//Below is a partial implemenation of a complete program. //Assume all missing functions are present and working correctly def jumbleWord(word:str) -> str: jumble = "" while len(word) > 0: index = random.randint(0, len(word) - 1) jumble += word[index] word = word[ : index] + word[index + 1: ] return jumble WORDS = ("python", "jumble", "easy", "difficult", "answer", "xylophone") word = random.choice(WORDS) correct = word jumble = jumbleWord(word) displayInstructions() print("The jumble is:", jumble) guess = input("\nYour guess: ") checkGuess(guess, correct) print("That's it! You guessed it!\n") print("Thanks for playing.") Given the program above what is the function header? return jumble jumble = "" while len(word) > 0: index = random.randint(0, len(word) - 1) jumble += word[index] word = word[ : index] + word[index + 1: ] return jumble jumble = jumbleWord(word) def jumbleWord(word:str) -> str: //Below is a partial implemenation of a complete program. //Assume all missing functions are present and working correctly def jumbleWord(word:str) -> str: jumble = "" while len(word) > 0: index = random.randint(0, len(word) - 1) jumble += word[index] word = word[ : index] + word[index + 1: ] return jumble WORDS = ("python", "jumble", "easy", "difficult", "answer", "xylophone") word = random.choice(WORDS) correct = word jumble = jumbleWord(word) displayInstructions() print("The jumble is:", jumble) guess = input("\nYour guess: ") checkGuess(guess, correct) print("That's it! You guessed it!\n") print("Thanks for playing.") Given the program above, what is the function body return jumble def jumbleWord(word:str) -> str: jumble = "" while len(word) > 0: index = random.randint(0, len(word) - 1) jumble += word[index] word = word[ : index] + word[index + 1: ] return jumble jumble = jumbleWord(word) //Below is a partial implemenation of a complete program. //Assume all missing functions are present and working correctly def jumbleWord(word:str) -> str: jumble = "" while len(word) > 0: index = random.randint(0, len(word) - 1) jumble += word[index] word = word[ : index] + word[index + 1: ] return jumble WORDS = ("python", "jumble", "easy", "difficult", "answer", "xylophone") word = random.choice(WORDS) correct = word jumble = jumbleWord(word) displayInstructions() print("The jumble is:", jumble) guess = input("\nYour guess: ") checkGuess(guess, correct) print("That's it! You guessed it!\n") print("Thanks for playing.") Given the program, what is the call to the user defined function? def jumbleWord(word:str) -> str: jumble = "" while len(word) > 0: index = random.randint(0, len(word) - 1) jumble += word[index] word = word[ : index] + word[index + 1: ] return jumble return jumble jumble = jumbleWord(word)
-
//Below is a partial implemenation of a complete
program .
//Assume all missing functions are present and working correctlydef jumbleWord(word:str) -> str:
jumble = ""
while len(word) > 0:
index = random.randint(0, len(word) - 1)
jumble += word[index]
word = word[ : index] + word[index + 1: ]
return jumbleWORDS = ("python", "jumble", "easy", "difficult", "answer", "xylophone")
word = random.choice(WORDS)
correct = word
jumble = jumbleWord(word)
displayInstructions()
print("The jumble is:", jumble)
guess = input("\nYour guess: ")
checkGuess(guess, correct)
print("That's it! You guessed it!\n")
print("Thanks for playing.")Given the program above what is the function header?
return jumble
jumble = ""
while len(word) > 0:
index = random.randint(0, len(word) - 1)
jumble += word[index]
word = word[ : index] + word[index + 1: ]
return jumblejumble = jumbleWord(word)
def jumbleWord(word:str) -> str:
-
//Below is a partial implemenation of a complete program.
//Assume all missing functions are present and working correctlydef jumbleWord(word:str) -> str:
jumble = ""
while len(word) > 0:
index = random.randint(0, len(word) - 1)
jumble += word[index]
word = word[ : index] + word[index + 1: ]
return jumbleWORDS = ("python", "jumble", "easy", "difficult", "answer", "xylophone")
word = random.choice(WORDS)
correct = word
jumble = jumbleWord(word)
displayInstructions()
print("The jumble is:", jumble)
guess = input("\nYour guess: ")
checkGuess(guess, correct)
print("That's it! You guessed it!\n")
print("Thanks for playing.")Given the program above, what is the function body
return jumble
def jumbleWord(word:str) -> str:
jumble = ""
while len(word) > 0:
index = random.randint(0, len(word) - 1)
jumble += word[index]
word = word[ : index] + word[index + 1: ]
return jumblejumble = jumbleWord(word)
//Below is a partial implemenation of a complete program.
//Assume all missing functions are present and working correctlydef jumbleWord(word:str) -> str:
jumble = ""
while len(word) > 0:
index = random.randint(0, len(word) - 1)
jumble += word[index]
word = word[ : index] + word[index + 1: ]
return jumbleWORDS = ("python", "jumble", "easy", "difficult", "answer", "xylophone")
word = random.choice(WORDS)
correct = word
jumble = jumbleWord(word)
displayInstructions()
print("The jumble is:", jumble)
guess = input("\nYour guess: ")
checkGuess(guess, correct)
print("That's it! You guessed it!\n")
print("Thanks for playing.")Given the program, what is the call to the user defined function?
def jumbleWord(word:str) -> str:
jumble = "" while len(word) > 0: index = random.randint(0, len(word) - 1) jumble += word[index] word = word[ : index] + word[index + 1: ] return jumblereturn jumble
jumble = jumbleWord(word)
-

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

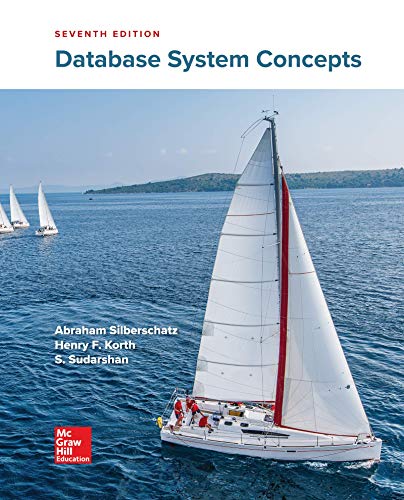
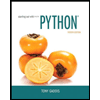
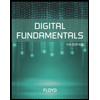
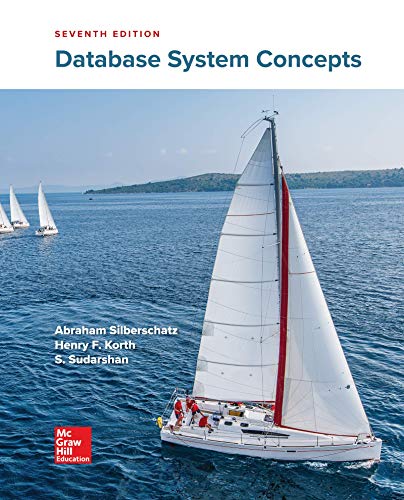
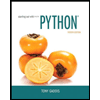
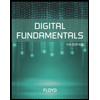
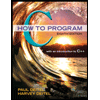
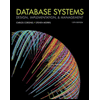
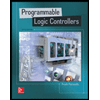