a) Write a function that will print the even elements in each level of the binary search tree. b) Write a function that will count total number of odd nodes of the tree. 10 / \ 6 13 / \ / \ 3 8 11 16 The output of the given tree when the function is applied: 10 6 8 16 Total number of odd nodes is 3. You have to answer both (a) and (b) in same code calling from main function. The base code is given bellow. Modify and write only the main function and the additional function needed to solve the problem in the answer script. BST code: #include using namespace std; struct node { int key; struct node *left, *right; }; // A utility function to search a given node bool search(struct node* root, int key) { // Base Cases: root is null or key is present at root if (root == NULL) return false; else if(root->key == key) return true; // Key is greater than root's key else if (root->key < key) return search(root->right, key); else // Key is smaller than root's key return search(root->left, key); } // A utility function to create a new BST node struct node *newNode(int item) { struct node *temp = new node; temp->key = item; temp->left = temp->right = NULL; return temp; } // A utility function to do inorder traversal of BST void inorder(struct node *root) { if (root != NULL) { inorder(root->left); cout<key<<"\n"; inorder(root->right); } } // A utility function to insert a new node with given key in BST struct node* insert(struct node* node, int key) { /* If the tree is empty, return a new node */ if (node == NULL) return newNode(key); /* Otherwise, recur down the tree */ if (key < node->key) node->left = insert(node->left, key); else if (key > node->key) node->right = insert(node->right, key); /* return the (unchanged) node pointer */ return node; } // Driver Program to test above functions int main() { struct node *root = NULL; root = insert(root, 50); insert(root, 30); insert(root, 20); insert(root, 40); insert(root, 70); insert(root, 60); insert(root, 80); if(search(root,60)==1) cout<<"Node Present\n"; else cout<<"Node not present\n"; // print inoder traversal of the BST inorder(root); return 0; }
- a) Write a function that will print the even elements in each level of the binary search tree.
- b) Write a function that will count total number of odd nodes of the tree.
10
/ \
6 13
/ \ / \
3 8 11 16
The output of the given tree when the function is applied:
10
6
8 16
Total number of odd nodes is 3.
You have to answer both (a) and (b) in same code calling from main function. The base code is given bellow. Modify and write only the main function and the additional function needed to solve the problem in the answer script.
BST code:
#include<iostream>
using namespace std;
struct node
{
int key;
struct node *left, *right;
};
// A utility function to search a given node
bool search(struct node* root, int key)
{
// Base Cases: root is null or key is present at root
if (root == NULL)
return false;
else if(root->key == key)
return true;
// Key is greater than root's key
else if (root->key < key)
return search(root->right, key);
else
// Key is smaller than root's key
return search(root->left, key);
}
// A utility function to create a new BST node
struct node *newNode(int item)
{
struct node *temp = new node;
temp->key = item;
temp->left = temp->right = NULL;
return temp;
}
// A utility function to do inorder traversal of BST
void inorder(struct node *root)
{
if (root != NULL)
{
inorder(root->left);
cout<<root->key<<"\n";
inorder(root->right);
}
}
// A utility function to insert a new node with given key in BST
struct node* insert(struct node* node, int key)
{
/* If the tree is empty, return a new node */
if (node == NULL) return newNode(key);
/* Otherwise, recur down the tree */
if (key < node->key)
node->left = insert(node->left, key);
else if (key > node->key)
node->right = insert(node->right, key);
/* return the (unchanged) node pointer */
return node;
}
// Driver Program to test above functions
int main()
{
struct node *root = NULL;
root = insert(root, 50);
insert(root, 30);
insert(root, 20);
insert(root, 40);
insert(root, 70);
insert(root, 60);
insert(root, 80);
if(search(root,60)==1)
cout<<"Node Present\n";
else
cout<<"Node not present\n";
// print inoder traversal of the BST
inorder(root);
return 0;
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

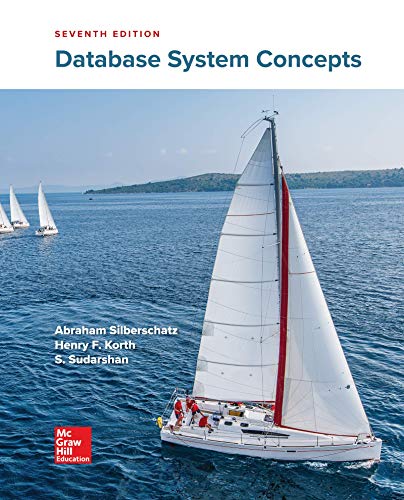
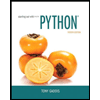
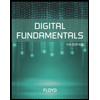
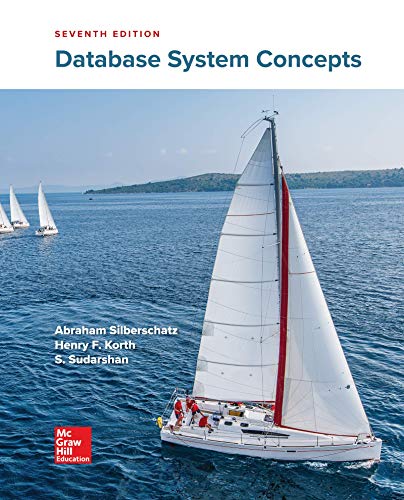
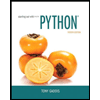
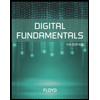
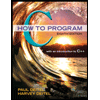
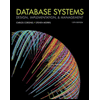
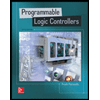