can someone help me with this in C++ In this program, you must implement I/O operator overloading (<< and >>) to match a shape's functionality. You must modify the following files: Triangle.h Implement the declaration for the overloaded I/O operator functions. Triangle.cpp Implement the calculateArea() function to find the area of the triangle. Implement the definitions for the overloaded I/O operator functions. The input function will take in two variables: The base and height (cin >> base >> height) Call the object's calculateArea() function after inputting the variables. The output function will send the following to the output stream (for base = 10 and height = 20): Triangle Object: Base: 10 Height: 20 Area is: 100 Square.h Implement the declaration for the overloaded I/O operator functions. Square.cpp Implement the calculateArea() function to find the area of the square. Implement the definitions for the overloaded I/O operator functions. The input function will take in two variables: The base and height (cin >> base >> height) Call the object's calculateArea() function after inputting the variables. The output function will send the following to the output stream (for base = 10 and height = 20): Square Object: Base: 10 Height: 20 Area is: 200 HINT: Print newline characters after every line (endl or "\n") Do not modify the main.cpp file. this is the code I have so far main.cpp #include "Triangle.h" #include "Square.h" #include using namespace std; int main() { //Do not modify return 0; } square.cpp #include "Square.h" #include using namespace std; Square::Square(){ base = 0; height = 0; } Square::Square(int b, int h) : base(b), height(h) { } void Square::setBase(int b){ base = b; } void Square::setHeight(int h){ height = h; } void Square::calculateArea(){ // calculate Area correctly depending on shape type } int Square::getBase() const{ return base; } int Square::getHeight() const{ return height; } double Square::getArea() const{ return area; } // Overloaded << operator // Overloaded >> operator square.h #ifndef SQUARE_H_ #define SQUARE_H_ #include using namespace std; class Square { public: Square(); Square(int b, int h); //Setters void setBase(int b); void setHeight(int h); //Getters int getBase() const; int getHeight() const; double getArea() const; void calculateArea(); // Overloaded << operator declaration // Overloaded >> operator declaration private: int base; int height; double area; }; #endif Triangle.cpp #include "Triangle.h" #include using namespace std; Triangle::Triangle(){ base = 0; height = 0; } Triangle::Triangle(int b, int h) : base(b), height(h) { } void Triangle::setBase(int b){ base = b; } void Triangle::setHeight(int h){ height = h; } void Triangle::calculateArea(){ // calculate Area correctly depending on shape type } int Triangle::getBase() const{ return base; } int Triangle::getHeight() const{ return height; } double Triangle::getArea() const{ return area; } // Overloaded << operator declaration // Overloaded >> operator declaration triangle.h #ifndef TRIANGLE_H_ #define TRIANGLE_H_ #include using namespace std; class Triangle { public: Triangle(); Triangle(int b, int h); //Setters void setBase(int b); void setHeight(int h); //Getters int getBase() const; int getHeight() const; double getArea() const; void calculateArea(); // Overloaded << operator declaration // Overloaded >> operator declaration private: int base; int height; double area; }; #endif
can someone help me with this in C++
In this program, you must implement I/O operator overloading (<< and >>) to match a shape's functionality.
You must modify the following files:
Triangle.h
- Implement the declaration for the overloaded I/O operator functions.
Triangle.cpp
- Implement the calculateArea() function to find the area of the triangle.
- Implement the definitions for the overloaded I/O operator functions.
- The input function will take in two variables: The base and height (cin >> base >> height)
- Call the object's calculateArea() function after inputting the variables.
The output function will send the following to the output stream (for base = 10 and height = 20):
Triangle Object: Base: 10 Height: 20 Area is: 100
Square.h
- Implement the declaration for the overloaded I/O operator functions.
Square.cpp
- Implement the calculateArea() function to find the area of the square.
- Implement the definitions for the overloaded I/O operator functions.
- The input function will take in two variables: The base and height (cin >> base >> height)
- Call the object's calculateArea() function after inputting the variables.
The output function will send the following to the output stream (for base = 10 and height = 20):
Square Object: Base: 10 Height: 20 Area is: 200
HINT: Print newline characters after every line (endl or "\n")
Do not modify the main.cpp file.
this is the code I have so far
main.cpp
#include "Triangle.h"
#include "Square.h"
#include <iostream>
using namespace std;
int main() {
//Do not modify
return 0;
}
square.cpp
#include "Square.h"
#include <iostream>
using namespace std;
Square::Square(){
base = 0;
height = 0;
}
Square::Square(int b, int h) : base(b), height(h) { }
void Square::setBase(int b){
base = b;
}
void Square::setHeight(int h){
height = h;
}
void Square::calculateArea(){
// calculate Area correctly depending on shape type
}
int Square::getBase() const{
return base;
}
int Square::getHeight() const{
return height;
}
double Square::getArea() const{
return area;
}
// Overloaded << operator
// Overloaded >> operator
square.h
#ifndef SQUARE_H_
#define SQUARE_H_
#include <iostream>
using namespace std;
class Square {
public:
Square();
Square(int b, int h);
//Setters
void setBase(int b);
void setHeight(int h);
//Getters
int getBase() const;
int getHeight() const;
double getArea() const;
void calculateArea();
// Overloaded << operator declaration
// Overloaded >> operator declaration
private:
int base;
int height;
double area;
};
#endif
Triangle.cpp
#include "Triangle.h"
#include <iostream>
using namespace std;
Triangle::Triangle(){
base = 0;
height = 0;
}
Triangle::Triangle(int b, int h) : base(b), height(h) { }
void Triangle::setBase(int b){
base = b;
}
void Triangle::setHeight(int h){
height = h;
}
void Triangle::calculateArea(){
// calculate Area correctly depending on shape type
}
int Triangle::getBase() const{
return base;
}
int Triangle::getHeight() const{
return height;
}
double Triangle::getArea() const{
return area;
}
// Overloaded << operator declaration
// Overloaded >> operator declaration
triangle.h
#ifndef TRIANGLE_H_
#define TRIANGLE_H_
#include <iostream>
using namespace std;
class Triangle {
public:
Triangle();
Triangle(int b, int h);
//Setters
void setBase(int b);
void setHeight(int h);
//Getters
int getBase() const;
int getHeight() const;
double getArea() const;
void calculateArea();
// Overloaded << operator declaration
// Overloaded >> operator declaration
private:
int base;
int height;
double area;
};
#endif

Trending now
This is a popular solution!
Step by step
Solved in 7 steps with 1 images

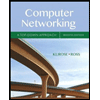
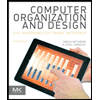
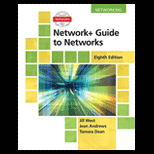
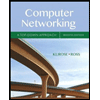
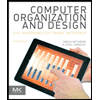
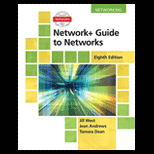
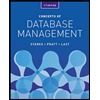
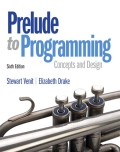
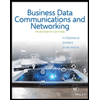