Which of the above choices (A, B, C or D) prints the name of each animal in the animalList object created from a previous question? // Assume that these accessor and mutator methods exist in the Animal class: // public void setAnimalName(String newName) { ... } // public String getAnimalName() { ... } // A for(int i = 0; i < animalList.length(); i++) { Animal tempAnimal = animalList[i]; System.out.println( tempAnimal.getAnimalName()); } // B for(int i = 0; i < animalList.length; i++) { Animal tempAnimal = animalList[i]; System.out.println( tempAnimal.getAnimalName()); } // C for(int i = 0; i < animalList.length; i++) { Animal tempAnimal = animalList[i]; System.out.println( tempAnimal.setAnimalName()); } // D for(int i = 0; i < animalList.length; i++) { Animal tempAnimal = animalList[i]; System.out.println(tempAnimal.animalName); }
Which of the above choices (A, B, C or D) prints the name of each animal in the animalList object created from a previous question?
// Assume that these accessor and mutator methods exist in the Animal class:
// public void setAnimalName(String newName) { ... }
// public String getAnimalName() { ... }
// A
for(int i = 0; i < animalList.length(); i++) {
Animal tempAnimal = animalList[i];
System.out.println(
tempAnimal.getAnimalName());
}
// B
for(int i = 0; i < animalList.length; i++) {
Animal tempAnimal = animalList[i];
System.out.println(
tempAnimal.getAnimalName());
}
// C
for(int i = 0; i < animalList.length; i++) {
Animal tempAnimal = animalList[i];
System.out.println(
tempAnimal.setAnimalName());
}
// D
for(int i = 0; i < animalList.length; i++) {
Animal tempAnimal = animalList[i];
System.out.println(tempAnimal.animalName);
}

Step by step
Solved in 2 steps

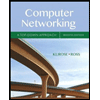
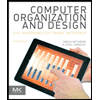
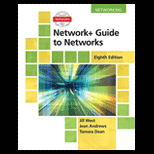
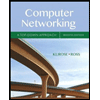
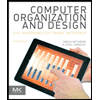
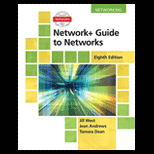
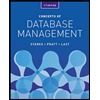
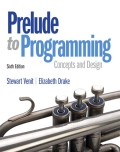
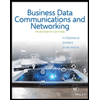