::mutex> lock(mutex1);
// Print count
std::cout << "Thread 1: Counting up -- " << i << std::endl;
// Sleeping thread for a duration
std::this_thread::sleep_for(std::chrono::milliseconds(400));
}
}
// Define countDown() function
void countDown() {
for(int i = 20; i >= 0; --i) {
// Lock mutex to protect shared resources
std::lock_guard<std::mutex> lock(mutex1);
// Print count
std::cout << "Thread 2: Counting down -- " << i << std::endl;
// Sleeping thread for a duration
std::this_thread::sleep_for(std::chrono::milliseconds(400));
}
}
// Main() function
int main() {
// Creating thread1 for countUp and joining it
std::thread thread1(countUp);
thread1.join();
// Creating thread2 for countDown and joining it
std::thread thread2(countDown);
thread2.join();
return 0;
}
Unlock instant AI solutions
Tap the button
to generate a solution
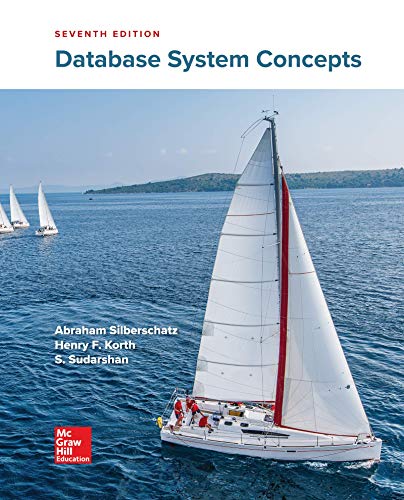
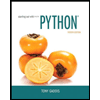
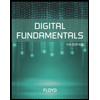
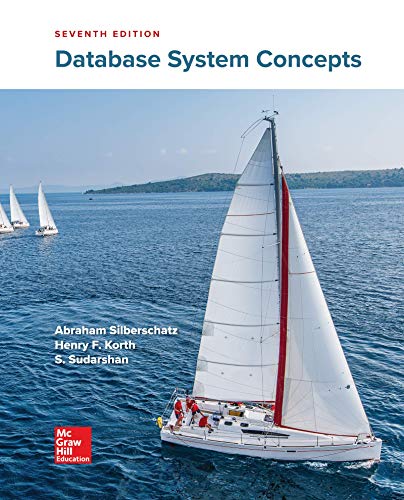
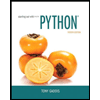
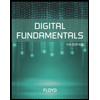
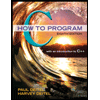
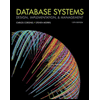
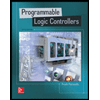