Write program in C++ using inhertiance and polymorphism. A car dealership wants you to keep track of their sold and leased vehicles. You have realized that all vehicles have a make, model and vehicle identification number so you are going to factor those attributes out and put them in the base class as private attributes. Additionally you realize that you need to create two derived classes: one that keeps track of vehicles that are sold and the other that keeps track of ones that are leased. Vehicles that are sold have a sale date and sale amount. Vehicles that are leased have a monthly lease payment and terms of the lease (number of years). For all classes, create a proper overloaded constructor and display method that properly displays the class’s attributes. For your display method make sure you are using proper run-time polymorphism techniques so that your code calls the correct display methods. Complete main.cpp and follow comments for instruction Given code of .cpp and .h classes attached below
Write program in C++ using inhertiance and polymorphism.
A car dealership wants you to keep track of their sold and leased vehicles. You have realized that all vehicles have a make, model and vehicle identification number so you are going to factor those attributes out and put them in the base class as private attributes. Additionally you realize that you need to create two derived classes: one that keeps track of vehicles that are sold and the other that keeps track of ones that are leased.
Vehicles that are sold have a sale date and sale amount. Vehicles that are leased have a monthly lease payment and terms of the lease (number of years).
For all classes, create a proper overloaded constructor and display method that properly displays the class’s attributes. For your display method make sure you are using proper run-time polymorphism techniques so that your code calls the correct display methods. Complete main.cpp and follow comments for instruction
Given code of .cpp and .h classes attached below



Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 1 images

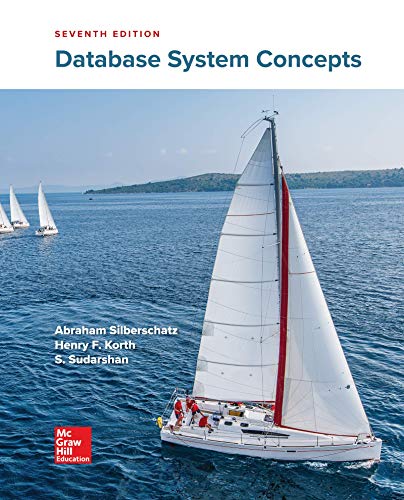
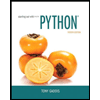
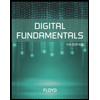
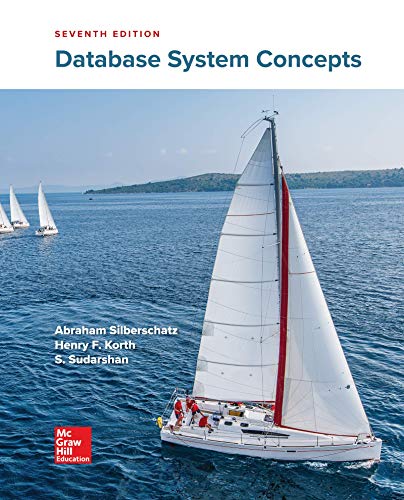
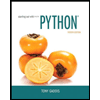
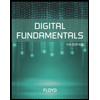
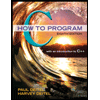
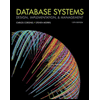
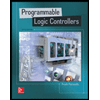