* Array Search */ package int2200.week7; import java.util.Scanner; public class arraySearch { public static void main(String[] args) { } } // create and initializ an arry int[] numbers = {2, -9, 0, 5, 12, -25, 22, 9, 8, 12}; int key; boolean foundit = false; // declare a vraiable and initialize it to false Scanner input = new Scanner (System.in); // Create a Scanner object System.out.println("=>> Array Search program "); System.out.print("Enter a number to search for: "); key=input.nextInt (); // access all elements using for each loop for (int i=0; i< numbers.length; i++) { if (key == numbers [i]) { foundit=true; } } if (foundit == true){ System.out.println("found it! :)"); }else { System.out.println("Sorry } notfound it! :("); System.out.println("==> End of the Search Program "); /* =>> Array Search program Enter a number to search for: 2 found it! :) ==> End of the Search Program */
* Array Search */ package int2200.week7; import java.util.Scanner; public class arraySearch { public static void main(String[] args) { } } // create and initializ an arry int[] numbers = {2, -9, 0, 5, 12, -25, 22, 9, 8, 12}; int key; boolean foundit = false; // declare a vraiable and initialize it to false Scanner input = new Scanner (System.in); // Create a Scanner object System.out.println("=>> Array Search program "); System.out.print("Enter a number to search for: "); key=input.nextInt (); // access all elements using for each loop for (int i=0; i< numbers.length; i++) { if (key == numbers [i]) { foundit=true; } } if (foundit == true){ System.out.println("found it! :)"); }else { System.out.println("Sorry } notfound it! :("); System.out.println("==> End of the Search Program "); /* =>> Array Search program Enter a number to search for: 2 found it! :) ==> End of the Search Program */
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
![```java
/*
* Array Search
*/
package int2200.week7;
import java.util.Scanner;
public class arraySearch {
public static void main(String[ ] args) {
// create and initialize an array
int[ ] numbers = {2, -9, 0, 5, 12, -25, 22, 9, 8, 12};
int key;
boolean foundit = false; // declare a variable and initialize it to false
Scanner input = new Scanner(System.in); // Create a Scanner object
System.out.println("==> Array Search program ");
System.out.print("Enter a number to search for: ");
key = input.nextInt();
// access all elements using for each loop
for (int i = 0; i< numbers.length ; i++) {
if ( key == numbers [i]){
foundit=true;
}
}
if (foundit == true){
System.out.println("found it! :)");
}else {
System.out.println("Sorry notfound it! :(");
}
System.out.println("==> End of the Search Program ");
}
}
/*
==> Array Search program
Enter a number to search for: 2
found it! :)
==> End of the Search Program
*/
```
### Explanation:
This is a Java program designed to perform a simple search within an array of integers. Below is a detailed breakdown of its components:
1. **Package and Imports:**
- The program is contained within the `int2200.week7` package.
- It imports the `java.util.Scanner` class to allow user input.
2. **Main Class (`arraySearch`):**
- The main class is named `arraySearch`.
3. **Main Method (`main`):**
- The `main` method is the entry point of the program.
- An array `numbers` is initialized with a set of integer values: `{2, -9, 0, 5, 12, -25, 22, 9, 8, 12}`.
- A boolean variable `foundit` is declared and initialized to `false` to track if the searched number is found.
4. **User Input:**
- A `Scanner` object named `input` is created to capture user input.
- The program prompts](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F2dedb04f-ec8e-4d86-87d9-6910be748f13%2F002f45d3-d036-4432-886a-826335dd9410%2Fa3rqwt_processed.png&w=3840&q=75)
Transcribed Image Text:```java
/*
* Array Search
*/
package int2200.week7;
import java.util.Scanner;
public class arraySearch {
public static void main(String[ ] args) {
// create and initialize an array
int[ ] numbers = {2, -9, 0, 5, 12, -25, 22, 9, 8, 12};
int key;
boolean foundit = false; // declare a variable and initialize it to false
Scanner input = new Scanner(System.in); // Create a Scanner object
System.out.println("==> Array Search program ");
System.out.print("Enter a number to search for: ");
key = input.nextInt();
// access all elements using for each loop
for (int i = 0; i< numbers.length ; i++) {
if ( key == numbers [i]){
foundit=true;
}
}
if (foundit == true){
System.out.println("found it! :)");
}else {
System.out.println("Sorry notfound it! :(");
}
System.out.println("==> End of the Search Program ");
}
}
/*
==> Array Search program
Enter a number to search for: 2
found it! :)
==> End of the Search Program
*/
```
### Explanation:
This is a Java program designed to perform a simple search within an array of integers. Below is a detailed breakdown of its components:
1. **Package and Imports:**
- The program is contained within the `int2200.week7` package.
- It imports the `java.util.Scanner` class to allow user input.
2. **Main Class (`arraySearch`):**
- The main class is named `arraySearch`.
3. **Main Method (`main`):**
- The `main` method is the entry point of the program.
- An array `numbers` is initialized with a set of integer values: `{2, -9, 0, 5, 12, -25, 22, 9, 8, 12}`.
- A boolean variable `foundit` is declared and initialized to `false` to track if the searched number is found.
4. **User Input:**
- A `Scanner` object named `input` is created to capture user input.
- The program prompts

Transcribed Image Text:### Java Program Exercise: Implementing a Do-While Loop
**Task:**
Modify the following Java program to achieve the specified output using a do-while loop.
**Requirements:**
- **Provide:**
- Detailed documentation and the exact output as described.
**Hint:**
A do-while loop is required for this task.
**Expected Program Output:**
```
==>> Array Search program
Enter a number to search for: 2
found it! :)
Enter any number to continue or number 0 to exit: 5
==>> Array Search program
Enter a number to search for: 6
Sorry not found it! :(
Enter any number to continue or number 0 to exit: 0
==>> End of the Search Program!
```
This task will help you understand the use of the do-while loop in a Java program. Make sure to provide the complete program with necessary comments explaining the logic for full understanding.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
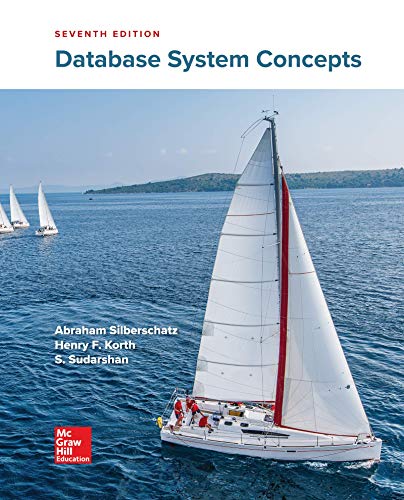
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
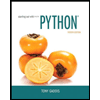
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
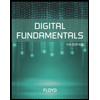
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
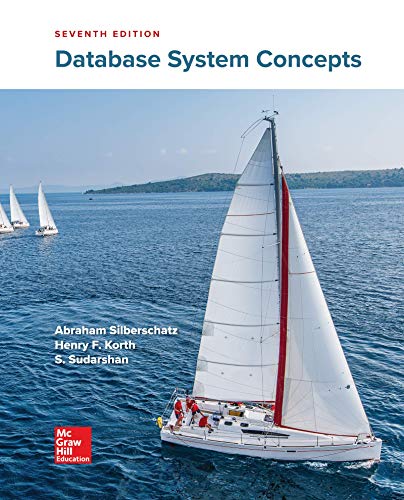
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
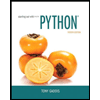
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
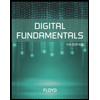
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
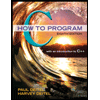
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
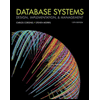
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
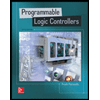
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education