C++ Shape is an abstract class with a pure virtual function: Area(). (2) Circle is inherited from Shape, with a data member Radius, and a member function Area() to get the area of a circle. (3) Triangle is inherited from Shape, with two data members Bottom-length and Height, and a member function Area() to get the area of a triangle. Please complete the definition of Shape, Circle and Triangle, and define other necessary functions, to let a user can use Shape, Circle and Triangle as follows: void main( ) { Shape* p = new Circle(2); cout << "The area of the circle is: " << PrintArea(*p) << endl; Triangle triangle (3,4); cout << "The area of the triangle is: " << PrintArea(triangle) << endl; delete p; } The outputs: The area of the circle is: 12.56 The area of the triangle is: 6 //Your codes with necessary explanations: My code: #include using namespace std; class Shape { public: double area; virtual void Area() = 0; void PrintArea(); }; class Triangle : public Shape { double Bottom_length, Height; public: Triangle(double l, double h) { Bottom_length=l; Height = h; } void Area() { area= 0.5* Bottom_length*Height; } void PrintArea(){ cout<
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
C++
Shape is an abstract class with a pure virtual function: Area(). (2) Circle is inherited from Shape, with a data member Radius, and a member function Area() to get the area of a circle. (3) Triangle is inherited from Shape, with two data members Bottom-length and Height, and a member function Area() to get the area of a triangle.
Please complete the definition of Shape, Circle and Triangle, and define other necessary functions, to let a user can use Shape, Circle and Triangle as follows:
void main( )
{
Shape* p = new Circle(2);
cout << "The area of the circle is: " << PrintArea(*p) << endl;
Triangle triangle (3,4);
cout << "The area of the triangle is: " << PrintArea(triangle) << endl;
delete p;
}
The outputs:
The area of the circle is: 12.56
The area of the triangle is: 6
//Your codes with necessary explanations:
My code:
#include <iostream>
using namespace std;
class Shape
{
public:
double area;
virtual void Area() = 0;
void PrintArea();
};
class Triangle : public Shape
{
double Bottom_length, Height;
public:
Triangle(double l, double h)
{
Bottom_length=l;
Height = h;
}
void Area()
{
area= 0.5* Bottom_length*Height;
}
void PrintArea(){
cout<<area;
}
};
class Circle : public Shape
{
double Radius;
public:
Circle(double r)
{
Radius=r;
}
void Area(){
area=3.14*Radius*Radius;
}
void PrintArea(){
cout<<area;
}
};
Void main()
{
Shape* p = new Circle(2);
cout << "The area of the circle is: " << PrintArea(*p) << endl;
Triangle triangle (3,4);
cout << "The area of the triangle is: " << PrintArea(triangle) << endl;
delete p;
}
Please remove errors and run this code

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 3 images

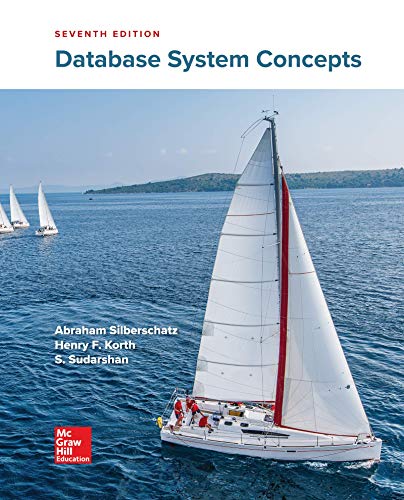
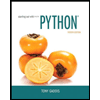
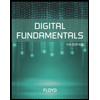
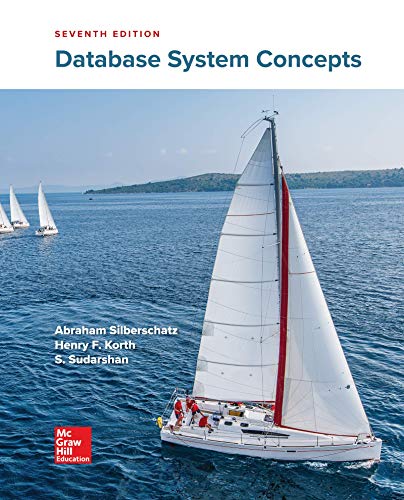
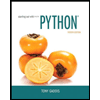
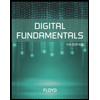
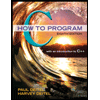
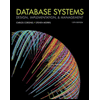
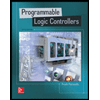