9. Show all the passes in the execution of the SelectionSort on the following set : { 20, 32, 50, 19, 10, 5, 8, 12};
9. Show all the passes in the execution of the SelectionSort on the following set :
{ 20, 32, 50, 19, 10, 5, 8, 12};

In selection sort algorithm,the lowest element is choosen and arrange it to the proper location and swap the current element with the next lowest number and so on.
Given set is : {20,32,50,19,10,5,8,12} Selection sort can be carried out as follows:
Given: 20 ,32,50,19,10,5,8,12
iteration 1: 20 32 50 19 10 5 8 12
iteration 2: 5 32 50 19 10 20 8 12
iteration 3: 5 8 50 19 10 20 32 12
iteration 4: 5 8 10 19 50 20 32 12
iteration : 5 8 10 12 50 20 32 19
iteration 6: 5 8 10 12 19 20 32 50
iteration 7:5 8 10 12 19 20 32 50
iteration 8:5 8 10 12 19 20 32 50
After Selection Sort
5 8 10 12 19 20 32 50
Program plan:
- include necessary header files
- create a class named SelectionSort
- create a public static method selectionSort()
- consider an array to store integer values
- check for the necessary conditions and search for lowest element and store it in the array
- In java there is inbuilt method selectionSort() use that for sorting.
- Each iteration is displays as for loop is used
- Finally we print the sorted array.
Program:
package com.sds.javabasic;
//create a class
public class SelectionSort{
public static void selectionSort(int[] arr){
for (int i = 0; i < arr.length - 1; i++)
{
int index = i;
for (int j = i + 1; j < arr.length; j++){
if (arr[j] < arr[index]){
index = j;//searching for lowest index
}
}
int smallerNumber = arr[index];
arr[index] = arr[i];
arr[i] = smallerNumber;
for(int k:arr){
System.out.print(k+" "); //displays each iteration
}
System.out.println();
}
}
public static void main(String a[]){
int[] arr1 = {20,32,50,19,10,5,8,12};
System.out.println("Before Selection Sort");
for(int i:arr1){
System.out.print(i+" ");
}
System.out.println();
selectionSort(arr1); //sorting array using selection sort
System.out.println("After Selection Sort");
for(int i:arr1){
System.out.print(i+" ");
}
}
}
Step by step
Solved in 3 steps

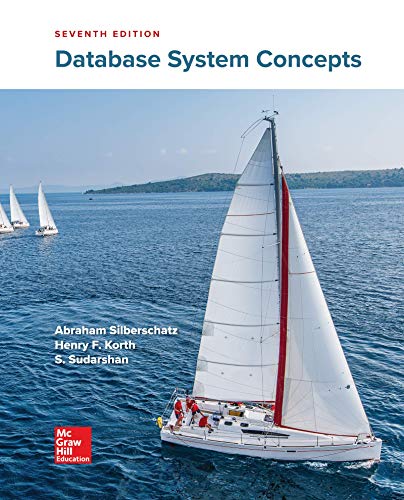
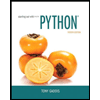
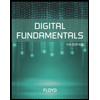
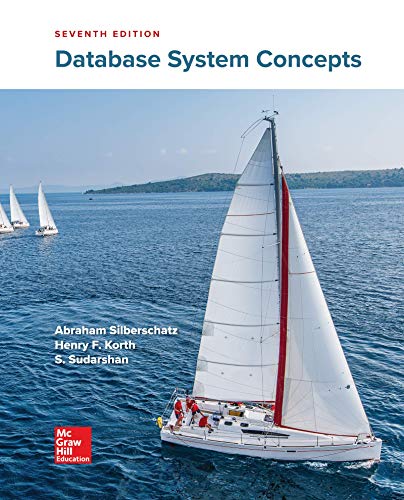
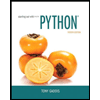
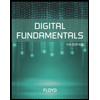
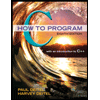
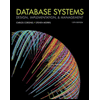
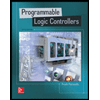