1. Using the following code c++ please insert a merge sort 2.compare the first code with a counter sort to see wish one is faster. #include using namespace std; // PLACE NAME HERE // Function prototypes void bubbleSortArray(int[], int); const int SIZE = 5; int main() { int values[SIZE] = { 9,2,0,11,5 }; // Displays the array before sorting cout << "The values before the bubble sort is performed are: "; for (int count = 0; count < SIZE; count++) cout << values[count] << " "; cout << endl; // Sort the array in ascending order using bubble sort algorithm bubbleSortArray(values, SIZE); // Display the array after sorting cout << "\n\nThe values after the bubble sort is performed are: "; for (int count = 0; count < SIZE; count++) cout << values[count] << " "; return 0; } //****************************************************************** // bubbleSortArray // // task: to sort values of an array in ascending order // data in: the array, the array size // data out: the sorted array // //****************************************************************** void bubbleSortArray(int array[], int elems) { bool swap; int temp; int bottom = elems - 1; // Bottom indicates the end part of the // array where the largest values have // settled in order do { swap = false; for (int count = 0; count < bottom; count++) { if (array[count] > array[count + 1]) { // The next three lines swap the two elements temp = array[count]; array[count] = array[count + 1]; array[count + 1] = temp; swap = true; // Indicates that a swap occurred } // Display array at each each step cout << "\nThe values are: TO BE IMPLEMENTED"; // Add code here to diplay the complete array at each step // Hint: for-loop similar to the one in the main() function for (int count = 0; count < SIZE; count++) cout << array[count] << " "; } bottom--; // Bottom is decremented by 1 since each pass through // the array adds one more value that is set in order // Loop repeats until a pass through the array with no swaps occurs } while (swap != false); }
1. Using the following code c++ please insert a merge sort
2.compare the first code with a counter sort to see wish one is faster.
#include<iostream>
using namespace std;
// PLACE NAME HERE
// Function prototypes
void bubbleSortArray(int[], int);
const int SIZE = 5;
int main()
{
int values[SIZE] = { 9,2,0,11,5 };
// Displays the array before sorting
cout << "The values before the bubble sort is performed are: ";
for (int count = 0; count < SIZE; count++)
cout << values[count] << " ";
cout << endl;
// Sort the array in ascending order using bubble sort
bubbleSortArray(values, SIZE);
// Display the array after sorting
cout << "\n\nThe values after the bubble sort is performed are: ";
for (int count = 0; count < SIZE; count++)
cout << values[count] << " ";
return 0;
}
//******************************************************************
// bubbleSortArray
//
// task: to sort values of an array in ascending order
// data in: the array, the array size
// data out: the sorted array
//
//******************************************************************
void bubbleSortArray(int array[], int elems)
{
bool swap;
int temp;
int bottom = elems - 1; // Bottom indicates the end part of the
// array where the largest values have
// settled in order
do
{
swap = false;
for (int count = 0; count < bottom; count++)
{
if (array[count] > array[count + 1])
{ // The next three lines swap the two elements
temp = array[count];
array[count] = array[count + 1];
array[count + 1] = temp;
swap = true; // Indicates that a swap occurred
}
// Display array at each each step
cout << "\nThe values are: TO BE IMPLEMENTED";
// Add code here to diplay the complete array at each step
// Hint: for-loop similar to the one in the main() function
for (int count = 0; count < SIZE; count++)
cout << array[count] << " ";
}
bottom--; // Bottom is decremented by 1 since each pass through
// the array adds one more value that is set in order
// Loop repeats until a pass through the array with no swaps occurs
} while (swap != false);
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

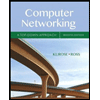
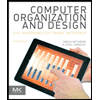
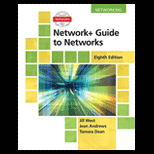
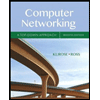
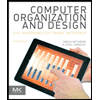
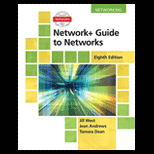
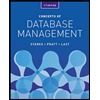
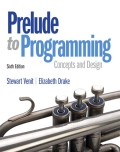
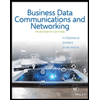