. For the code below, draw a picture of the program stack when the function partition() is called the first time. You only have to draw the part of the stack for quicksort() and partition(). # extracted from suquant's reply at # https://stackoverflow.com/questions/18262306/quicksort-with-python def partition(array, begin, end): pivot = begin for i in range(begin+1, end+1): if array[i] <= array[begin]: pivot += 1 array[i], array[pivot] = array[pivot], array[i] array[pivot], array[begin] = array[begin], array[pivot] return pivot def quicksort(array, begin, end): if begin >= end: return pivot = partition(array, begin, end) quicksort(array, begin, pivot-1) quicksort(array, pivot+1, end) # added calling code if __name__ == "__main__": mylist = [8, 2, 17, 4, 12] quicksort(mylist, 0, 4)
1. For the code below, draw a picture of the program stack when the function partition() is called the first time. You only have to draw the part of the stack for quicksort() and partition().
# extracted from suquant's reply at
# https://stackoverflow.com/questions/18262306/quicksort-with-python
def partition(array, begin, end):
pivot = begin
for i in range(begin+1, end+1):
if array[i] <= array[begin]:
pivot += 1
array[i], array[pivot] = array[pivot], array[i]
array[pivot], array[begin] = array[begin], array[pivot]
return pivot
def quicksort(array, begin, end):
if begin >= end:
return
pivot = partition(array, begin, end)
quicksort(array, begin, pivot-1)
quicksort(array, pivot+1, end)
# added calling code
if __name__ == "__main__":
mylist = [8, 2, 17, 4, 12]
quicksort(mylist, 0, 4)

Step by step
Solved in 2 steps with 1 images

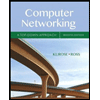
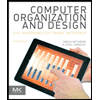
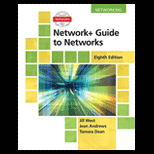
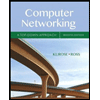
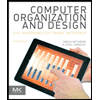
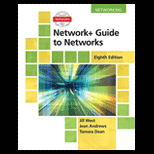
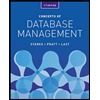
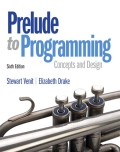
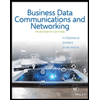