1. Write a custom unittest Class that tests a giving si module which has a SimpleInteger class with the following functions: add(a, b) -> returns a + b subtract(a, b) -> returns a - b multiply (a, b) -> returns a b isequal (a, b) > returns True if a == b otherwise False isgreater than (a, b) -> returns True if a > b otherwise False Note: All functions return a None if either a or b are not integers.
1. Write a custom unittest Class that tests a giving si module which has a SimpleInteger class with the following functions: add(a, b) -> returns a + b subtract(a, b) -> returns a - b multiply (a, b) -> returns a b isequal (a, b) > returns True if a == b otherwise False isgreater than (a, b) -> returns True if a > b otherwise False Note: All functions return a None if either a or b are not integers.
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question

Transcribed Image Text:### Creating a Custom Unittest Class for a SimpleInteger Module
This guide details how to design a unittest class to test a `SimpleInteger` class within an `si` module. The module includes several arithmetic functions. Here's a breakdown of each step and function involved:
#### Functions in the SimpleInteger Class:
1. **add(a, b)**: Returns the sum of `a` and `b`.
2. **subtract(a, b)**: Returns the difference between `a` and `b`.
3. **multiply(a, b)**: Returns the product of `a` and `b`.
4. **isequal(a, b)**: Returns `True` if `a` is equal to `b`, otherwise `False`.
5. **isgreaterthan(a, b)**: Returns `True` if `a` is greater than `b`, otherwise `False`.
**Note:** All functions return `None` if either `a` or `b` is not an integer.
#### Steps to Create a Test Module:
1. **Create a File:**
- Name your file `test.py`.
2. **Import Required Modules:**
- Include built-in modules `unittest` and `io`.
- Import the `si` module.
3. **Define a Testing Class:**
- Create a class named `Test01_add_valid_data` inheriting from `unittest.TestCase`.
- **Member Function for Testing:**
1. Define a function `test_list_int` to test integer addition.
2. Inside this function:
- Instantiate the `SimpleInteger` class.
- Call the `add` function with integers, e.g., `1` and `2`.
- Use `unittest`'s `self.assertEqual` to compare the expected output (e.g., `3`) with the actual output.
4. **Documentation:**
- Use docstrings to describe what each unit of the test is checking and the expected result for a given input.
This guide assists you in setting up robust unittests to ensure the correctness of arithmetic functions within a `SimpleInteger` class, ensuring reliable output for integer inputs.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 1 images

Recommended textbooks for you
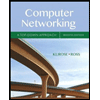
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
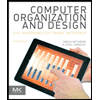
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
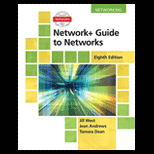
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
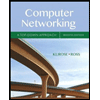
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
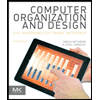
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
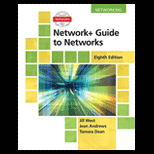
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
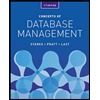
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
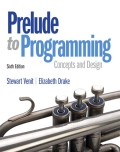
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
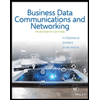
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY