C PROGRAM BEING USED: In the following code, how do I replace the Merge Sort code with the qsort() standard library function? The sorting function in the code should be utilizing the qsort() function and not the Merge Sort code. //include reuired headr files #include //this header is used for srand() and rand() functions #include //this header file is used for time() function #include //main() function int main() { //declare array and variables int a[100], number, i, j, temp; // Use current time as // seed for random generator srand(time(0)); //create random number between 0 to 99 and assign to array for(i = 1; i <=100; i++) a[i]=rand() % 99 + 1; //print source array which is not sorted printf("Source Array:\n"); for(i = 1; i <= 100; i++) { //assign array value to n int n=a[i]; //declare count variable and initialise to 0 int count=0; //this loop counts numer of didig in number while (n != 0) { n /= 10; // n = n/10 ++count; } //if count is 1 then it append 0 at starting when printing if(count==1) { printf("0%d ", a[i]); } //otherwise it print number else { printf("%d ",a[i]); } //display 20 numbers in each line if(i%20==0) { printf("\n"); } } //this is used for sorting using selection sort //set i to first location and after each iteration increment the i for(i = 1; i <= 100; i++) { //set j to next of i value //compare all next values in array with i value for(j = i + 1; j <= 100; j++) { //if a[i] value is greater than a[j] then swap the element if(a[i] > a[j]) { temp = a[i]; a[i] = a[j]; a[j] = temp; } } } //print sorted array printf("\nDestination array:\n"); for(i = 1; i <= 100; i++) { //assign array value to n int n=a[i]; //declare count variable and initialise to 0 int count=0; //this loop counts numer of didig in number while (n != 0) { n /= 10; // n = n/10 ++count; } //if count is 1 then it append 0 at starting when printing if(count==1) { printf("0%d ", a[i]); } //otherwise it print number
C PROGRAM BEING USED: In the following code, how do I replace the Merge Sort code with the qsort() standard library function? The sorting function in the code should be utilizing the qsort() function and not the Merge Sort code.
//include reuired headr files
#include <stdio.h>
//this header is used for srand() and rand() functions
#include <stdlib.h>
//this header file is used for time() function
#include <time.h>
//main() function
int main()
{
//declare array and variables
int a[100], number, i, j, temp;
// Use current time as
// seed for random generator
srand(time(0));
//create random number between 0 to 99 and assign to array
for(i = 1; i <=100; i++)
a[i]=rand() % 99 + 1;
//print source array which is not sorted
printf("Source Array:\n");
for(i = 1; i <= 100; i++) {
//assign array value to n
int n=a[i];
//declare count variable and initialise to 0
int count=0;
//this loop counts numer of didig in number
while (n != 0)
{
n /= 10; // n = n/10
++count;
}
//if count is 1 then it append 0 at starting when printing
if(count==1)
{
printf("0%d ", a[i]);
}
//otherwise it print number
else
{
printf("%d ",a[i]);
}
//display 20 numbers in each line
if(i%20==0)
{
printf("\n");
}
}
//this is used for sorting using selection sort
//set i to first location and after each iteration increment the i
for(i = 1; i <= 100; i++) {
//set j to next of i value
//compare all next values in array with i value
for(j = i + 1; j <= 100; j++) {
//if a[i] value is greater than a[j] then swap the element
if(a[i] > a[j]) {
temp = a[i];
a[i] = a[j];
a[j] = temp;
}
}
}
//print sorted array
printf("\nDestination array:\n");
for(i = 1; i <= 100; i++) {
//assign array value to n
int n=a[i];
//declare count variable and initialise to 0
int count=0;
//this loop counts numer of didig in number
while (n != 0)
{
n /= 10; // n = n/10
++count;
}
//if count is 1 then it append 0 at starting when printing
if(count==1)
{
printf("0%d ", a[i]);
}
//otherwise it print number
else
{
printf("%d ",a[i]);
}
//display 20 numbers in each line
if(i%20==0)
{
printf("\n");
}
}
printf("\n");
return 0;
}
The output of the program should look remarkably similar to the image output.


Step by step
Solved in 2 steps with 1 images

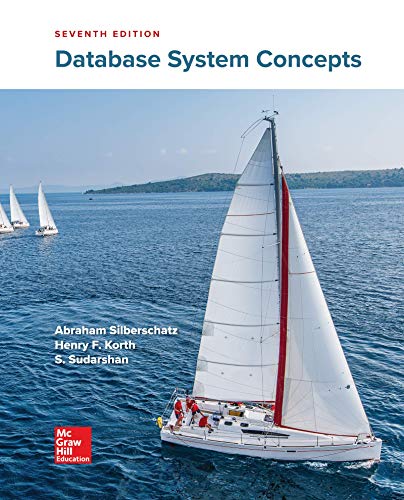
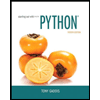
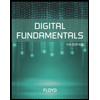
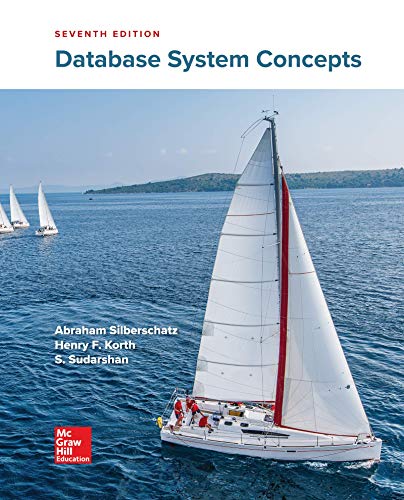
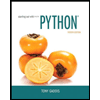
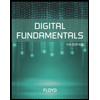
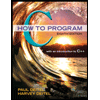
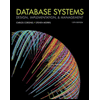
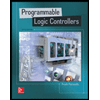