9.10 LAB: Parsing dates Complete main() to read dates from input, one date per line. Each date's format must be as follows: March 1, 1990. Any date not following that format is incorrect and should be ignored. Use the substr() function to parse the string and extract the date. The input ends with -1 on a line alone. Output each correct date as: 3-1-1990. Ex: If the input is: March 1, 1990 April 2 1995 7/15/20 December 13, 2003 -1 then the output is: 3-1-1990 12-13-2003 Use the provided DateParser() function to convert a month string to an integer. If the month string is valid, an integer in the range 1 to 12 inclusive is returned, otherwise 0 is returned. Ex: DateParser("February") returns 2 and DateParser("7/15/20") returns 0. #include #include using namespace std; int DateParser(string month) { int monthInt = 0; if (month == "January") monthInt = 1; else if (month == "February") monthInt = 2; else if (month == "March") monthInt = 3; else if (month == "April") monthInt = 4; else if (month == "May") monthInt = 5; else if (month == "June") monthInt = 6; else if (month == "July") monthInt = 7; else if (month == "August") monthInt = 8; else if (month == "September") monthInt = 9; else if (month == "October") monthInt = 10; else if (month == "November") monthInt = 11; else if (month == "December") monthInt = 12; return monthInt; } int main () { // TODO: Read dates from input, parse the dates to find the ones // in the correct format, and output in m-d-yyyy format }
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
9.10 LAB: Parsing dates
Complete main() to read dates from input, one date per line. Each date's format must be as follows: March 1, 1990. Any date not following that format is incorrect and should be ignored. Use the substr() function to parse the string and extract the date. The input ends with -1 on a line alone. Output each correct date as: 3-1-1990.
Ex: If the input is:
March 1, 1990
April 2 1995
7/15/20
December 13, 2003
-1
then the output is:
3-1-1990
12-13-2003
Use the provided DateParser() function to convert a month string to an integer. If the month string is valid, an integer in the range 1 to 12 inclusive is returned, otherwise 0 is returned. Ex: DateParser("February") returns 2 and DateParser("7/15/20") returns 0.
#include <iostream>
#include <string>
using namespace std;
int DateParser(string month) {
int monthInt = 0;
if (month == "January")
monthInt = 1;
else if (month == "February")
monthInt = 2;
else if (month == "March")
monthInt = 3;
else if (month == "April")
monthInt = 4;
else if (month == "May")
monthInt = 5;
else if (month == "June")
monthInt = 6;
else if (month == "July")
monthInt = 7;
else if (month == "August")
monthInt = 8;
else if (month == "September")
monthInt = 9;
else if (month == "October")
monthInt = 10;
else if (month == "November")
monthInt = 11;
else if (month == "December")
monthInt = 12;
return monthInt;
}
int main () {
// TODO: Read dates from input, parse the dates to find the ones
// in the correct format, and output in m-d-yyyy format
}

Trending now
This is a popular solution!
Step by step
Solved in 6 steps with 1 images

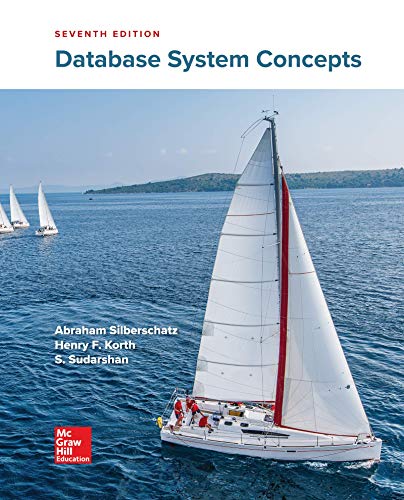
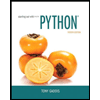
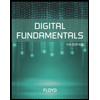
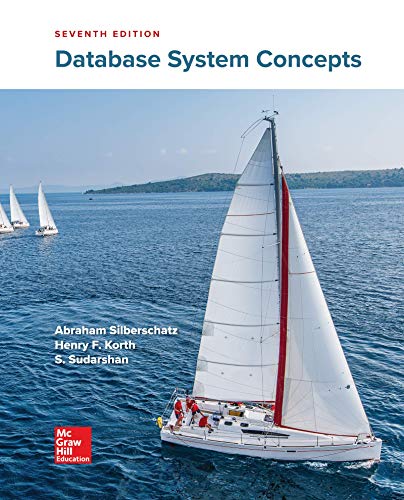
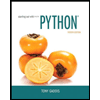
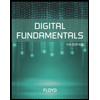
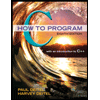
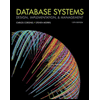
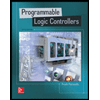