Given an ArrayList called myAL that contains the following: ["blue", "red", "yellow"] write code to insert the elements "purple" and "orange" that keeps the list in alphabetical order.
Given an ArrayList called myAL that contains the following:
["blue", "red", "yellow"]
write code to insert the elements "purple" and "orange" that keeps the list in alphabetical order.

Algorithm:
1) First we add the elements into the array list
2) then print the array list
3) then add remaining elements into the array list
4) sort the array list
5)print the sorted arraylist
Code: in java
import java.io.*;
import java.util.ArrayList;
import java.util.*;
class Arraylist1 //class declaration
{
public static void main(String[] args) //main method
throws IOException
{
int size = 5;
ArrayList<String> myAL = new ArrayList<String>(size);
myAL.add("blue");//adding the elements into the list
myAL.add("red");
myAL.add("yellow");
System.out.println(myAL);//printing the added elements
myAL.add("purple");//adding remaining elements into the arraylist
myAL.add("orange");
Collections.sort(myAL);//sorting the array list
System.out.println(myAL);//printing the array list elements after sorting
for (int i=0; i<myAL.size(); i++)
System.out.print(myAL.get(i)+" ");//printing the final output
}
}
Code Screenshot:
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

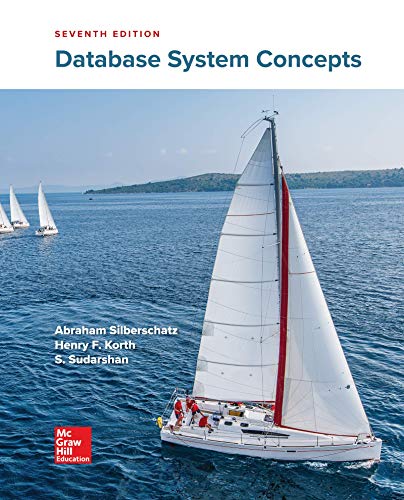
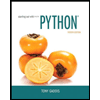
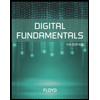
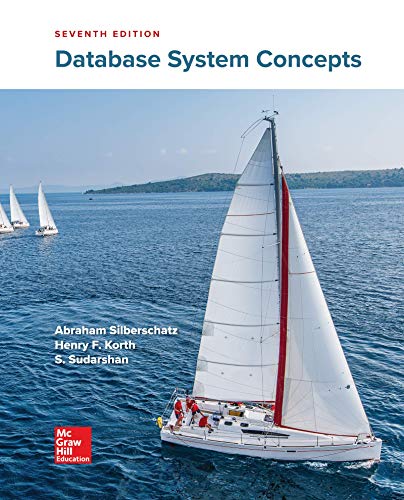
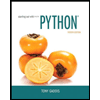
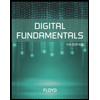
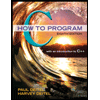
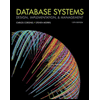
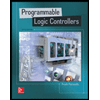