Write a program that takes an integer list as input and sorts the list into descending order using selection sort. The program should use nested loops and output the list after each iteration of the outer loop, thus outputting the list N-1 times (where N is the size of the list).
2.15 LAB: Descending selection sort with output during execution
Write a program that takes an integer list as input and sorts the list into descending order using selection sort. The program should use nested loops and output the list after each iteration of the outer loop, thus outputting the list N-1 times (where N is the size of the list).
Important Coding Guidelines:
- Use comments, and whitespaces around operators and assignments.
- Use line breaks and indent your code.
- Use naming conventions for variables, functions, methods, and more. This makes it easier to understand the code.
- Write simple code and do not over complicate the logic. Code exhibits simplicity when it’s well organized, logically minimal, and easily readable.
Ex: If the input is:
20 10 30 40the output is:
[40, 10, 30, 20] [40, 30, 10, 20] [40, 30, 20, 10]Ex: If the input is:
7 8 3the output is:
[8, 7, 3] [8, 7, 3]Note: Use print(numbers) to output the list numbers and achieve the format shown in the example.
numbers = []
user_input = input()
tokens = user_input.split()
for token in tokens:
numbers.append(int(tokens))
#Convert the string input into an array of integers.
for j in range(len(numbers)-1):
# Find index of smallest remaining element
index_largest = j
for j in range(j+1, len(numbers)):
if numbers[j] < numbers[index_largest]:
index_largest = j
# Swap numbers[i] and numbers[index_smallest]
temp = numbers[j]
numbers[j] = numbers[index_largest]
numbers[index_largest] = temp
print(numbers)
![SCTU Class Homepage.
zy Section 2.15 - CS 233T: Fun X
= zyBooks
✰ learn.zybooks.com/zybook/CS233-2204A-01-2204A/chapter/2/section/15
My library > CS 233T: Fundamentals of Data Structures home >
2.15: LAB: Descending selection sort with output during execution
LAB
ACTIVITY
Note: Use print (numbers) to output the list numbers and achieve the format shown in the example.
422794.2543518.qx3zqy7
6
7
1 numbers = []
2 user_input = input()
3 tokens = user_input.split()
4 for token in tokens:
5
8
9
10
11
Section 2.1 - CS 233T: Fund X
12
13
14
15
- 16 17 18
2.15.1: LAB: Descending selection sort with output during execution
Thank you. Your reservation X
numbers.append(int (tokens))
#Convert the string input into an array of integers.
for j in range (len (numbers)-1):
# Find index of smallest remaining element
index_largest = j
for j in range(j+1, len(numbers)):
if numbers [j] < numbers [index_largest]:
index_largest = j
Develop mode
# Swap numbers[i] and numbers [index_smallest]
temp = numbers[j]
numbers [j] = numbers [index_largest]
Submit mode
Enter program input (optional)
20 10 30 40
main.py
Print - Your Confirmed Rese X
b Answered: CHALLENGE AC
zyBooks catalog
0/10
Load default template...
Run your program as often as you'd like, before submitting for grading. Below, type any needed
input values in the first box, then click Run program and observe the program's output in the
second box.
le
+
? Help/FAQ
Stacey Queen
X
:
3:46 PM
8/29/2022](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F788fc729-a951-45c4-9909-47eea7bbf82f%2Fbabe527b-0f0e-4bd2-9ba2-6f78b61554e4%2Fppcn7pi_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

hi this helps me wiht the solution but how can I make it indented (image for reference)
Thanks
![1:Compare output
Output is nearly correct, but whitespace differs. See highlights below. Special character legend
Input
Your output
Expected output
20 10 30 40
[40,10,30,20] [40,30,10,20] [40,30,20,10]
[40, 10, 30, 2014
[40, 30, 10, 2014
[40, 30, 20, 10]](https://content.bartleby.com/qna-images/question/a090ff11-7282-4dfe-a94b-07141c4dc3b0/867477ae-de7a-41d1-96cb-aec6ffe3856e/99j924a_thumbnail.png)
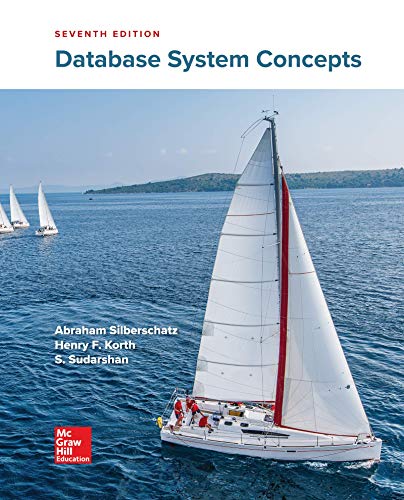
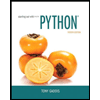
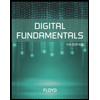
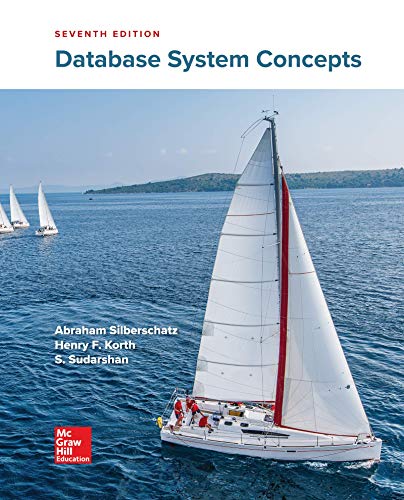
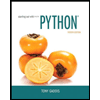
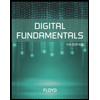
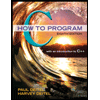
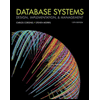
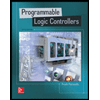