} * Calculates a tax payer's tax assuming they are a corporation. * @precondition payer != null AND payer.isCorporation ()==true @postcondition none @param payer the tax payer * @return the tax assuming the payer is a corporation; should be zero if no (or negative) income public static final double CORPORATE_TAX_RATE 0.21; public double tax() { return -1; } public double calculate Corporate Income Tax (TaxPayer payer) { if(tax() <= 0) return tax(); return -1;
} * Calculates a tax payer's tax assuming they are a corporation. * @precondition payer != null AND payer.isCorporation ()==true @postcondition none @param payer the tax payer * @return the tax assuming the payer is a corporation; should be zero if no (or negative) income public static final double CORPORATE_TAX_RATE 0.21; public double tax() { return -1; } public double calculate Corporate Income Tax (TaxPayer payer) { if(tax() <= 0) return tax(); return -1;
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
This is what I have so far I am trying to calculate the tax as the TaxPayer's income (returned by its getIncome method) times a corporate tax rate of 21% (0.21). This is what I have so far. I also would like to save the variable in a double named tax. I am having trouble figuring out to call the TaxPayers income to multiply it by the tax rate.

Transcribed Image Text:The code displays a Java class named `TaxPayer`, which is used to model individuals or businesses that pay taxes. Here’s a detailed explanation and transcription for an educational context:
### Code Explanation:
1. **Class Definition:**
- `public class TaxPayer` declares a class named `TaxPayer`.
- The class has four private fields: `name`, `age`, `isCorporation`, and `income`, representing the taxpayer's details.
2. **Constructor:**
- The constructor `public TaxPayer(String name, int age, double income, boolean isCorporation)` initializes a new instance of `TaxPayer`.
- Preconditions and postconditions ensure proper initialization:
- **Precondition:** The `name` must not be null or empty, and `age` must be non-negative.
- **Postcondition:** The `getName()`, `getAge()`, and `getIsBusiness()` methods must return the values provided during initialization.
3. **Parameters:**
- `@param name`: Represents the name of the person or business.
- `@param age`: Represents the individual's age (ignored if it's a business).
- `@param income`: Represents the annual income.
- `@param isCorporation`: Boolean indicating if the taxpayer is a business.
4. **Methods:**
- `public String getName()`: Returns the name of the taxpayer.
- `public int getAge()`: Returns the age of the taxpayer.
### Code Transcription:
```java
public class TaxPayer {
private String name;
private int age;
private boolean isCorporation;
private double income;
/**
* Creates a new taxpayer.
*
* @precondition name != null AND !name.isEmpty() AND age >= 0
* @postcondition getName()==name AND getAge()==age AND getIsBusiness()==isBusiness
*
* @param name the person or business' name
* @param age the person's age (ignored if it is a business)
* @param income the annual income
* @param isCorporation true if the taxpayer is a business, false otherwise
*/
public TaxPayer(String name, int age, double income, boolean isCorporation) {
super();
this.name = name;
this.age = age;
this.isCorporation =

Transcribed Image Text:```java
public TaxCalculator() {
}
/**
* Calculates a tax payer's tax assuming they are a corporation.
*
* @precondition payer != null AND payer.isCorporation()==true
* @postcondition none
*
* @param payer the tax payer
* @return the tax assuming the payer is a corporation; should be zero if no (or negative) income
*/
public static final double CORPORATE_TAX_RATE = 0.21;
public double tax() {
return -1;
}
public double calculateCorporateIncomeTax(TaxPayer payer) {
if (tax() <= 0)
return tax();
return -1;
}
```
### Explanation
This code defines a class responsible for calculating the tax for corporations. Here’s a breakdown of its components:
- **Class and Method Descriptions:**
- **Constructor:** `public TaxCalculator()` is a default constructor for the `TaxCalculator` class.
- **JavaDoc Comment:**
- Provides important information about the `calculateCorporateIncomeTax` function:
- **Purpose:** Calculates a taxpayer's tax, assuming they are a corporation.
- **Precondition:** The taxpayer (`payer`) must not be null and must be confirmed as a corporation (`payer.isCorporation() == true`).
- **Postcondition:** None specified, indicating no particular condition after execution.
- **Parameters:** Takes a `TaxPayer` object named `payer`.
- **Return:** Should return the tax amount assuming the payer is a corporation; expected to be zero if there's no income or if the income is negative.
- **Fields and Constants:**
- `CORPORATE_TAX_RATE`: A static final constant representing the corporate tax rate (21%).
- **Methods:**
- `public double tax()`: A method that currently returns -1, possibly as a placeholder or error condition.
- `public double calculateCorporateIncomeTax(TaxPayer payer)`:
- Calls the `tax()` method.
- Returns `tax()` if it is less than or equal to zero, otherwise returns -1.
### Code Functionality
The `TaxCalculator` class is designed to calculate corporate taxes using a defined corporate tax rate. However, the current implementation does not compute taxes correctly due to placeholder logic in the `calculateCorporateIncomeTax` method which returns -1 instead of a calculated value.
The code requires further implementation to perform
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 4 images

Similar questions
Recommended textbooks for you
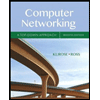
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
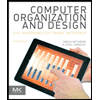
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
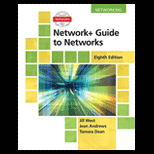
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
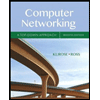
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
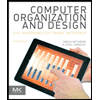
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
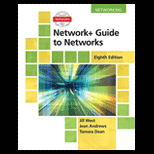
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
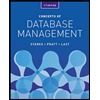
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
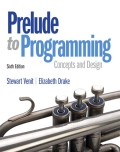
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
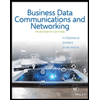
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY