A C++ assignment Implement the GradedActivity class. Copying from the pdfs is fine. Create a new class Assignment which is derived from GradedActivity. It should have three private member ints for 3 different parts of an assignment score: functionality (max 50 points), efficiency (max 25 points), and style (max 25 points). Create member function set() in Assignment which takes three parameter ints and sets the member variables. It should also set its score member, which is inherited from GradedActivity, using the setScore() function, to functionality + efficiency + style. Signature: void Assignment::set(int, int, int) Create a main program which instantiates an Assignment, asks the user for its functionality, efficiency, and style scores, and prints out the score and letter grade for the assignment.
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
A C++ assignment
- Implement the GradedActivity class. Copying from the pdfs is fine.
- Create a new class Assignment which is derived from GradedActivity. It should have three private member ints for 3 different parts of an assignment score: functionality (max 50 points), efficiency (max 25 points), and style (max 25 points).
- Create member function set() in Assignment which takes three parameter ints and sets the member variables. It should also set its score member, which is inherited from GradedActivity, using the setScore() function, to functionality + efficiency + style. Signature:
void Assignment::set(int, int, int)
- Create a main program which instantiates an Assignment, asks the user for its functionality, efficiency, and style scores, and prints out the score and letter grade for the assignment.
___________________________________________________
Examples from the pdfs:
numQuestions = q;
numMissed = m;
numToPass = p;
pointsEach = 100.0 / numQuestions;
numericScore = 100.0 - (numMissed * pointsEach);
if ((numQuestions - numMissed) >= numToPass) return 'P';
else return 'F';}
___________________________________________________
{ numQuestions = 0; numMissed = 0; numToPass = 0; }
void set(int, int, int); char getLetterGrade() const;};
___________________________________________________
{ score = 0.0; } GradedActivity(double s)
{ score = s; }
void setScore(double s)
{ score = s; } double getScore()
{ return score; } char getLetterGrade() const;quiz.set(10,2,8);
cout << "The score on quiz is: ";
cout << quiz.getScore() << endl;
cout << "The letter grade is: ";
cout << quiz.getLetterGrade() << endl;
____________________________________________________________________________________________________________________________________________________________________________________________________________
Polymorphism Chapter:
{
private:
{ numQuestions = 0; numMissed = 0; numToPass = 0; }
void set(int, int, int); char getLetterGrade() const;
int main()
{ GradedActivity HW1; PassFailExam quiz; GradedActivity *gaPtr;HW1.setScore(80); quiz.set(10,2,8);
gaPtr = &HW1;
cout << "HW1 grade: " << gaPtr->getLetterGrade() << endl;
gaPtr = &quiz;cout << "quiz grade: " << gaPtr->getLetterGrade() << endl; }
___________________________________________________
{ score = 0.0; } GradedActivity(double s)
{ score = s; }
void setScore(double s)
{ score = s; } double getScore()
int main()
{ GradedActivity HW1; PassFailExam quiz; GradedActivity *gaPtr;HW1.setScore(80); quiz.set(10,2,8);
___________________________________________________
int main()
{ GradedActivity HW1;HW1.setScore(80);
quiz.set(10,2,8);
gaPtr = &HW1;
cout << "HW1 grade: " << gaPtr->getLetterGrade() << endl;
gaPtr = &quiz;cout << "quiz grade: " << gaPtr->getLetterGrade() << endl;
___________________________________________________
{ score = 0.0; } GradedActivity(double s)
{ score = s; }
void setScore(double s)
{ score = s; } double getScore()
{ return score; }
virtual char getLetterGrade() const;
};

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 4 images

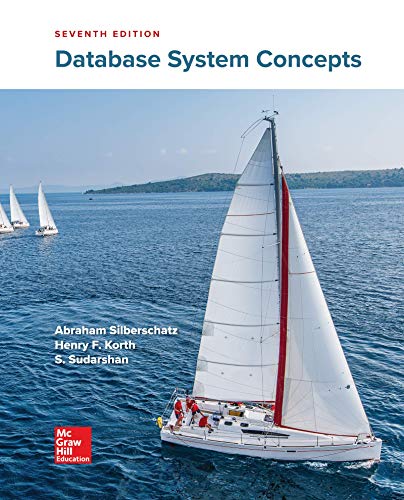
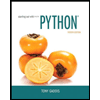
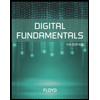
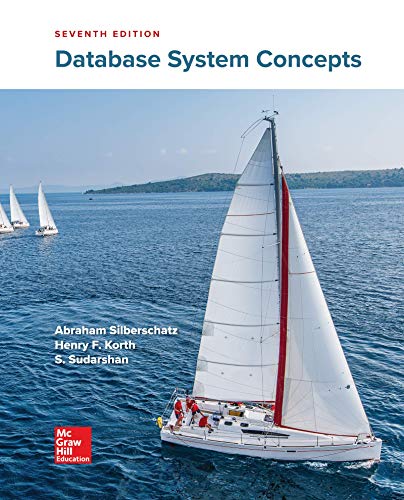
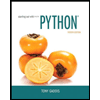
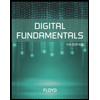
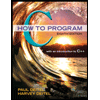
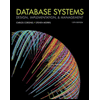
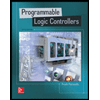