A Flexible Account Class File Account.java contains a definition for a simple bank account class with methods to withdraw, deposit, get the balance and account number, and return a String representation. Note that the constructor for this class creates a random account number. Save this class to your directory and study it to see how it works. Then modify it as follows: 1. Overload the constructor as follows: • public Account (double initBal, String owner, long number) - initializes the balance, owner, and account number as specified public Account (double initBal, String owner) - initializes the balance and owner as specified; randomly generates the account number. public Account (String owner) - initializes the owner as specified; sets the initial balance to 0 and randomly generates the account number. 2. Overload the withdraw method with one that also takes a fee and deducts that fee from the account. File TestAccount.java contains a simple program that exercises these methods. Save it to your directory, study it to see what it does, and use it to test your modified Account class. // Account.java // // A bank account class with methods to deposit to, withdraw from, // change the name on, and get a String representation // of the account. //** public class Account { private double balance; private String name; private long acctNum; //-- //Constructor /- public Account (double initBal, String owner, long number) { balance = initBal; initializes balance, owner, and account number name = owner; acctNum = number; //-- // Checks to see if balance is sufficient for withdrawal. // If so, decrements balance by amount; if not, prints message. //-- public void withdraw(double amount) { if (balance >= amount) balance -= amount; else System.out.println ("Insufficient funds"); // Adds deposit amount to balance. //
A Flexible Account Class File Account.java contains a definition for a simple bank account class with methods to withdraw, deposit, get the balance and account number, and return a String representation. Note that the constructor for this class creates a random account number. Save this class to your directory and study it to see how it works. Then modify it as follows: 1. Overload the constructor as follows: • public Account (double initBal, String owner, long number) - initializes the balance, owner, and account number as specified public Account (double initBal, String owner) - initializes the balance and owner as specified; randomly generates the account number. public Account (String owner) - initializes the owner as specified; sets the initial balance to 0 and randomly generates the account number. 2. Overload the withdraw method with one that also takes a fee and deducts that fee from the account. File TestAccount.java contains a simple program that exercises these methods. Save it to your directory, study it to see what it does, and use it to test your modified Account class. // Account.java // // A bank account class with methods to deposit to, withdraw from, // change the name on, and get a String representation // of the account. //** public class Account { private double balance; private String name; private long acctNum; //-- //Constructor /- public Account (double initBal, String owner, long number) { balance = initBal; initializes balance, owner, and account number name = owner; acctNum = number; //-- // Checks to see if balance is sufficient for withdrawal. // If so, decrements balance by amount; if not, prints message. //-- public void withdraw(double amount) { if (balance >= amount) balance -= amount; else System.out.println ("Insufficient funds"); // Adds deposit amount to balance. //
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Couldn't fit the whole problem into 1

Transcribed Image Text:A Flexible Account Class
File Account.java contains a definition for a simple bank account class with methods to withdraw, deposit, get the balance
and account number, and return a String representation. Note that the constructor for this class creates a random account
number. Save this class to your directory and study it to see how it works. Then modify it as follows:
1. Overload the constructor as follows:
• public Account (double initBal, String owner, long number) - initializes the balance, owner, and account
number as specified
public Account (double initBal, String owner) - initializes the balance and owner as specified; randomly
generates the account number.
• public Account (String owner) - initializes the owner as specified; sets the initial balance to 0 and randomly
generates the account number.
2. Overload the withdraw method with one that also takes a fee and deducts that fee from the account.
File TestAccount.java contains a simple program that exercises these methods. Save it to your directory, study it to see what
it does, and use it to test your modified Account class.
// Account.java
//
// A bank account class with methods to deposit to, withdraw from,
// change the name on, and get a String representation
// of the account.
//*****************:
*******
public class Account
{
private double balance;
private String name;
private long acctNum;
//-
//Constructor
//----
public Account (double initBal, String owner, long number)
{
initializes balance,
owner, and account number
balance = initBal;
= owner;
acctNum = number;
name
}
//-
// Checks to see if balance is sufficient for withdrawal.
// If so, decrements balance by amount; if not, prints message.
//--
public void withdraw (double amount)
{
if (balance >= amount)
balance -=
amount;
else
System.out.println("Insufficient funds") ;
//-
// Adds deposit amount to balance.
//--
![public void deposit (double amount)
{
balance += amount;
}
//-
// Returns balance.
//--
public double getBalance ()
{
return balance;
}
//-
// Returns a string containing the name, account number, and balance.
//--
public String toString ( )
{
return "Name:"
name
"\nAccount Number: "
+ acctNum +
"\nBalance:
+ balance;
}
}
//*********
******
*****
*************
// TestAccount.java
//
// A simple driver to test the overloaded methods of
// the Account class.
//***************************
***********
import java.util.Scanner;
public class TestAccount
public static void main(String[] args)
{
String name;
double balance;
long acctNum;
Account acct;
Scanner scan
= new Scanner(System.in);
System.out.println("Enter account holder's first name");
= scan.next ();
acct = new Account (name);
System.out.println("Account for "
System.out.println(acct);
name
+ ": ");
+
name
System.out.println("\nEnter initial balance");
balance = scan.nextDouble ();
acct = new Account (balance,name);
System.out.println("Account for
System.out.println(acct);
+ ":");
%3D
+
name
110
Chapter 7: Object-Oriented Design
System.out.println("\nEnter account number");
acctNum =
scan.nextLong();
acct = new Account (balance,name,acctNum);
System.out.println( "Account for
System.out.println(acct);
name
":");
System.out.print("\nDepositing 100 into account, balance is now ");
acct.deposit(100);
System.out.println(acct.getBalance ());
System.out.print ("\nWithdrawing $25, balance is now ");
acct.withdraw (25);
System.out.println(acct.getBalance ());
System.out.print("\nWithdrawing $25 with $2 fee, balance is now ");
acct.withdraw(25,2);
System.out.println (acct.getBalance ());
System.out.println(" \nBye!");
}
}](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F6a453cf0-4fa7-465e-ab7f-471dd1e543ba%2F7b6b2229-ad75-4f1d-a298-3f715f6b50c5%2F8yqtyz_processed.jpeg&w=3840&q=75)
Transcribed Image Text:public void deposit (double amount)
{
balance += amount;
}
//-
// Returns balance.
//--
public double getBalance ()
{
return balance;
}
//-
// Returns a string containing the name, account number, and balance.
//--
public String toString ( )
{
return "Name:"
name
"\nAccount Number: "
+ acctNum +
"\nBalance:
+ balance;
}
}
//*********
******
*****
*************
// TestAccount.java
//
// A simple driver to test the overloaded methods of
// the Account class.
//***************************
***********
import java.util.Scanner;
public class TestAccount
public static void main(String[] args)
{
String name;
double balance;
long acctNum;
Account acct;
Scanner scan
= new Scanner(System.in);
System.out.println("Enter account holder's first name");
= scan.next ();
acct = new Account (name);
System.out.println("Account for "
System.out.println(acct);
name
+ ": ");
+
name
System.out.println("\nEnter initial balance");
balance = scan.nextDouble ();
acct = new Account (balance,name);
System.out.println("Account for
System.out.println(acct);
+ ":");
%3D
+
name
110
Chapter 7: Object-Oriented Design
System.out.println("\nEnter account number");
acctNum =
scan.nextLong();
acct = new Account (balance,name,acctNum);
System.out.println( "Account for
System.out.println(acct);
name
":");
System.out.print("\nDepositing 100 into account, balance is now ");
acct.deposit(100);
System.out.println(acct.getBalance ());
System.out.print ("\nWithdrawing $25, balance is now ");
acct.withdraw (25);
System.out.println(acct.getBalance ());
System.out.print("\nWithdrawing $25 with $2 fee, balance is now ");
acct.withdraw(25,2);
System.out.println (acct.getBalance ());
System.out.println(" \nBye!");
}
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 5 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
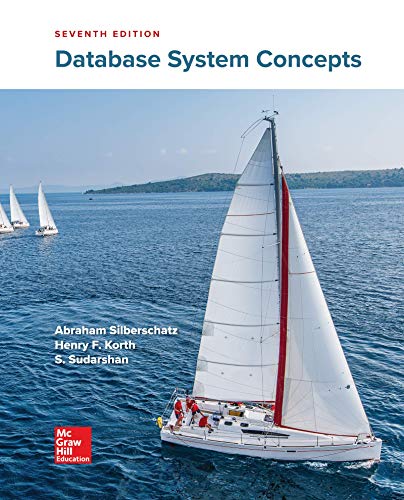
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
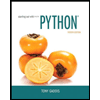
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
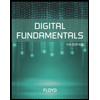
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
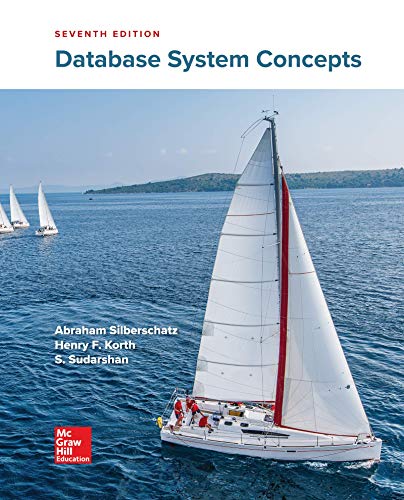
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
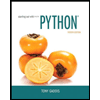
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
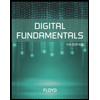
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
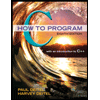
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
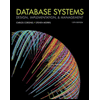
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
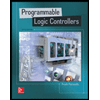
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education