add the following methods listed below to the SortedArrayCollection class shown in screen shot public String toString() // Creates and returns a string that correctly represents the current collection. public T smallest() // Returns null if the collection is empty, otherwise returns the smallest element of the collection. public int greater(T element) // Returns a count of the number of elements e in the collection that are greater then element, that is such that e.compareTo(element) is > 0 public MySortedArrayCollection combine (MySortedArrayCollectionother) // Creates and returns a new SortedArrayCollection object that is a combination of this object and the argument object. public T []toArray() // Returns an array containing all of the elements of the collection. public void clear() // Removes all elements. public boolean equals(Object o) // Takes an Object argument, returning true if it is equal to the current collection and false otherwise public boolean addAll(MySortedArrayCollectionc) // Takes a MySortedArrayCollection argument and adds its contents to the current collection; returns a boolean indicating success or failure. public boolean retainAll(MySortedArrayCollectionc) // Takes a MySortedArrayCollection argument and removes any elements from the current collection that are not in the argument collection; returns a boolean indicating success or failure. public void removeAll(MySortedArrayCollectionc){ // Takes a MySortedArrayCollection argument and removes any elements from the current collection that are also in the argument collection. then write a driver program to test that the methods listed above were implemented corre
add the following methods listed below to the SortedArrayCollection class shown in screen shot
public String toString()
// Creates and returns a string that correctly represents the current collection.
public T smallest()
// Returns null if the collection is empty, otherwise returns the smallest element of the collection.
public int greater(T element)
// Returns a count of the number of elements e in the collection that are greater then element, that is such that e.compareTo(element) is > 0
public MySortedArrayCollection <T> combine (MySortedArrayCollection<T>other)
// Creates and returns a new SortedArrayCollection object that is a combination of this object and the argument object.
public T []toArray()
// Returns an array containing all of the elements of the collection.
public void clear()
// Removes all elements.
public boolean equals(Object o)
// Takes an Object argument, returning true if it is equal to the current collection and false otherwise
public boolean addAll(MySortedArrayCollection<T>c)
// Takes a MySortedArrayCollection argument and adds its contents to the current collection; returns a boolean indicating success or failure.
public boolean retainAll(MySortedArrayCollection<T>c)
// Takes a MySortedArrayCollection argument and removes any elements from the current collection that are not in the argument collection; returns a boolean indicating success or failure.
public void removeAll(MySortedArrayCollection<T>c){
// Takes a MySortedArrayCollection argument and removes any elements from the current collection that are also in the argument collection.
then write a driver program to test that the methods listed above were implemented correctly
![### SortedArrayCollection Class Implementation
The `SortedArrayCollection<T>` class implements the `CollectionInterface<T>`. It is a generic class designed to store elements in a sorted array. Here is a breakdown of its components:
#### Fields
- `protected final int DEFCAP = 100;`
- Default capacity for the array.
- `protected int origCap;`
- Stores the original capacity of the array.
- `protected T[] elements;`
- Array to hold the elements of the collection.
- `protected int numElements = 0;`
- Tracks the number of elements currently in the collection.
- `protected boolean found;`
- A flag to indicate if the target element is found in the array.
- `protected int location;`
- Represents the location of the target if found, or the insertion point if not found.
#### Constructors
- **Default Constructor**
- Initializes the collection with the default capacity `DEFCAP` and sets `origCap`.
- **Parameterized Constructor**
- Accepts an integer `capacity` to define the size of the elements array and sets `origCap`.
#### Methods
- `protected void enlarge()`
- Increases the capacity of the collection:
- **Creates a larger array:** Allocates a new array with additional space equal to `origCap`.
- **Copies contents:** Transfers existing elements to the new larger array.
- **Reassigns the reference:** Updates the `elements` reference to the new larger array.
- `protected void find(T target)`
- Searches for a target element in the array:
- **Initializes `location` and `found`:** Sets `location` to 0 and `found` to false.
- **Checks if empty:** Calls `recFind` if the array is not empty to find or decide the position.
- `protected void recFind(T target, int first, int last)`
- A recursive helper method used by `find`:
- **Determines the `location`:** Calculates the middle of the current range.
- **Performs comparison:**
- Uses `compareTo` for comparison of `target` with current element.
- Updates `found` and `location` based on the comparison result.
- Calls itself recursively to adjust the range when the target is not found.
This class is useful for managing collections that need to maintain a sorted order and](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F1b2c1e36-9974-49eb-8a0c-fe4d3c635f06%2F54eb418a-ec20-4c53-9907-68eb69d86130%2Fhkdsa7b_processed.png&w=3840&q=75)


Trending now
This is a popular solution!
Step by step
Solved in 3 steps

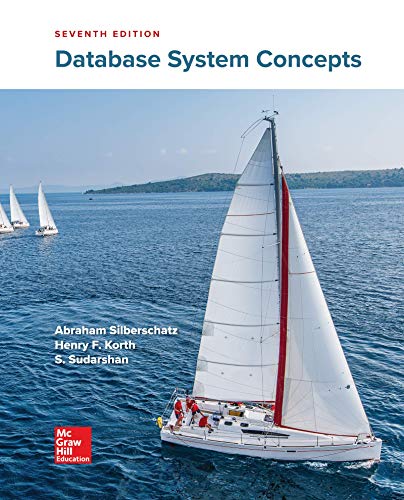
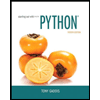
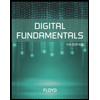
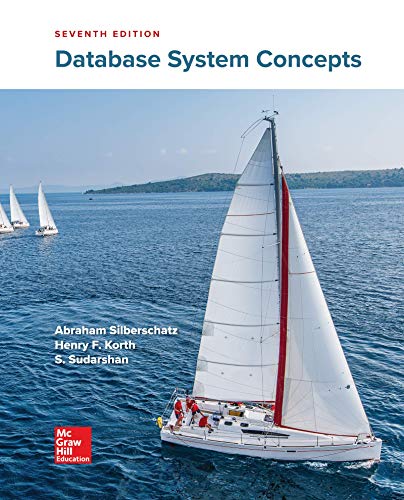
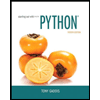
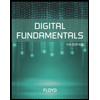
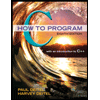
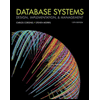
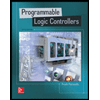