Write a C++ program which creates a Class named as Person which represents a person by its Id (it must be unique), Name, Age, Address and Phone no. This class should have a parameterized constructor and destructor, the getter/setter functions and a showoutput () member function that prints the information of a Person object. PART A Create a Class named as Student that inherits publicly from class Person. A Student object is distinguished by the same attributes as a Person object plus his Course name, Room no (which is allocated to him) and GPA. This class should also have parameterized constructor and default destructor, setter/getter functions and a showoutput () member function. Your input should be similar to the following: *-*-*-**-*-*-*-*-*-*-*-*-* Enter Student's Information *-*-*-**-*-*-*-*-*-*-*-*-* Enter the Roll No: 1 Enter the Name of the Person: khuram Enter the Age of the Person: 23 Enter the Address of the Person: lahore Enter the Phone No of the Person: 234566 Enter the Course Discipline of the Person [CS/IT]: CS Enter the Room No of the Person: 2 Enter the GPA of the Person: 3.5 Your output should be similar to the following: *-*-*-*-*-*-*-*-*-* Student's Data *-*-*-*-*-*-*-*-*-* Student Id: 1 Student Name: khuram Student Age: 23 Student Address: lahore Student Phone Number: 234566 Student Course Discipline [CS/IT]: CS Student's Room No: 2 Student's GPA: 3.5
Write a C++ program which creates a Class named as Person which represents a person by its Id (it must be unique),
Name,
Age, Address and Phone no.
This class should have a parameterized constructor and destructor, the getter/setter functions and a showoutput () member function that prints the information of a Person object.
PART A
Create a Class named as Student that inherits publicly from class Person. A Student object is
distinguished by the same attributes as a Person object plus his
Course name,
Room no (which is allocated to him) and GPA.
This class should also have parameterized constructor and default destructor, setter/getter functions and a showoutput () member function.
Your input should be similar to the following:
*-*-*-**-*-*-*-*-*-*-*-*-*
Enter Student's Information
*-*-*-**-*-*-*-*-*-*-*-*-*
Enter the Roll No: 1
Enter the Name of the Person: khuram
Enter the Age of the Person: 23
Enter the Address of the Person: lahore
Enter the Phone No of the Person: 234566
Enter the Course Discipline of the Person [CS/IT]: CS
Enter the Room No of the Person: 2
Enter the GPA of the Person: 3.5
Your output should be similar to the following:
*-*-*-*-*-*-*-*-*-*
Student's Data
*-*-*-*-*-*-*-*-*-*
Student Id: 1
Student Name: khuram
Student Age: 23
Student Address: lahore
Student Phone Number: 234566
Student Course Discipline [CS/IT]: CS
Student's Room No: 2
Student's GPA: 3.5
PART B
Similarly, also develop a Class named as Employee that inherits publicly from class Person. An Employee object is distinguished by the same attributes as a Person object plus his
Department name,
Gross salary,
Tax percentage and Net salary.
This class should also have a parameterized constructor, default destructor, setter/getter functions and a showoutput () member function.
Your program should ask the user about the all the information required for Employee.
Your input should be similar to the following:
*-*-*-**-*-*-*-*-*-*-*-*-*
Enter Employee's Information
*-*-*-**-*-*-*-*-*-*-*-*-*
Enter the Employee Id: 1
Enter the Name of the Person: kamran
Enter the Age of the Person: 32
Enter the Address of the Person: karachi
Enter the Phone No of the Person: 234567
Enter the Department of the Person: CS Enter the Gross Salary of the Person: 30000
Enter the Tax Percentage of the Person: 15
Your output should be similar to the following:
*-*-*-*-*-*-*-*-*-*
Employee's Data
*-*-*-*-*-*-*-*-*-*
Employee Id: 1 |
|
Employee Name: Employee Age: 32 |
kamran |
Employee Address: |
karachi |
Employee Phone Number: |
234567 |
Employee Department: |
CS |
Employee's Gross Salary: |
30000 with 15 % of Tax |
Employee's Net Salary: |
25500 |

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

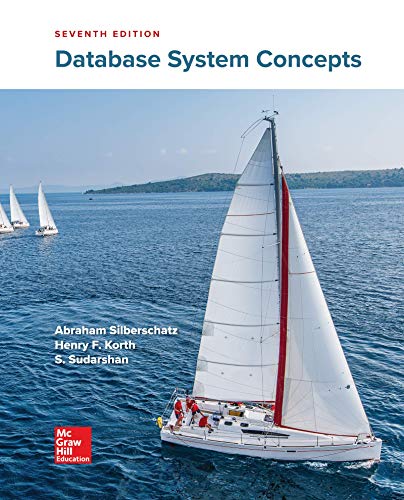
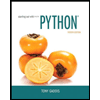
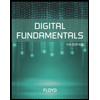
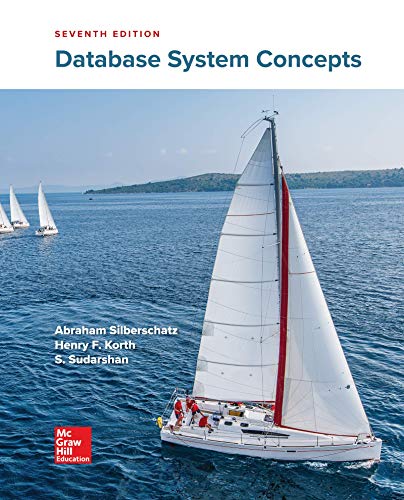
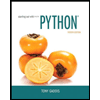
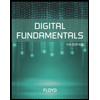
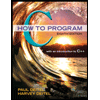
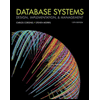
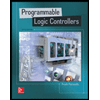