write this in pseudocode #4 – In pseudocode, write a for-loop that decreases from 100 to 2 by 2s. Inside the loop, print the value of the loop variable unless the value is from 60 to 80 (inclusive). Use only 1 if-statement. Do NOT write Python code! Write your answer below: #5 – Review the decision structure given below. If myAnswer > 20 Then myAnswer = myAnswer - 25 Else If myAnswer <= 5 Then If myAnswer < 0 Then myAnswer = 10 If myAnswer < 3 Then myAnswer += 10 Else myAnswer += 1 Else myAnswer = 9 If myAnswer < 10 Then myAnswer += 1 Display myAnswer What are the results displayed when the decision structure above executes for each given value below: If myAnswer = 6 then myAnswer = myAnswer = 15 then myAnswer = myAnswer = 30 then myAnswer = myAnswer = 2 then myAnswer = myAnswer = -1 then myAnswer = #6 – 2-dimensional arrays can be thought of as containing rows and columns. What are the subscript values for a 2-dimensional array? Enter them in the array below (first set given) [0,0] [ ] [ ] [ ] [ ] [ ] [ ] [ ] [ ] [ ] [ ] [ ] [ ] [ ] [ ]
Max Function
Statistical function is of many categories. One of them is a MAX function. The MAX function returns the largest value from the list of arguments passed to it. MAX function always ignores the empty cells when performing the calculation.
Power Function
A power function is a type of single-term function. Its definition states that it is a variable containing a base value raised to a constant value acting as an exponent. This variable may also have a coefficient. For instance, the area of a circle can be given as:
write this in pseudocode
#4 – In pseudocode, write a for-loop that decreases from 100 to 2 by 2s. Inside the loop, print the value of the loop variable unless the value is from 60 to 80 (inclusive). Use only 1
if-statement. Do NOT write Python code!
Write your answer below:
#5 – Review the decision structure given below.
If myAnswer > 20 Then
myAnswer = myAnswer - 25
Else If myAnswer <= 5 Then
If myAnswer < 0 Then
myAnswer = 10
If myAnswer < 3 Then
myAnswer += 10
Else
myAnswer += 1
Else
myAnswer = 9
If myAnswer < 10 Then
myAnswer += 1
Display myAnswer
What are the results displayed when the decision structure above executes for each given value below:
If myAnswer = 6 then myAnswer =
myAnswer = 15 then myAnswer =
myAnswer = 30 then myAnswer =
myAnswer = 2 then myAnswer =
myAnswer = -1 then myAnswer =
#6 – 2-dimensional arrays can be thought of as containing rows and columns. What are the subscript values for a 2-dimensional array?
Enter them in the array below (first set given)
[0,0] [ ] [ ]
[ ] [ ] [ ]
[ ] [ ] [ ]
[ ] [ ] [ ]
[ ] [ ] [ ]

Trending now
This is a popular solution!
Step by step
Solved in 4 steps

In Pseudocode
#7 – List 4 main data types we used in this course and describe the data that is stored in each one. List examples of variable names that make sense for each data type.
#8 – Debug this input validation function and fix it. There is a lot wrong with it!
Function Integer getNumberGreaterThanX(msg, x)
myInteger = getInteger(msg)
While myInteger < x
Display "The number must be < “, myInteger , “. Try again."
Input x
End While
End Function
Write the corrected function below:
#9 – Write a validation function based on the provided variables and call statement below. The validation function should return an Integer value greater than zero or print an error message until an acceptable value is entered.
Declare Integer positiveValue = 0
Declare String message = "Enter the year you were born (no negative numbers!)"
positiveValue = getNumberGreaterThanZero(message)
Write the function definition below:
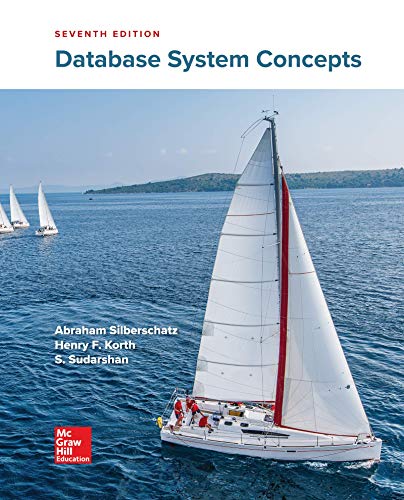
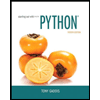
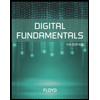
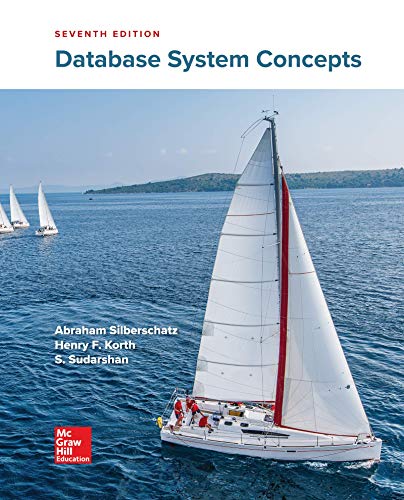
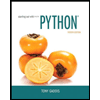
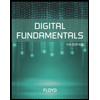
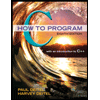
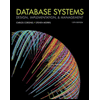
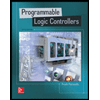