Assume that you are implementing a heap using a fixed size array. 1- Illustrate how the following heap would be constructed (show the content of the array, show in detail only what happens when nodes DOB and 17 are added): The nodes are inserted in the following order (key, value): (9, -4), (3, -6), (11, -4), (13, -7), (14, -2), (12, -8), (33-5), (17, -7), (6, -3). 2- Illustrate what happens to the heap when invoking remove (show the details). 3- Assume that you have an array of integers, write a code that would allow you to sort the array in ascending order (smallest to largest), using only a heap.
Assume that you are implementing a heap using a fixed size array. 1- Illustrate how the following heap would be constructed (show the content of the array, show in detail only what happens when nodes DOB and 17 are added): The nodes are inserted in the following order (key, value): (9, -4), (3, -6), (11, -4), (13, -7), (14, -2), (12, -8), (33-5), (17, -7), (6, -3). 2- Illustrate what happens to the heap when invoking remove (show the details). 3- Assume that you have an array of integers, write a code that would allow you to sort the array in ascending order (smallest to largest), using only a heap.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
![### Heap Implementation Using a Fixed Size Array
This tutorial will guide you through the process of implementing a heap using a fixed size array, illustrating the heap construction and removal processes in detail, and demonstrating how to sort an array using a heap.
#### 1. Constructing the Heap
Illustrate how the following heap would be constructed (show the content of the array, showing in detail only what happens when nodes with keys `9` and `17` are added):
The nodes are inserted in the following order (key, value):
- (9, -4)
- (3, -6)
- (11, -4)
- (13, -7)
- (14, -2)
- (12, -8)
- (33, -5)
- (17, -7)
- (6, -3)
**Heap Construction Steps:**
1. Insert (9, -4)
2. Insert (3, -6)
3. Insert (11, -4)
4. Insert (13, -7)
5. Insert (14, -2)
6. Insert (12, -8)
7. Insert (33, -5)
8. Insert (17, -7)
9. Insert (6, -3)
**Detailed Illustration for Adding Nodes 9 and 17:**
- Initial array with node (9, -4): `[ (9, -4) ]`
- After adding node (17, -7), the array content needs reordering per heap properties. The new array will reflect the heap restructuring needed to insert node (17, -7).
#### 2. Removing an Element from the Heap
Illustrate what happens to the heap when invoking remove (show the details).
**Heap Removal Steps:**
- After removing the root of the heap, restructure the array to maintain heap properties. This involves repositioning nodes to satisfy the heap ordering rules, usually done by promoting the next largest element to the root and adjusting the tree accordingly.
#### 3. Sorting an Array Using a Heap
Assume that you have an array of integers. Write a code that would allow you to sort the array in ascending order (smallest to largest), using only a heap.
**Pseudocode for Heap Sort:**
```python
def heapify(arr, n, i):
largest = i
left = 2 * i + 1
right = 2 * i + 2](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Ff64dcbf8-f12f-4842-986c-dba4c32e364d%2F582456d4-8c28-4726-9d88-ce5d50e22b04%2F654vfz_processed.jpeg&w=3840&q=75)
Transcribed Image Text:### Heap Implementation Using a Fixed Size Array
This tutorial will guide you through the process of implementing a heap using a fixed size array, illustrating the heap construction and removal processes in detail, and demonstrating how to sort an array using a heap.
#### 1. Constructing the Heap
Illustrate how the following heap would be constructed (show the content of the array, showing in detail only what happens when nodes with keys `9` and `17` are added):
The nodes are inserted in the following order (key, value):
- (9, -4)
- (3, -6)
- (11, -4)
- (13, -7)
- (14, -2)
- (12, -8)
- (33, -5)
- (17, -7)
- (6, -3)
**Heap Construction Steps:**
1. Insert (9, -4)
2. Insert (3, -6)
3. Insert (11, -4)
4. Insert (13, -7)
5. Insert (14, -2)
6. Insert (12, -8)
7. Insert (33, -5)
8. Insert (17, -7)
9. Insert (6, -3)
**Detailed Illustration for Adding Nodes 9 and 17:**
- Initial array with node (9, -4): `[ (9, -4) ]`
- After adding node (17, -7), the array content needs reordering per heap properties. The new array will reflect the heap restructuring needed to insert node (17, -7).
#### 2. Removing an Element from the Heap
Illustrate what happens to the heap when invoking remove (show the details).
**Heap Removal Steps:**
- After removing the root of the heap, restructure the array to maintain heap properties. This involves repositioning nodes to satisfy the heap ordering rules, usually done by promoting the next largest element to the root and adjusting the tree accordingly.
#### 3. Sorting an Array Using a Heap
Assume that you have an array of integers. Write a code that would allow you to sort the array in ascending order (smallest to largest), using only a heap.
**Pseudocode for Heap Sort:**
```python
def heapify(arr, n, i):
largest = i
left = 2 * i + 1
right = 2 * i + 2
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
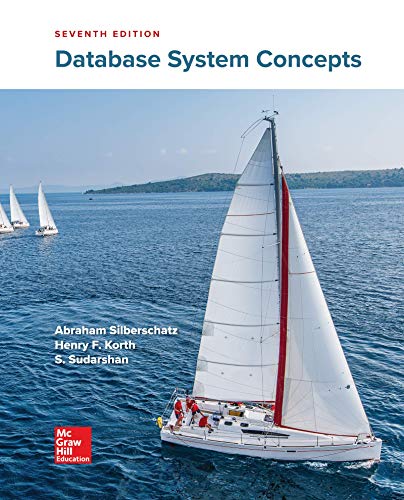
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
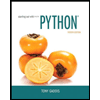
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
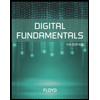
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
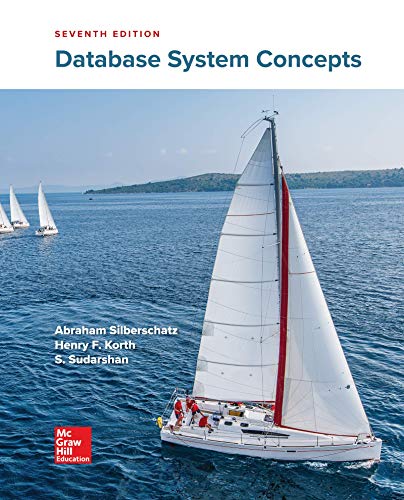
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
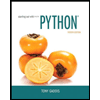
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
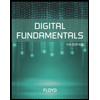
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
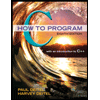
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
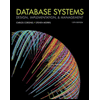
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
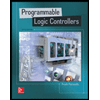
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education