Build the ItemToPurchase class with the following specifications: Attributes item_name (string) item_price (float) item_quantity (int) Default constructor Initializes item's name = "none", item's price = 0, item's quantity = 0 Method print_item_cost() Ex. of print_item_cost() output: Bottled Water 10 @ $1 = $10 (2) In the main section of your code, prompt the user for two items and create two objects of the ItemToPurchase class.
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
(1) Build the ItemToPurchase class with the following specifications:
Attributes
-
- item_name (string)
- item_price (float)
- item_quantity (int)
- Default constructor
- Initializes item's name = "none", item's price = 0, item's quantity = 0
- Method
- print_item_cost()
Ex. of print_item_cost() output:
Bottled Water 10 @ $1 = $10
(2) In the main section of your code, prompt the user for two items and create two objects of the ItemToPurchase class.
Ex:
Item 1
Enter the item name:
Chocolate Chips
Enter the item price:
3
Enter the item quantity:
1
Item 2
Enter the item name:
Bottled Water
Enter the item price:
1
Enter the item quantity:
10
(3) Add the costs of the two items together and output the total cost.
Ex:
TOTAL COST
Chocolate Chips 1 @ $3 = $3
Bottled Water 10 @ $1 = $10
Total: $13
Here is the code that needs to be fixed:
class ItemToPurchase:
def __init__(self, item_name = "none", item_price = 0, item_quantity = 0):
self.item_name = item_name
self.item_price = item_price
self.item_quantity = item_quantity
def print_item_cost(self):
print("{} {} @ ${} = ${}".format(self.item_name, self.item_quantity, self.item_price, (self.item_quantity * self.item_price)))
print("Item 1")
print("Enter the item name:")
name = input()
print("Enter the item price:")
price = float(input())
print("Enter the item quantity:")
quantity = int(input())
i1 = ItemToPurchase(name, price, quantity)
print("Item 2")
print("Enter the item name:")
name = input()
print("Enter the item price:")
price = float(input())
print("Enter the item quantity:")
quantity = int(input())
i2 = ItemToPurchase(name, price, quantity)
totCost = (i1.item_quantity * i1.item_price) + (i2.item_quantity * i2.item_price)
i1.print_item_cost()
i2.print_item_cost()
print()
print("Total: ${}".format(totCost))

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

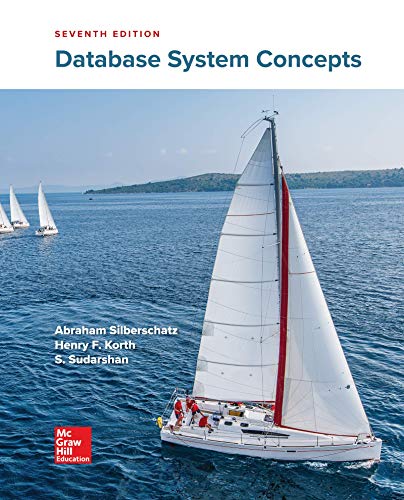
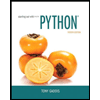
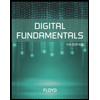
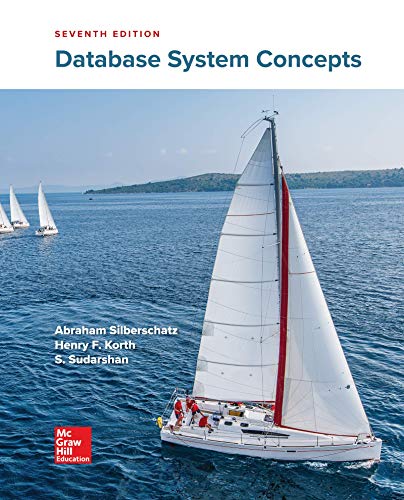
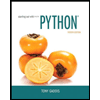
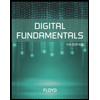
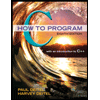
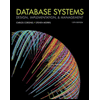
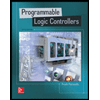