C Programming Could you update my code using the code I provided. I need help with 5. #include #include #define TRUE 1 #define FALSE 0 #define NAME 20 #define ROW 8 #define COL 8 #define SPACE ' ' #define PLAYER_X 1 #define PLAYER_O 2 #define ZERO 0 #define ONE 1 #define TWO 2 #define FOUR 4 #define INVALID -1 // function prototypes void welcomeScreen (); void displayExplicitBoard(); void clearScreen(); void playGame(); // main function int main() { // call function welcomeScreen welcomeScreen(); // call function clearScreen clearScreen(); // call function displayExplicitBoard // displayExplicitBoard(); // call function playGame playGame(); // program executed successfully return 0; } // welcomeScreen function displays the Othello logo and rules of the game void welcomeScreen () { printf ("\t\t OOOO TTTTTT HH HH EEEEEE LL LL OOOO \n"); printf ("\t\tOO OO TT HH HH EE LL LL OO OO \n"); printf ("\t\tOO OO TT HHHHHH EEEE LL LL OO OO \n"); printf ("\t\tOO OO TT HH HH EE LL LL OO OO \n"); printf ("\t\t OOOO TT HH HH EEEEEE LLLLLLL LLLLLL OOOO \n"); printf ("\n\n");// printf ("OTHELLO GAME RULES:\n"); printf("\t1. A square 8 x 8 board\n"); printf("\t2. 64 discs colored black (X) on one side and white (O) on the opposite side.\n"); printf("\t3. The board will start with 2 black discs (X) and 2 white discs (O) at the center of the board.\n"); printf("\t4. They are arranged with black (X) forming a North-East to South-West direction. White (O) is forming a North-West to South-East direction\n"); printf("\t5. The goal is to get the majority of color discs on the board at the end of the game.\n"); printf("\t6. Each player gets 32 discs and black (X) always starts the game.\n"); printf("\t7. The game alternates between white (O) and black (X) until one player can not make a valid move to outflank the opponent or both players have no valid moves.\n"); printf("\t8. When a player has no valid moves, they pass their turn and the opponent continues.\n"); printf("\t9. A player cannot voluntarily forfeit their turn.\n"); printf("\t10. When both players can not make a valid move the game ends.\n"); } // function displayExplicitBoard displays a hardcoded version of an Othello board void displayExplicitBoard() { printf("|-----------------------------------------------------|\n"); printf("| | A | B | C | D | E | F | G | H |\n"); printf("|-----------------------------------------------------|\n"); printf("| 1 | | | | | | | | |\n"); printf("|-----------------------------------------------------|\n"); printf("| 2 | | | | | | | | |\n"); printf("|-----------------------------------------------------|\n"); printf("| 3 | | | | | | | | |\n"); printf("|-----------------------------------------------------|\n"); printf("| 4 | | | | O | X | | | |\n"); printf("|-----------------------------------------------------|\n"); printf("| 5 | | | | X | O | | | |\n"); printf("|-----------------------------------------------------|\n"); printf("| 6 | | | | | | | | |\n"); printf("|-----------------------------------------------------|\n"); printf("| 7 | | | | | | | | |\n"); printf("|-----------------------------------------------------|\n"); printf("| 8 | | | | | | | | |\n"); printf("|-----------------------------------------------------|\n"); } // function clearScreen clears the screen for display purposes void clearScreen() { printf("\n\t\t\t\tHit to continue!\n"); char enter; scanf("%c", &enter ); // send the clear screen command Windows system("cls"); // send the clear screen command for UNIX flavor operating systems // system("clear"); } void playGame() { // get player names char playerX[NAME]; char playerO[NAME]; // black (X) always goes first int currentPlayer = PLAYER_X; int loop = ZERO; printf("Player X, please enter your name\n"); scanf("%s", playerX); printf("Player O, please enter your name\n"); scanf("%s", playerO); printf("%s and %s, let's play Othello!\n", playerX, playerO); while(loop < FOUR) { // call function displayExplicitBoard displayExplicitBoard(); // switch players for each move if(currentPlayer == PLAYER_X) { printf("%s, it is your turn\n", playerX); // switch players currentPlayer = PLAYER_O; } else if(currentPlayer == PLAYER_O) { printf("%s, it is your turn\n", playerO); currentPlayer = PLAYER_X; } system("pause"); loop++; } }
C Programming
Could you update my code using the code I provided. I need help with 5.
#include <stdio.h>
#include <stdlib.h>
#define TRUE 1
#define FALSE 0
#define NAME 20
#define ROW 8
#define COL 8
#define SPACE ' '
#define PLAYER_X 1
#define PLAYER_O 2
#define ZERO 0
#define ONE 1
#define TWO 2
#define FOUR 4
#define INVALID -1
// function prototypes
void welcomeScreen ();
void displayExplicitBoard();
void clearScreen();
void playGame();
// main function
int main()
{
// call function welcomeScreen
welcomeScreen();
// call function clearScreen
clearScreen();
// call function displayExplicitBoard
// displayExplicitBoard();
// call function playGame
playGame();
// program executed successfully
return 0;
}
// welcomeScreen function displays the Othello logo and rules of the game
void welcomeScreen ()
{
printf ("\t\t OOOO TTTTTT HH HH EEEEEE LL LL OOOO \n");
printf ("\t\tOO OO TT HH HH EE LL LL OO OO \n");
printf ("\t\tOO OO TT HHHHHH EEEE LL LL OO OO \n");
printf ("\t\tOO OO TT HH HH EE LL LL OO OO \n");
printf ("\t\t OOOO TT HH HH EEEEEE LLLLLLL LLLLLL OOOO \n");
printf ("\n\n");//
printf ("OTHELLO GAME RULES:\n");
printf("\t1. A square 8 x 8 board\n");
printf("\t2. 64 discs colored black (X) on one side and white (O) on the opposite side.\n");
printf("\t3. The board will start with 2 black discs (X) and 2 white discs (O) at the center of the board.\n");
printf("\t4. They are arranged with black (X) forming a North-East to South-West direction. White (O) is forming a North-West to South-East direction\n");
printf("\t5. The goal is to get the majority of color discs on the board at the end of the game.\n");
printf("\t6. Each player gets 32 discs and black (X) always starts the game.\n");
printf("\t7. The game alternates between white (O) and black (X) until one player can not make a valid move to outflank the opponent or both players have no valid moves.\n");
printf("\t8. When a player has no valid moves, they pass their turn and the opponent continues.\n");
printf("\t9. A player cannot voluntarily forfeit their turn.\n");
printf("\t10. When both players can not make a valid move the game ends.\n");
}
// function displayExplicitBoard displays a hardcoded version of an Othello board
void displayExplicitBoard()
{
printf("|-----------------------------------------------------|\n");
printf("| | A | B | C | D | E | F | G | H |\n");
printf("|-----------------------------------------------------|\n");
printf("| 1 | | | | | | | | |\n");
printf("|-----------------------------------------------------|\n");
printf("| 2 | | | | | | | | |\n");
printf("|-----------------------------------------------------|\n");
printf("| 3 | | | | | | | | |\n");
printf("|-----------------------------------------------------|\n");
printf("| 4 | | | | O | X | | | |\n");
printf("|-----------------------------------------------------|\n");
printf("| 5 | | | | X | O | | | |\n");
printf("|-----------------------------------------------------|\n");
printf("| 6 | | | | | | | | |\n");
printf("|-----------------------------------------------------|\n");
printf("| 7 | | | | | | | | |\n");
printf("|-----------------------------------------------------|\n");
printf("| 8 | | | | | | | | |\n");
printf("|-----------------------------------------------------|\n");
}
// function clearScreen clears the screen for display purposes
void clearScreen()
{
printf("\n\t\t\t\tHit <ENTER> to continue!\n");
char enter;
scanf("%c", &enter );
// send the clear screen command Windows
system("cls");
// send the clear screen command for UNIX flavor
// system("clear");
}
void playGame()
{
// get player names
char playerX[NAME];
char playerO[NAME];
// black (X) always goes first
int currentPlayer = PLAYER_X;
int loop = ZERO;
printf("Player X, please enter your name\n");
scanf("%s", playerX);
printf("Player O, please enter your name\n");
scanf("%s", playerO);
printf("%s and %s, let's play Othello!\n", playerX, playerO);
while(loop < FOUR)
{
// call function displayExplicitBoard
displayExplicitBoard();
// switch players for each move
if(currentPlayer == PLAYER_X)
{
printf("%s, it is your turn\n", playerX);
// switch players
currentPlayer = PLAYER_O;
}
else if(currentPlayer == PLAYER_O)
{
printf("%s, it is your turn\n", playerO);
currentPlayer = PLAYER_X;
}
system("pause");
loop++;
}
}


The correct answer for the above mentioned question is given in the following steps for your reference.
Step by step
Solved in 4 steps with 3 images

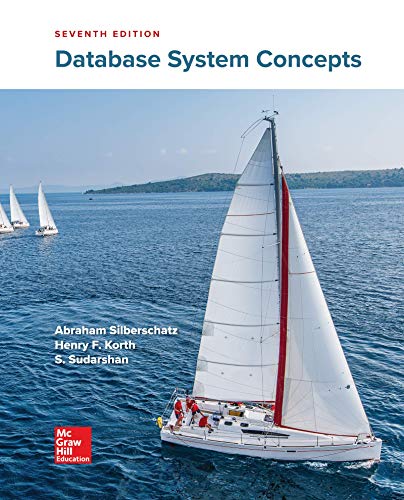
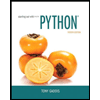
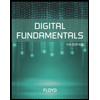
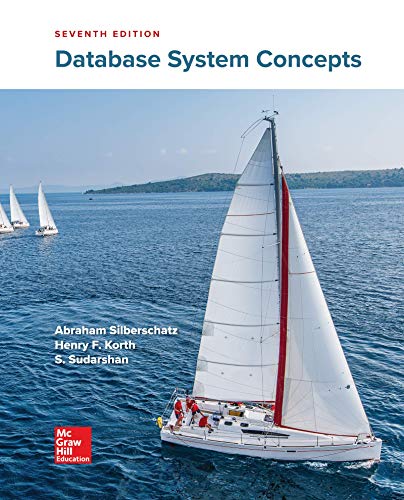
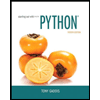
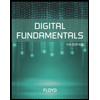
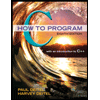
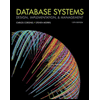
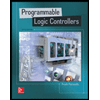