The vector of constant terms b contains all ones, except that the first and last elements are zero. You can use td to find that x1= −0.10557. The following code format should help: def td(l, m, u, b): '''Solve a linear system Ax = b where A is tridiagonal
IN PYTHON I want a code in python and no need to post work on paper Iwant a screenshot of the code
A tridiagonal matrix is one where the only nonzero elements are the ones on the main diagonal and the ones immediately above and below it.Write a function that solves a linear system whose coefficient matrix is tridiag- onal. In this case, Gauss elimination can be made much more efficient because most elements are already zero and don't need to be modified or added. As an example, consider a linear system Ax = b with 100,000 unknowns and the same number of equations. The coefficient matrix A is tridiagonal, with all elements on the main diagonal equal to 3 and all elements on the diagonals above and below it equal to 1. The
The following code format should help:
def td(l, m, u, b):
'''Solve a linear system Ax = b where A is tridiagonal
Inputs: l, lower diagonal of A, n-1 vector
m, main diagonal of A, n vector
u, upper diagonal of A, n-1 vector
b, right-hand constant in each equation, n vector
Output: x, vector of unknowns, n vector
Example: if A = 2 -2 0 0
-1 4 -2 0
0 -1 6 -2
0 0 -1 8
and b = [24; 12; -98; 55],
then l = [-1; -1; -1], m = [2; 4; 6; 8], u = [-2; -2; -2],
and x = [10; -2; -15; 5]'''
THANK YOU!

-
Create the function
td
which takes the argumentsl
,m
,u
, andb
. These represent the lower diagonal, main diagonal, upper diagonal, and right-hand side of the linear systemAx = b
, respectively. -
Initialize the variable
n
to be the length of the main diagonalm
. Also, create a listx
of zeros to hold the solution to the linear system. -
Perform forward elimination to transform the coefficient matrix
A
into upper triangular form. This involves dividing each row ofA
by its pivot element and subtracting it from the row below it. In the tridiagonal case, this involves only two nonzero elements per row. Specifically, fori
from1
ton-1
, compute the factorl[i-1] / m[i-1]
, subtractfactor * u[i-1]
fromm[i]
, and subtractfactor * b[i-1]
fromb[i]
. -
Perform backward substitution to solve for the unknowns
x
. This involves starting at the last row and computing eachx[i]
based on the values ofx[i+1]
and the coefficients in the corresponding row ofA
. Specifically, setx[n-1] = b[n-1] / m[n-1]
, and fori
fromn-2
down to0
, computex[i]
as(b[i] - u[i]*x[i+1]) / m[i]
. -
Return the solution vector
x
. -
Create an example usage where
l
,m
,u
, andb
correspond to the given tridiagonal matrixA
and constant vectorb
. Call thetd
function with these inputs and print the resulting solution vectorx
.
Step by step
Solved in 4 steps with 2 images

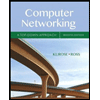
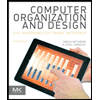
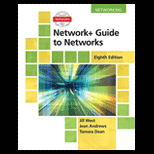
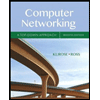
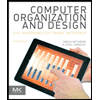
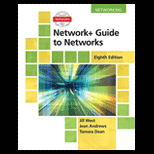
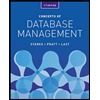
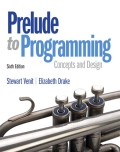
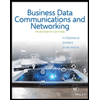